![]() |
OrCad Cadance |
Voronoi PCB Lamp V2
Hey Everyone what's up.
So here's one of the previous projects I made a few weeks ago.
Voronoi Diagram-Based PCB Lamp is an RGB Lamp made entirely from PCBs, I used four rectangular PCBs with two square PCBs to make the body of the LAMP.
RGB Color in this Lamp can be changed by pressing the button on top of it, this button is connected with the lower square board that contains the RGB LEDs and the Attiny85 Microcontroller.
SUPPLIES
- Attiny85 SOIC8
- Custom PCBs- Main Square MCU PCB and Rectangular PCB
- WS2812B LEDs (2020 Package)
- USB Type C Port
- Diode M7
- 2R0 Resistor 1206 Package
- Arduino as ISP Setup for flashing the MCU
- SMD button
- UC202 Connector and wire harness
- Solder wire
PROBLEM IN PREVIOUS EDITION
Version 1 of this project had a few issues, including the wrong footprint issue for the RGB LED and the Gap Issue between two rectangular boards.
For solving the Gap Issue, I made the Square PCBs (Microcontroller board and switchboard) a little bit smaller than their previous version.
I decreased the square length by 1mm from all the sides and this was the result.
As for the RGB LED issue, I added SMD WS2812B 2020 Package LED on the top side of the PCB and that solved the RGB LED issue.
PCB Design
This was the schematic I used in this built, it contains an Attiny85 connected with four RGB LEDs that are all powered by a USB Type C Port which has a Diode and a Load resistor in series to limit the output current.
After finalizing the schematic, I converted it into a board file and prepared two PCBs that were 1mm shorter from all sides.
This Length reduction thing was crucial as it controls the gap that occurs during the final soldering process of Side PCBs.
PCBWAY REVIEW
After finalizing the PCBs, I send both PCBs to PCBWAY for samples.
I choose a white soldermask with Black Silkscreen and placed the order.
After waiting for a week, PCBs arrived and their quality was super good.
Overall, there was no error whatsoever and these PCBs that I made were complex.
Loved how each detail I made in this PCB was proper and perfect.
Check out PCBWAY for getting great PCB service for less cost!
PCB ASSEMBLY PROCESS
PCB Assembly Process consists of five major steps that include
- Removing Conjoined PCBs
- Solder Paste Dispensing Process
- Pick & place process
- Hotplate reflow
- Switch and connector PCB assembly
Removing Conjoined PCBs
While Designing the Square PCBs, I made two separate boards, one for microcontroller setup and one for the switch that also acts as a Lid of the Lamp.
If I made two PCBs and placed an order for them separately, that would've cost a lot so in order to save a few bucks, I connected two same-size square boards together with two RIBs that can be easily cut down to separate two PCBs.
This saves a lot of costs and it's a pretty neat method of combining two or more orders together, it's a kind of PCB Paneling.
Solder Paste Dispensing Process
We apply solder paste with a solder paste dispenser syringe or the proper method is to use stencil here which I don't have. but anyway, after this, we can now move on to the next process, which is to add components to each pad.
Pick & place process
Next, we carefully pick all the components with an ESD tweezer and place them in their assigned place one by one.
Hotplate reflow
Then after doing the pick and place process, we carefully lifted the whole PCB and place it on the hotplate.
Basically, in this hotplate reflow process, the surface heats up to the solder paste melting temp and it slowly melts. after a few mins when the solder paste completely melts, we remove the PCB and let it cool down for a moment.
Switch and connector PCB assembly
I then added SMD Button and UC202 connector manually with help of a soldering iron.
CODE
Here's the code I used in this project, it's based around Adafruit's neopixel library so you need to download that before using this sketch.
As for Uploading this sketch into the Attiny, do check out this post of mine.
#include <Adafruit_NeoPixel.h>
#define BUTTON_PIN 4 //D1- 5// driven with a pull-up resistor so the switch should// pull the pin to ground momentarily. On a high -> low// transition the button press logic will execute.
#define PIXEL_PIN 0 // Digital IO pin connected to the NeoPixels.//D4 - 2
#define PIXEL_COUNT 4
// Parameter 1 = number of pixels in strip, neopixel stick has 8
// Parameter 2 = pin number (most are valid)
// Parameter 3 = pixel type flags, add together as needed:
// NEO_RGB Pixels are wired for RGB bitstream
// NEO_GRB Pixels are wired for GRB bitstream, correct for neopixel stick
// NEO_KHZ400 400 KHz bitstream (e.g. FLORA pixels)
// NEO_KHZ800 800 KHz bitstream (e.g. High Density LED strip), correct for neopixel stick
Adafruit_NeoPixel strip = Adafruit_NeoPixel(PIXEL_COUNT, PIXEL_PIN, NEO_GRB + NEO_KHZ800);
bool oldState = HIGH;
int showType = 0;
void setup() {
pinMode(BUTTON_PIN, INPUT_PULLUP);
strip.begin();
strip.show(); // Initialize all pixels to 'off'
}
void loop() {
// Get current button state.bool newState = digitalRead(BUTTON_PIN);
// Check if state changed from high to low (button press).if (newState == LOW && oldState == HIGH) {
// Short delay to debounce button.
delay(20);
// Check if button is still low after debounce.
newState = digitalRead(BUTTON_PIN);
if (newState == LOW) {
showType++;
if (showType > 9)
showType=0;
startShow(showType);
}
}
// Set the last button state to the old state.
oldState = newState;
}
void startShow(int i) {
switch(i){
case 0: colorWipe(strip.Color(0, 0, 0), 50); // Black/offbreak;
case 1: colorWipe(strip.Color(255, 0, 0), 50); // Redbreak;
case 2: colorWipe(strip.Color(0, 255, 0), 50); // Greenbreak;
case 3: colorWipe(strip.Color(0, 0, 255), 50); // Bluebreak;
case 4: theaterChase(strip.Color(127, 127, 127), 50); // Whitebreak;
case 5: theaterChase(strip.Color(127, 0, 0), 50); // Redbreak;
case 6: theaterChase(strip.Color( 0, 0, 127), 50); // Bluebreak;
case 7: rainbow(20);
break;
case 8: rainbowCycle(20);
break;
case 9: theaterChaseRainbow(50);
break;
}
}
// Fill the dots one after the other with a color
void colorWipe(uint32_t c, uint8_t wait) {
for(uint16_t i=0; i<strip.numPixels(); i++) {
strip.setPixelColor(i, c);
strip.show();
delay(wait);
}
}
void rainbow(uint8_t wait) {
uint16_t i, j;
for(j=0; j<256; j++) {
for(i=0; i<strip.numPixels(); i++) {
strip.setPixelColor(i, Wheel((i+j) & 255));
}
strip.show();
delay(wait);
}
}
// Slightly different, this makes the rainbow equally distributed throughout
void rainbowCycle(uint8_t wait) {
uint16_t i, j;
for(j=0; j<256*5; j++) { // 5 cycles of all colors on wheelfor(i=0; i< strip.numPixels(); i++) {
strip.setPixelColor(i, Wheel(((i * 256 / strip.numPixels()) + j) & 255));
}
strip.show();
delay(wait);
}
}
//Theatre-style crawling lights.
void theaterChase(uint32_t c, uint8_t wait) {
for (int j=0; j<10; j++) { //do 10 cycles of chasingfor (int q=0; q < 3; q++) {
for (int i=0; i < strip.numPixels(); i=i+3) {
strip.setPixelColor(i+q, c); //turn every third pixel on
}
strip.show();
delay(wait);
for (int i=0; i < strip.numPixels(); i=i+3) {
strip.setPixelColor(i+q, 0); //turn every third pixel off
}
}
}
}
//Theatre-style crawling lights with rainbow effect
void theaterChaseRainbow(uint8_t wait) {
for (int j=0; j < 256; j++) { // cycle all 256 colors in the wheelfor (int q=0; q < 3; q++) {
for (int i=0; i < strip.numPixels(); i=i+3) {
strip.setPixelColor(i+q, Wheel( (i+j) % 255)); //turn every third pixel on
}
strip.show();
delay(wait);
for (int i=0; i < strip.numPixels(); i=i+3) {
strip.setPixelColor(i+q, 0); //turn every third pixel off
}
}
}
}
// Input a value 0 to 255 to get a color value.
// The colours are a transition r - g - b - back to r.
uint32_t Wheel(byte WheelPos) {
WheelPos = 255 - WheelPos;
if(WheelPos < 85) {
return strip.Color(255 - WheelPos * 3, 0, WheelPos * 3);
}
if(WheelPos < 170) {
WheelPos -= 85;
return strip.Color(0, WheelPos * 3, 255 - WheelPos * 3);
}
WheelPos -= 170;
return strip.Color(WheelPos * 3, 255 - WheelPos * 3, 0);
}
Rectangular Board Main assembly
After testing the Main MCU working, we can now move on to the final process which is to put everything together and start the assembly process.
- I first added solder wire on one side of the soldering pad
- after placing the Main PCB in its right position, I joined its soldering pad with the soldering pad of the wall PCB. I then added solder wire on another side as well.
- I then placed the switch PCB with the same method in its place and added other wall PCBs on both sides by soldering their pads together.
Final Result
Here's the final result with no Gap issue and no LED issue this time.
Conclusion and V3
This LAMP does work but there's still a flaw in this project.
I accidentally made the wrong connections to the LED which I edited out by adding a few jumper wires and disconnecting existing connections for the LED.
Here's how I corrected things, followed the right connections, and made changes to PCB Design.
This is it for today, Leave a comment if you guys need any help, and I'll be back with another project soon!
Thanks, PCBWAY for supporting this project, Do check PCBWAY for getting great PCB service for less cost!
Peace
#include <Adafruit_NeoPixel.h>
#define BUTTON_PIN 4 //D1- 5
// driven with a pull-up resistor so the switch should
// pull the pin to ground momentarily. On a high -> low
// transition the button press logic will execute.
#define PIXEL_PIN 0 // Digital IO pin connected to the NeoPixels.//D4 - 2
#define PIXEL_COUNT 4
// Parameter 1 = number of pixels in strip, neopixel stick has 8
// Parameter 2 = pin number (most are valid)
// Parameter 3 = pixel type flags, add together as needed:
// NEO_RGB Pixels are wired for RGB bitstream
// NEO_GRB Pixels are wired for GRB bitstream, correct for neopixel stick
// NEO_KHZ400 400 KHz bitstream (e.g. FLORA pixels)
// NEO_KHZ800 800 KHz bitstream (e.g. High Density LED strip), correct for neopixel stick
Adafruit_NeoPixel strip = Adafruit_NeoPixel(PIXEL_COUNT, PIXEL_PIN, NEO_GRB + NEO_KHZ800);
bool oldState = HIGH;
int showType = 0;
void setup() {
pinMode(BUTTON_PIN, INPUT_PULLUP);
strip.begin();
strip.show(); // Initialize all pixels to 'off'
}
void loop() {
// Get current button state.
bool newState = digitalRead(BUTTON_PIN);
// Check if state changed from high to low (button press).
if (newState == LOW && oldState == HIGH) {
// Short delay to debounce button.
delay(20);
// Check if button is still low after debounce.
newState = digitalRead(BUTTON_PIN);
if (newState == LOW) {
showType++;
if (showType > 9)
showType=0;
startShow(showType);
}
}
// Set the last button state to the old state.
oldState = newState;
}
void startShow(int i) {
switch(i){
case 0: colorWipe(strip.Color(0, 0, 0), 50); // Black/off
break;
case 1: colorWipe(strip.Color(255, 0, 0), 50); // Red
break;
case 2: colorWipe(strip.Color(0, 255, 0), 50); // Green
break;
case 3: colorWipe(strip.Color(0, 0, 255), 50); // Blue
break;
case 4: theaterChase(strip.Color(127, 127, 127), 50); // White
break;
case 5: theaterChase(strip.Color(127, 0, 0), 50); // Red
break;
case 6: theaterChase(strip.Color( 0, 0, 127), 50); // Blue
break;
case 7: rainbow(20);
break;
case 8: rainbowCycle(20);
break;
case 9: theaterChaseRainbow(50);
break;
}
}
// Fill the dots one after the other with a color
void colorWipe(uint32_t c, uint8_t wait) {
for(uint16_t i=0; i<strip.numPixels(); i++) {
strip.setPixelColor(i, c);
strip.show();
delay(wait);
}
}
void rainbow(uint8_t wait) {
uint16_t i, j;
for(j=0; j<256; j++) {
for(i=0; i<strip.numPixels(); i++) {
strip.setPixelColor(i, Wheel((i+j) & 255));
}
strip.show();
delay(wait);
}
}
// Slightly different, this makes the rainbow equally distributed throughout
void rainbowCycle(uint8_t wait) {
uint16_t i, j;
for(j=0; j<256*5; j++) { // 5 cycles of all colors on wheel
for(i=0; i< strip.numPixels(); i++) {
strip.setPixelColor(i, Wheel(((i * 256 / strip.numPixels()) + j) & 255));
}
strip.show();
delay(wait);
}
}
//Theatre-style crawling lights.
void theaterChase(uint32_t c, uint8_t wait) {
for (int j=0; j<10; j++) { //do 10 cycles of chasing
for (int q=0; q < 3; q++) {
for (int i=0; i < strip.numPixels(); i=i+3) {
strip.setPixelColor(i+q, c); //turn every third pixel on
}
strip.show();
delay(wait);
for (int i=0; i < strip.numPixels(); i=i+3) {
strip.setPixelColor(i+q, 0); //turn every third pixel off
}
}
}
}
//Theatre-style crawling lights with rainbow effect
void theaterChaseRainbow(uint8_t wait) {
for (int j=0; j < 256; j++) { // cycle all 256 colors in the wheel
for (int q=0; q < 3; q++) {
for (int i=0; i < strip.numPixels(); i=i+3) {
strip.setPixelColor(i+q, Wheel( (i+j) % 255)); //turn every third pixel on
}
strip.show();
delay(wait);
for (int i=0; i < strip.numPixels(); i=i+3) {
strip.setPixelColor(i+q, 0); //turn every third pixel off
}
}
}
}
// Input a value 0 to 255 to get a color value.
// The colours are a transition r - g - b - back to r.
uint32_t Wheel(byte WheelPos) {
WheelPos = 255 - WheelPos;
if(WheelPos < 85) {
return strip.Color(255 - WheelPos * 3, 0, WheelPos * 3);
}
if(WheelPos < 170) {
WheelPos -= 85;
return strip.Color(0, WheelPos * 3, 255 - WheelPos * 3);
}
WheelPos -= 170;
return strip.Color(WheelPos * 3, 255 - WheelPos * 3, 0);
}
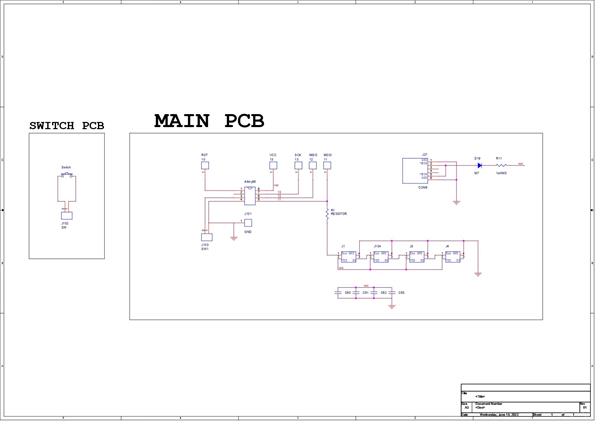
Voronoi PCB Lamp V2
*PCBWay community is a sharing platform. We are not responsible for any design issues and parameter issues (board thickness, surface finish, etc.) you choose.
- Comments(0)
- Likes(1)
-
(DIY) C64iSTANBUL Jun 17,2022
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by Arnov Arnov sharma
-
Delete Button XL Greetings everyone and welcome back, and here's something fun and useful.In essence, the Delete Butt...
-
Arduino Retro Game Controller Greetings everyone and welcome back. Here's something fun.The Arduino Retro Game Controller was buil...
-
Super Power Buck Converter Greetings everyone and welcome back!Here's something powerful, The SUPER POWER BUCK CONVERTER BOARD ...
-
Pocket Temp Meter Greetings and welcome back.So here's something portable and useful: the Pocket TEMP Meter project.As...
-
Pico Powered DC Fan Driver Hello everyone and welcome back.So here's something cool: a 5V to 12V DC motor driver based around a...
-
Mini Solar Light Project with a Twist Greetings.This is the Cube Light, a Small and compact cube-shaped emergency solar light that boasts ...
-
PALPi V5 Handheld Retro Game Console Hey, Guys what's up?So this is PALPi which is a Raspberry Pi Zero W Based Handheld Retro Game Consol...
-
DIY Thermometer with TTGO T Display and DS18B20 Greetings.So this is the DIY Thermometer made entirely from scratch using a TTGO T display board and...
-
Motion Trigger Circuit with and without Microcontroller GreetingsHere's a tutorial on how to use an HC-SR505 PIR Module with and without a microcontroller t...
-
Motor Driver Board Atmega328PU and HC01 Hey, what's up folks here's something super cool and useful if you're making a basic Robot Setup, A ...
-
Power Block Hey Everyone what's up!So this is Power block, a DIY UPS that can be used to power a bunch of 5V Ope...
-
Goku PCB Badge V2 Hey everyone what's up!So here's something SUPER cool, A PCB Board themed after Goku from Dragon Bal...
-
RGB Mixinator V2 Hey Everyone how you doin!So here's a fun little project that utilizes an Arduino Nano, THE MIXINATO...
-
Gengar PCB Art Hey guys and how you doing!So this is the GENGAR PCB Badge or a Blinky Board which is based around 5...
-
R2D2 Mini Edition So here's something special, A Mini R2D2 PCB that speaks ASTROMECH.Astromech is a fictional language...
-
C-3PO Blinky Board Hey guys and how you doing!So this is the C3P0 PCB Badge or a Blinky Board which is based around 555...
-
WALKPi Breadboard Version Greetings everyone and welcome back, Here's something loud and musical.Similar to a traditional walk...
-
BOXcilloscope Greetings to everyone, and welcome back.This is BOXcilloscope, a homemade portable oscilloscope that...
-
-
-
3D printed Enclosure Backplate for Riden RD60xx power supplies
56 0 0 -
-
-
-
Sega Master System RGB Encoder Switcher Z80 QSB v1.2
57 0 0 -
18650 2S2P Battery Charger, Protection and 5V Output Board
78 0 0 -
High Precision Thermal Imager + Infrared Thermometer | OpenTemp
422 0 6 -
Sony PlayStation Multi Output Frequency Oscillator (MOFO) v1
129 0 2