|
Arduino_UNO_R3 |
x 1 | |
|
L293D Motor Driver Shield |
x 1 | |
|
Generic Jumper WiresVarious
|
x 1 | |
|
Robot Chassis, Wheels, Geared Motors |
x 1 | |
|
Line Sensor Modules |
x 1 | |
|
Black Tape |
x 1 |
![]() |
arduino IDEArduino
|
Line Follower Robot using Arduino
A Line Follower Robot (LFR) is an autonomous robot that follows a predefined path, typically a black line on a white surface or vice versa. This guide will walk you through building and programming an Arduino-powered Line Follower Robot from scratch.
How a Line Follower Robot Works
The robot detects and follows a line using IR sensors. Black surfaces absorb most light, while white surfaces reflect it. The IR sensors, consisting of an IR emitter (LED) and an IR receiver (photodiode), use this principle:
White surface: The emitted IR light reflects back to the sensor.
Black line: The light is absorbed, and the sensor detects no reflection.
How does a Line Follower Robot Navigates?
The robot's movement is controlled by the signals received from two sensors:
Moving Forward: Both sensors detect the white surface (black line in between), so both motors move forward.
Turning Left: The left sensor detects the black line, stopping or reversing the left motor while the right motor continues forward.
Turning Right: The right sensor detects the black line, causing the left motor to move forward while the right motor stops or reverses.
Stopping: Both sensors detect the black line, prompting the robot to halt.
Why Use an L293D Motor Driver Shield?
DC motors require higher voltage and current than what Arduino can provide. The L293D motor driver shield handles power management and provides bi-directional control, enabling both forward and reverse motion.
To learn more, refer to our L293D Motor Driver Shield Tutorial.
Wiring Diagram & Connections
The circuit includes:
Two IR sensors
One L293D motor driver
Four 12V BO motors
Arduino UNO
Connections:
IR Sensor VCC & GND → Arduino VCC & GND
Left Sensor Output → Arduino Analog A0
Right Sensor Output → Arduino Analog A1
Left Motors → Motor Driver Shield Port M3
Right Motors → Motor Driver Shield Port M4
Power Supply → 12V battery (connected to Arduino and shield)
Safety Tip: When uploading code, disconnect the power jumper on the motor driver shield and remove the 12V power supply before connecting Arduino via USB.
Step-by-Step Assembly
Step 1: Prepare the Chassis
Use a readymade chassis or build one manually.
Solder all four motors with 15cm wires.
Step 2: Mount the Components
Secure motors, sensors, and wheels to the chassis.
Position the sensors correctly:
Distance between sensors: 11 to 11.5 cm
Sensor height from surface: 2 cm
Step 3: Connect the Parts
Attach the motor driver shield onto the Arduino board.
Connect sensors and motors as per the wiring diagram.
Step 4: Upload the Code
Disconnect power jumper before programming.
Upload the Arduino Line Follower Robot Code.
Step 5: Power On the Robot
Attach the battery pack at the rear.
Secure it with a wire tie or double-sided tape.
Reconnect the power jumper.
Arduino Line Follower Robot Code
/*
Library used: Adafruit Motor Shield library V1 version: 1.0.1
For this code to run as expected:
1.The centre to centre distance between the Line sensors should be 11 to 11.5 cm
2. The width of black tape should be 4.8 to 5 cm
3. The distance of the sensor LED from the flat ground surface should be 2 cm.
*/
#include <AFMotor.h>
// MACROS for Debug print, while calibrating set its value to 1 else keep it 0
#define DEBUG_PRINT 0
// MACROS for Analog Input
#define LEFT_IR A0
#define RIGHT_IR A1
// MACROS to control the Robot
#define DETECT_LIMIT 300
#define FORWARD_SPEED 60
#define TURN_SHARP_SPEED 150
#define TURN_SLIGHT_SPEED 120
#define DELAY_AFTER_TURN 140
#define BEFORE_TURN_DELAY 10
// BO Motor control related data here
// Here motors are running using M3 and M4 of the shield and Left Motor is connected to M3 and Right Motor is connected to M4 using IC2 of the shield
AF_DCMotor motorL(3); // Uses PWM0B pin of Arduino Pin 5 for Enable
AF_DCMotor motorR(4); // Uses PWM0A pin of Arduino Pin 6 for Enable
// variables to store the analog values
int left_value;
int right_value;
// Set the last direction to Stop
char lastDirection = 'S';
void setup() {
#if DEBUG_PRINT
Serial.begin(9600);
#endif
// Set the current speed of Left Motor to 0
motorL.setSpeed(0);
// turn on motor
motorL.run(RELEASE);
// Set the current speed of Right Motor to 0
motorR.setSpeed(0);
// turn off motor
motorR.run(RELEASE);
// To provide starting push to Robot these values are set
motorR.run(FORWARD);
motorL.run(FORWARD);
motorL.setSpeed(255);
motorR.setSpeed(255);
delay(40); // delay of 40 ms
}
void loop() {
left_value = analogRead(LEFT_IR);
right_value = analogRead(RIGHT_IR);
#if DEBUG_PRINT
// This is for debugging. To check the analog inputs the DETECT_LIMIT MACRO value 300 is set by analysing the debug prints
Serial.print(left_value);
Serial.print(",");
Serial.print(right_value);
Serial.print(",");
Serial.print(lastDirection);
Serial.write(10);
#endif
// Right Sensor detects black line and left does not detect
if (right_value >= DETECT_LIMIT && !(left_value >= DETECT_LIMIT)) {
turnRight();
}
// Left Sensor detects black line and right does not detect
else if ((left_value >= DETECT_LIMIT) && !(right_value >= DETECT_LIMIT)) {
turnLeft();
}
// both sensors doesn't detect black line
else if (!(left_value >= DETECT_LIMIT) && !(right_value >= DETECT_LIMIT)) {
moveForward();
}
// both sensors detect black line
else if ((left_value >= DETECT_LIMIT) && (right_value >= DETECT_LIMIT)) {
stop();
}
}
void moveForward() {
if (lastDirection != 'F') {
// To provide starting push to Robot when last direction was not forward
motorR.run(FORWARD);
motorL.run(FORWARD);
motorL.setSpeed(255);
motorR.setSpeed(255);
lastDirection = 'F';
delay(20);
} else {
// If the last direction was forward
motorR.run(FORWARD);
motorL.run(FORWARD);
motorL.setSpeed(FORWARD_SPEED);
motorR.setSpeed(FORWARD_SPEED);
}
}
void stop() {
if (lastDirection != 'S') {
// When stop is detected move further one time to check if its actual stop or not, needed when the robot turns
motorR.run(FORWARD);
motorL.run(FORWARD);
motorL.setSpeed(255);
motorR.setSpeed(255);
lastDirection = 'S';
delay(40);
} else {
// When stop is detected next time then stop the Robot
motorL.setSpeed(0);
motorR.setSpeed(0);
motorL.run(RELEASE);
motorR.run(RELEASE);
lastDirection = 'S';
}
}
void turnRight(void) {
// If first time Right Turn is taken
if (lastDirection != 'R') {
lastDirection = 'R';
// Stop the motor for some time
motorL.setSpeed(0);
motorR.setSpeed(0);
delay(BEFORE_TURN_DELAY);
// take Slight Right turn
motorL.run(FORWARD);
motorR.run(BACKWARD);
motorL.setSpeed(TURN_SLIGHT_SPEED);
motorR.setSpeed(TURN_SLIGHT_SPEED);
} else {
// take sharp Right turn
motorL.run(FORWARD);
motorR.run(BACKWARD);
motorL.setSpeed(TURN_SHARP_SPEED);
motorR.setSpeed(TURN_SHARP_SPEED);
}
delay(DELAY_AFTER_TURN);
}
void turnLeft() {
// If first time Left Turn is taken
if (lastDirection != 'L') {
lastDirection = 'L';
// Stop the motor for some time
motorL.setSpeed(0);
motorR.setSpeed(0);
delay(BEFORE_TURN_DELAY);
// take slight Left turn
motorR.run(FORWARD);
motorL.run(BACKWARD);
motorL.setSpeed(TURN_SLIGHT_SPEED);
motorR.setSpeed(TURN_SLIGHT_SPEED);
} else {
// take sharp Left turn
motorR.run(FORWARD);
motorL.run(BACKWARD);
motorL.setSpeed(TURN_SHARP_SPEED);
motorR.setSpeed(TURN_SHARP_SPEED);
}
delay(DELAY_AFTER_TURN);
}
Video
To learn more checkout this tutorial: Line Follower Robot using Arduino
Line Follower Robot using Arduino
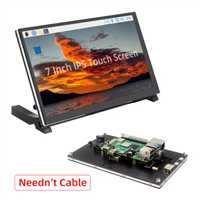
Raspberry Pi 5 7 Inch Touch Screen IPS 1024x600 HD LCD HDMI-compatible Display for RPI 4B 3B+ OPI 5 AIDA64 PC Secondary Screen(Without Speaker)
BUY NOW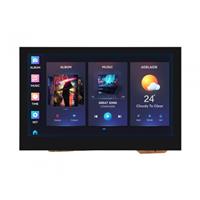
ESP32-S3 4.3inch Capacitive Touch Display Development Board, 800×480, 5-point Touch, 32-bit LX7 Dual-core Processor
BUY NOW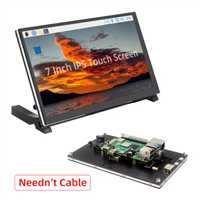
Raspberry Pi 5 7 Inch Touch Screen IPS 1024x600 HD LCD HDMI-compatible Display for RPI 4B 3B+ OPI 5 AIDA64 PC Secondary Screen(Without Speaker)
BUY NOW- Comments(1)
- Likes(1)

- PCBWay TeamFeb 06,20250 CommentsReply
-
Engineer Feb 10,2025
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by Rachana Jain
-
Line Follower Robot using Arduino A Line Follower Robot (LFR) is an autonomous robot that follows a predefined path, typically a black...
-
Ultrasonic Sensor HC-SR04 Interfacing with Arduino The HC-SR04 Ultrasonic Sensor is a widely used and easy-to-implement sensor for distance measurement...
-
Interfacing IR Sensor Module with Arduino Nano In this project, we will learn how to interface an Infrared (IR) sensor module with an Arduino Nano....
-
Modifying a Hotplate to a Reflow Solder Station
187 0 2 -
MPL3115A2 Barometric Pressure, Altitude, and Temperature Sensor
139 0 1 -
-
Nintendo 64DD Replacement Shell
184 0 1 -
V2 Commodore AMIGA USB-C Power Sink Delivery High Efficiency Supply Triple Output 5V ±12V OLED display ATARI compatible shark 100W
393 4 1 -
How to measure weight with Load Cell and HX711
432 0 3 -
-
Instrumentation Input, high impedance with 16 bit 1MSPS ADC for SPI
532 0 0