Aditya ATOM
INDIA • + Follow
Edit Project
Components
|
Mini Breadboard |
x 2 | |
|
Arduino Nano |
x 1 | |
|
MPU6050 |
x 1 | |
|
L293d driver IC |
x 1 | |
|
N20 gear motor |
x 2 | |
|
N20 motor brackets |
x 2 | |
|
N20 motor wheels |
x 1 |
Tools, APP Software Used etc.
![]() |
arduino IDEArduino
|
|
![]() |
Autodesk Fusion 360Autodesk
|
Description
The Breadboarded Self Balancing Robot
A self Balancing Robot where electronic modules and components are connected to each other on a breadboard.
How easy it is to assemble:
- 3D print the chassis
- mount the brackets on the chassis
- insert the motor
- connect all modules and components together on breadboard using jumpers
- insert the breadboard and batteries on the chassis
Making the Robot to balance:
- install the drivers for arduino
- install libraries for MPU6050 and PID controller
- Upload the code in arduino
Power the robot using 9V batteries and see it in action
More build details here.
All the project files are on Github.
Code
Arduino Code
C/C++
//Installing necessary libraries
#include <Wire.h>
#include <I2Cdev.h>
#include <MPU6050.h>
#include <PID_v1.h>
//defined macros
#define LeftMotorDir 11
#define LeftMotorPower 5
#define RightMotorDir 10
#define RightMotorPower 6
#define LeftMotorEnable 8
#define RightMotorEnable 9
//the angle where the robot is stable
double Setpoint = -0.75;
double Input, Output;
//PID controllers
double Kp = 4.6;
double Kd = 0.04;
double Ki = 1;
//required variables
int accY, accZ, gyroX;
float accAngle = 0, gyroAngle = 0, previousAngle = 0;
float gyroRate = 0;
int val = 0;
//instance of class PID
PID myPID(&Input, &Output, &Setpoint, Kp, Ki, Kd, DIRECT);
//instance of class MPU6050
MPU6050 mpu;
/*.............................SETUP.................................*/
/*...................................................................*/
void setup() {
//initializing MPU6050
mpu.initialize();
//setting the pinmodes
pinMode(LeftMotorEnable, OUTPUT);
pinMode(LeftMotorDir, OUTPUT);
pinMode(LeftMotorPower, OUTPUT);
pinMode(RightMotorEnable, OUTPUT);
pinMode(RightMotorDir, OUTPUT);
pinMode(RightMotorPower, OUTPUT);
//making enable pin high
digitalWrite(LeftMotorEnable, HIGH);
digitalWrite(RightMotorEnable, HIGH);
//setting PID parameters
myPID.SetMode(AUTOMATIC);
myPID.SetOutputLimits(-255, 255); // may change (50,255);
myPID.SetSampleTime(5); //how often pid is evaluated in millisec
myPID.SetControllerDirection(REVERSE);
//initialize the timer
initTimer2();
}
/*..............................LOOP.................................*/
/*...................................................................*/
void loop() {
accZ = mpu.getAccelerationZ();
accY = mpu.getAccelerationY();
gyroX = mpu.getRotationX();
accAngle = atan2(accZ, -accY) * RAD_TO_DEG;
gyroRate = gyroX / 131;
Input = 0.97 * (previousAngle + gyroAngle) + 0.03 * (accAngle);
previousAngle = Input;
myPID.Compute();
if (Output > Setpoint)
{
digitalWrite(LeftMotorDir, LOW);
digitalWrite(RightMotorDir, LOW);
val = map(Output, 0, 255, 18, 255);
analogWrite(LeftMotorPower, val);
analogWrite(RightMotorPower, val);
}
if (Output < Setpoint)
{
digitalWrite(LeftMotorDir, HIGH);
digitalWrite(RightMotorDir, HIGH);
val = map(Output, -255, 0, 0, 237);
analogWrite(LeftMotorPower, val);
analogWrite(RightMotorPower, val);
}
}
/*................... ........ISR_TIMER2.............................*/
/*...................................................................*/
ISR(TIMER2_COMPA_vect)
{
gyroAngle = (float)gyroRate * 0.001;
}
/*...........................iniTimer2...............................*/
/*...................................................................*/
void initTimer2()
{
//reset timer2 control register A
TCCR2A = 0;
//set CTC mode
TCCR2A |= (1 << WGM21);
TCCR2A &= ~(1 << WGM20);
TCCR2B &= ~(1 << WGM22);
//prescaler of 128
TCCR2B &= ~(1 << CS21);
TCCR2B |= ((1 << CS22) | (1 << CS20));
//reset counter
TCNT2 = 0;
//set compare register
OCR2A = 125;
//enable timer1 compare match interrupt
TIMSK2 |= (1 << OCIE2A);
//enable global interrupt
sei();
}
Schematic and Layout
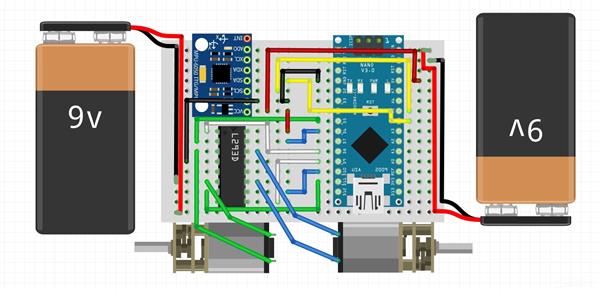
CAD-Custom parts and enclosures
Sep 24,2021
2,296 views
The Breadboarded Self Balancing Robot
A Self Balancing Robot made around a breadboard.
2296
1
0
10.00 (1)
Published: Sep 24,2021
Purchase
Donation Received ($)
PCBWay Donate 10% cost To Author
*PCBWay community is a sharing platform. We are not responsible for any design issues and parameter issues (board thickness, surface finish, etc.) you choose.
Copy this HTML into your page to embed a link to order this shared project
Copy
Under the
Attribution-NonCommercial-ShareAlike (CC BY-NC-SA)
License.
- Comments(0)
- Likes(1)
Upload photo
You can only upload 5 files in total. Each file cannot exceed 2MB. Supports JPG, JPEG, GIF, PNG, BMP
0 / 10000
It looks like you have not written anything. Please add a comment and try again.
You can upload up to 5 images!
Image size should not exceed 2MB!
File format not supported!
View More
-
Engineer Sep 30,2021
View More
VOTING
1 votes
- 1 USER VOTES
10.00
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
Design
1/4
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
Usability
2/4
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
Creativity
3/4
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
Content
4/4
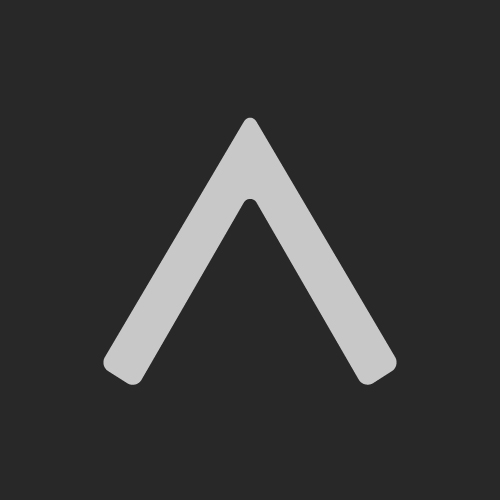
-
10design
-
10usability
-
10creativity
-
10content
10.00
More by Aditya ATOM
You may also like
-
Build a Walking Robot: Theo Jansen Style 3D Printed Octopod
145 0 4 -
-
-
kmMiniSchield MIDI I/O - IN/OUT/THROUGH MIDI extension for kmMidiMini
141 0 0 -
DIY Laser Power Meter with Arduino
218 0 2 -
-
-
Box & Bolt, 3D Printed Cardboard Crafting Tools
187 0 2