ASUR
INDIA • + Follow
Edit Project
Components
|
ESP8266 |
x 1 |
Description
Home Automation 8 Ch Relay 5 V ESP8266 - (ASUR)
Home Automation PCB, for 8 Ch Relay of 5 V
In this IoT project, I have shown how to make Alexa home automation project with manual switches using NodeMCU or ESP8266 and relay module. With this internet of things project, you can control 4 home appliances from Alexa and manual switches with and without internet. So this is a very useful homemade IoT based home automation system that you can easily make with NodeMCU, Relay Module, Push Buttons, and Alexa Echo Dot devices.
get coding idea from here
#ifdef ARDUINO_ARCH_ESP32
#include <WiFi.h>
#else
#include <ESP8266WiFi.h>
#endif
#include <Espalexa.h>
// define the GPIO connected with Relays and switches
#define RelayPin1 5 //D1
#define RelayPin2 4 //D2
#define RelayPin3 14 //D5
#define RelayPin4 12 //D6
#define SwitchPin1 10 //SD3
#define SwitchPin2 0 //D3
#define SwitchPin3 13 //D7
#define SwitchPin4 3 //RX
#define wifiLed 16 //D0
int toggleState_1 = 0; //Define integer to remember the toggle state for relay 1
int toggleState_2 = 0; //Define integer to remember the toggle state for relay 2
int toggleState_3 = 0; //Define integer to remember the toggle state for relay 3
int toggleState_4 = 0; //Define integer to remember the toggle state for relay 4
// prototypes
boolean connectWifi();
//callback functions
void firstLightChanged(uint8_t brightness);
void secondLightChanged(uint8_t brightness);
void thirdLightChanged(uint8_t brightness);
void fourthLightChanged(uint8_t brightness);
// WiFi Credentials
const char* ssid = "WiFi Name";
const char* password = "WiFi Password";
// device names
String Device_1_Name = "Study Lamp";
String Device_2_Name = "CFL";
String Device_3_Name = "Yellow light";
String Device_4_Name = "Red light";
boolean wifiConnected = false;
Espalexa espalexa;
//our callback functions
void firstLightChanged(uint8_t brightness)
{
//Control the device
if (brightness == 255)
{
digitalWrite(RelayPin1, LOW);
Serial.println("Device1 ON");
toggleState_1 = 1;
}
else
{
digitalWrite(RelayPin1, HIGH);
Serial.println("Device1 OFF");
toggleState_1 = 0;
}
}
void secondLightChanged(uint8_t brightness)
{
//Control the device
if (brightness == 255)
{
digitalWrite(RelayPin2, LOW);
Serial.println("Device2 ON");
toggleState_2 = 1;
}
else
{
digitalWrite(RelayPin2, HIGH);
Serial.println("Device2 OFF");
toggleState_2 = 0;
}
}
void thirdLightChanged(uint8_t brightness)
{
//Control the device
if (brightness == 255)
{
digitalWrite(RelayPin3, LOW);
Serial.println("Device3 ON");
toggleState_3 = 1;
}
else
{
digitalWrite(RelayPin3, HIGH);
Serial.println("Device3 OFF");
toggleState_3 = 0;
}
}
void fourthLightChanged(uint8_t brightness)
{
//Control the device
if (brightness == 255)
{
digitalWrite(RelayPin4, LOW);
Serial.println("Device4 ON");
toggleState_4 = 1;
}
else
{
digitalWrite(RelayPin4, HIGH);
Serial.println("Device4 OFF");
toggleState_4 = 0;
}
}
void relayOnOff(int relay){
switch(relay){
case 1:
if(toggleState_1 == 0){
digitalWrite(RelayPin1, LOW); // turn on relay 1
toggleState_1 = 1;
Serial.println("Device1 ON");
}
else{
digitalWrite(RelayPin1, HIGH); // turn off relay 1
toggleState_1 = 0;
Serial.println("Device1 OFF");
}
delay(100);
break;
case 2:
if(toggleState_2 == 0){
digitalWrite(RelayPin2, LOW); // turn on relay 2
toggleState_2 = 1;
Serial.println("Device2 ON");
}
else{
digitalWrite(RelayPin2, HIGH); // turn off relay 2
toggleState_2 = 0;
Serial.println("Device2 OFF");
}
delay(100);
break;
case 3:
if(toggleState_3 == 0){
digitalWrite(RelayPin3, LOW); // turn on relay 3
toggleState_3 = 1;
Serial.println("Device3 ON");
}else{
digitalWrite(RelayPin3, HIGH); // turn off relay 3
toggleState_3 = 0;
Serial.println("Device3 OFF");
}
delay(100);
break;
case 4:
if(toggleState_4 == 0){
digitalWrite(RelayPin4, LOW); // turn on relay 4
toggleState_4 = 1;
Serial.println("Device4 ON");
}
else{
digitalWrite(RelayPin4, HIGH); // turn off relay 4
toggleState_4 = 0;
Serial.println("Device4 OFF");
}
delay(100);
break;
default : break;
}
}
// connect to wifi – returns true if successful or false if not
boolean connectWifi()
{
boolean state = true;
int i = 0;
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
Serial.println("");
Serial.println("Connecting to WiFi");
// Wait for connection
Serial.print("Connecting...");
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
if (i > 20) {
state = false; break;
}
i++;
}
Serial.println("");
if (state) {
Serial.print("Connected to ");
Serial.println(ssid);
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
}
else {
Serial.println("Connection failed.");
}
return state;
}
void addDevices(){
// Define your devices here.
espalexa.addDevice(Device_1_Name, firstLightChanged); //simplest definition, default state off
espalexa.addDevice(Device_2_Name, secondLightChanged);
espalexa.addDevice(Device_3_Name, thirdLightChanged);
espalexa.addDevice(Device_4_Name, fourthLightChanged);
espalexa.begin();
}
void setup()
{
Serial.begin(115200);
pinMode(RelayPin1, OUTPUT);
pinMode(RelayPin2, OUTPUT);
pinMode(RelayPin3, OUTPUT);
pinMode(RelayPin4, OUTPUT);
pinMode(wifiLed, OUTPUT);
pinMode(SwitchPin1, INPUT_PULLUP);
pinMode(SwitchPin2, INPUT_PULLUP);
pinMode(SwitchPin3, INPUT_PULLUP);
pinMode(SwitchPin4, INPUT_PULLUP);
//During Starting all Relays should TURN OFF
digitalWrite(RelayPin1, HIGH);
digitalWrite(RelayPin2, HIGH);
digitalWrite(RelayPin3, HIGH);
digitalWrite(RelayPin4, HIGH);
// Initialise wifi connection
wifiConnected = connectWifi();
if (wifiConnected)
{
addDevices();
}
else
{
Serial.println("Cannot connect to WiFi. So in Manual Mode");
delay(1000);
}
}
void loop()
{
if (WiFi.status() != WL_CONNECTED)
{
//Serial.print("WiFi Not Connected ");
//Serial.println(wifiConnected);
digitalWrite(wifiLed, HIGH); //Turn off WiFi LED
//Manual Switch Control
if (digitalRead(SwitchPin1) == LOW){
delay(200);
relayOnOff(1);
}
else if (digitalRead(SwitchPin2) == LOW){
delay(200);
relayOnOff(2);
}
else if (digitalRead(SwitchPin3) == LOW){
delay(200);
relayOnOff(3);
}
else if (digitalRead(SwitchPin4) == LOW){
delay(200);
relayOnOff(4);
}
}
else
{
//Serial.print("WiFi Connected ");
//Serial.println(wifiConnected);
digitalWrite(wifiLed, LOW);
//Manual Switch Control
if (digitalRead(SwitchPin1) == LOW){
delay(200);
relayOnOff(1);
}
else if (digitalRead(SwitchPin2) == LOW){
delay(200);
relayOnOff(2);
}
else if (digitalRead(SwitchPin3) == LOW){
delay(200);
relayOnOff(3);
}
else if (digitalRead(SwitchPin4) == LOW){
delay(200);
relayOnOff(4);
}
//WiFi Control
if (wifiConnected){
espalexa.loop();
delay(1);
}
else {
wifiConnected = connectWifi(); // Initialise wifi connection
if(wifiConnected){
addDevices();
}
}
}
}
Dec 29,2020
2,354 views
end-flag
Home Automation 8 Ch Relay 5 V ESP8266 - (ASUR)
2 Layers PCB 95.8 x 90.6 mm FR-4, 1.6 mm, 1, HASL with lead, Black Solder Mask, White silkscreen
Home Automation PCB, for 8 Ch Relay of 5 V
2354
7
1
6.38 (2)
Published: Dec 29,2020
Purchase
Donation Received ($)
PCBWay Donate 10% cost To Author
*PCBWay community is a sharing platform. We are not responsible for any design issues and parameter issues (board thickness, surface finish, etc.) you choose.
Copy this HTML into your page to embed a link to order this shared project
Copy
Under the
Attribution-ShareAlike (CC BY-SA)
License.
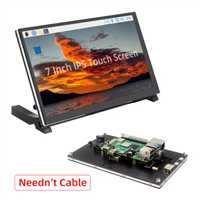
Raspberry Pi 5 7 Inch Touch Screen IPS 1024x600 HD LCD HDMI-compatible Display for RPI 4B 3B+ OPI 5 AIDA64 PC Secondary Screen(Without Speaker)
BUY NOW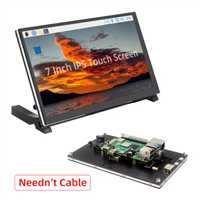
Raspberry Pi 5 7 Inch Touch Screen IPS 1024x600 HD LCD HDMI-compatible Display for RPI 4B 3B+ OPI 5 AIDA64 PC Secondary Screen(Without Speaker)
BUY NOW- Comments(1)
- Likes(7)

Upload photo
0 / 10000
It looks like you have not written anything. Please add a comment and try again.
You can upload up to 5 images!
Image size should not exceed 2MB!
File format not supported!
- David HoggardMar 05,20210 CommentsReply
-
Daniel K Feb 15,2024
-
W01449A Jan 27,2024
-
CESAR CASQUERO PERIS Mar 15,2022
-
Jeffery Thompson Jan 03,2022
-
Engineer Jan 01,2022
-
adab Jan 26,2021
-
Engineer Jan 15,2021
View More
VOTING
2 votes
- 2 USER VOTES
6.38
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
Design
1/4
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
Usability
2/4
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
Creativity
3/4
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
Content
4/4

-
1design
-
2usability
-
4creativity
-
4content
2.75
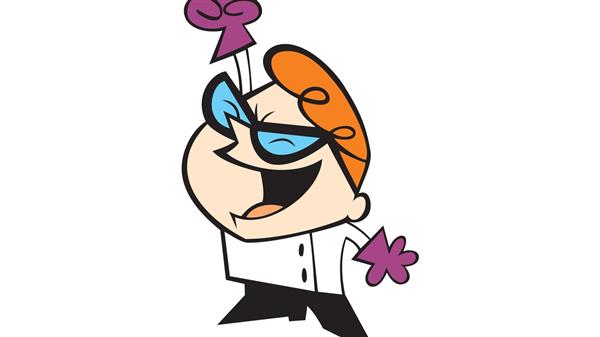
-
10design
-
10usability
-
10creativity
-
10content
10.00
More by ASUR
You may also like
-
How to measure weight with Load Cell and HX711
146 0 1 -
-
Instrumentation Input, high impedance with 16 bit 1MSPS ADC for SPI
349 0 0 -
RGB LED Matrix input module for the Framework Laptop 16
576 0 2 -
-
📦 StackBox: Modular MDF Storage Solution 📦
366 0 3