|
EasyEDAEasyEDA
|
Bit-Duino All-in-one robotics shield
This Arduino Uno Development shield is made for beginners who can try to program themselves using reference codes'. They can easily use the reference code and create their project! Using this, you can control two motors and two servo motors. Your imagination is your only limit! Using this you can learn how to use a few sensors and you can create your own project from scratch!
How to power up the shield.
You can use any type of battery, it's your choice. Make sure they are in a battery casing and have two wires as negative and positive. The screw terminal is used here as the connector.
Operating voltage - 7 to 9V
Connecting sensors and modules.
Let's get to know each component and what are they for,
L293D IC - Used to control the two motors.
L.E.D. - As a power indicator.
1k Resistor - Reduce the voltage and current to L.E.D.
Male pin headers - To connect the shield to Arduino, join servo motors, and connect sensors.
Female pin headers - To connect sensors, to connect extra pins.
SPDT switch - To on/off the shield.
Push-button - To reset the code.
Screw-terminal - To connect power wires, to connect output motor wires.
Reference codes'
Code is supplied with #define and the servo library.
#include <Servo.h>
#define motor1 4
#define motor2 5
#define motor3 6
#define motor4 7
Servo.servo1;
Servo.servo2;
#define tx 0
#define rx 1
#define trig 2
#define echo 8
#define pir_out 3
#define temp 11
#define ir1 A0
#define ir2 A1
#define sda A4
#define scl A5
void setup() {
// put your setup code here, to run once:
servo1.attach(9);
servo2.attach(10);
}
void loop() {
// put your main code here, to run repeatedly:
}
If you want to control servo motors put this in format and in every single program.
#include <Servo.h>
Servo.servo1;
Servo.servo2;
void setup() {
// put your setup code here, to run once:
servo1.attach(9);
servo2.attach(10);
}
void loop() {
// put your main code here, to run repeatedly:
}
Code for motor control.
#define motor1 4
#define motor2 5
#define motor3 6
#define motor4 7
#define tx 0
#define rx 1
#define trig 2
#define echo 8
#define pir_out 3
#define temp 11
#define ir1 A0
#define ir2 A1
#define sda A4
#define scl A5
void setup() {
// put your setup code here, to run once:
pinMode(motor1, OUTPUT)
pinMode(motor2, OUTPUT)
pinMode(motor3, OUTPUT)
pinMode(motor4, OUTPUT)// telling arduino that the motor pins are output/they are the controlling pins.
}
void loop() {
// put your main code here, to run repeatedly:
//1st motor forward/backward code
digitalWrite(motor1, HIGH)
digitalWrite(motor2, LOW)// runs the 1st motor in a direction (connct the motor to the screw terminal and see where it is rotating, if rotating forward it is forward, if rotating backward it is backward)
digitalWrite(motor1, LOW)
digitalWrite(motor2, HIGH)// runs the 1st motor in the opposite direction according to the above piece of code.
//
//2nd motor forward/backward code
digitalWrite(motor3, HIGH)
digitalWrite(motor4, LOW)// runs the 2nd motor in a direction (connct the motor to the screw terminal and see where it is rotating, if rotating forward it is forward, if rotating backward it is backward)
digitalWrite(motor3, LOW)
digitalWrite(motor4, HIGH)// runs the 2nd motor in the opposite direction according to the above piece of code.
//
//1st motor stop code
digitalWrite(motor1, LOW)
digitalWrite(motor2, LOW)//stops the 1st motor.
//
//2nd motor stop code
digitalWrite(motor3, LOW)
digitalWrite(motor4, LOW)//stops the 2nd motor.
//
//to turn you should make one wheel stop and one running, and then record where its turning. then you can make a function called "turn *the side turned*" and use it on your project. make functions to forward(all two wheels running forward), backward (all two wheels running backward), stop( all two wheels stop) and use them on your project.
}
Ultrasonic Distance Sensor code
#define motor1 4
#define motor2 5
#define motor3 6
#define motor4 7
#define tx 0
#define rx 1
#define trig 2
#define echo 8
#define pir_out 3
#define temp 11
#define ir1 A0
#define ir2 A1
#define sda A4
#define scl A5
void setup() {
// put your setup code here, to run once:
pinMode(trig,OUTPUT);//setting pin trig to output
pinMode(echo,INPUT);//setting pin echo to input
}
void loop() {
// put your main code here, to run repeatedly:
digitalWrite(trig,LOW);
delayMicroseconds(2);
digitalWrite(trig,HIGH);
delayMicroseconds(2);
digitalWrite(trig,LOW);
long t = pulseIn(echo, HIGH);// starting the sensor
long inches = t / 74 / 2;
long cm = t / 29 / 2; // getiing the real in/cm value
if(cm<10){
//whatever you program here will run if the sensor detects something less than 10cm away from it*sensor*. can replace the above "<" with any symbol and make it "less than", "equal", "equal or less than" or "equal or greater than".
}
}
PIR sensor code
#define motor1 4
#define motor2 5
#define motor3 6
#define motor4 7
#define tx 0
#define rx 1
#define trig 2
#define echo 8
#define pir_out 3
#define temp 11
#define ir1 A0
#define ir2 A1
#define sda A4
#define scl A5
void setup() {
// put your setup code here, to run once:
pinMode(pir_out, INPUT);
}
void loop() {
// put your main code here, to run repeatedly:
int val = digitalRead(pir_out);
if (val == 1){
//put a function here and it will run when sensor detects motion.
}
if (val == 0){
//put a function here and it will run when sensor doesn't detect any motion.
}
}
HC-05/HC-06 Bluetooth module code(storing and receiving numbers)
char ble;
#define motor1 4
#define motor2 5
#define motor3 6
#define motor4 7
#define tx 0
#define rx 1
#define trig 2
#define echo 8
#define pir_out 3
#define temp 11
#define ir1 A0
#define ir2 A1
#define sda A4
#define scl A5
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
}
void loop() {
// put your main code here, to run repeatedly:
if(Serial.available()){
ble = Serial.read();
}
if (ble == ' //any number you are going to send through bluetooth '){
//anything you code here gonna run when bluetooth module detects the given number. only numbers supported and requires smartphone to send signals. you can use any android Arduino Bluetooth control app and test the signal using the code below. remember when uploading the code remove the bluetooth module and reconnect it when the uploading is finished. or else the code is not gonna upload or it can severely damage your bluetooth module.
}
}
For storing and receiving characters via Bluetooth visit https://forum.arduino.cc/t/receiving-text-strings-over-bluetooth/232452
For other sensor codes visit https://forum.arduino.cc
If you are a starter in Arduino programming visit https://www.arduino.cc/en/Guide/
Always use the "#define and the servo library." code when you are building something with this.
Why did I make this?
I made this because when I was a beginner I struggled using other types of motor controllers. So the problems were,
- Always forget the pins when doing big projects.
- Very messy with a lot of wires connecting Arduino to sensors.
- Not having dedicated sensor connectors.
- Not having an in-built switch, which always meant to connect one externally.
So on this board, I have eliminated all those problems.
What kind of projects can I do with this board?
All sorts of projects! Like
- Obstacle avoiding car
- Line following car
- Motion sensed automatic soap dispenser and much more! Imagination is your only limit!
To Be Noted,
- When soldering, solder the Arduino Uno male pins under the PCB so that they can be used as a shield. Eg:-
- You should know how to program Arduino Uno (example: make a function)
- It's your choice to connect the sensor & module pins with female/male headers.
- After soldering the components, cut off the excess parts.
- Use an I.C. socket base in case you want to replace the I.C.
- No republishing, only use for your work.
- D.P. stands for DigitalPin
- Temperature sensor out D.P. is 11
- TEMP stands for temperature sensor(DHT11 module)
- U.D.S. stands for Ultrasonic Distance Sensor
- BT1 stands for Bluetooth module(compatible HC-05, HC-06 4pin modules)
- Digital IR is an Infrared sensor.
- PIR is a Passive Infrared Sensor/ Motion sensor.
- Use an I2C converter when using the L.C.D. display module.
#include <Servo.h> #define motor1 4 #define motor2 5 #define motor3 6 #define motor4 7 Servo.servo1; Servo.servo2; #define tx 0 #define rx 1 #define trig 2 #define echo 8 #define pir_out 3 #define temp 11 #define ir1 A0 #define ir2 A1 #define sda A4 #define scl A5 void setup() { // put your setup code here, to run once: servo1.attach(9); servo2.attach(10); } void loop() { // put your main code here, to run repeatedly: }
#include <Servo.h> #define motor1 4 #define motor2 5 #define motor3 6 #define motor4 7 Servo.servo1; Servo.servo2; #define tx 0 #define rx 1 #define trig 2 #define echo 8 #define pir_out 3 #define temp 11 #define ir1 A0 #define ir2 A1 #define sda A4 #define scl A5 void setup() { // put your setup code here, to run once: servo1.attach(9); servo2.attach(10); pinMode(motor1, OUTPUT) pinMode(motor2, OUTPUT) pinMode(motor3, OUTPUT) pinMode(motor4, OUTPUT)// telling arduino that the motor pins are output/they are the controlling pins. } void loop() { // put your main code here, to run repeatedly: //1st motor forward/backward code digitalWrite(motor1, HIGH) digitalWrite(motor2, LOW)// runs the 1st motor in a direction (connct the motor to the screw terminal and see where it is rotating, if rotating forward it is forward, if rotating backward it is backward) digitalWrite(motor1, LOW) digitalWrite(motor2, HIGH)// runs the 1st motor in the opposite direction according to the above piece of code. // //2nd motor forward/backward code digitalWrite(motor3, HIGH) digitalWrite(motor4, LOW)// runs the 2nd motor in a direction (connct the motor to the screw terminal and see where it is rotating, if rotating forward it is forward, if rotating backward it is backward) digitalWrite(motor3, LOW) digitalWrite(motor4, HIGH)// runs the 2nd motor in the opposite direction according to the above piece of code. // //1st motor stop code digitalWrite(motor1, LOW) digitalWrite(motor2, LOW)//stops the 1st motor. // //2nd motor stop code digitalWrite(motor3, LOW) digitalWrite(motor4, LOW)//stops the 2nd motor. // //to turn you should make one wheel stop and one running, and then record where its turning. then you can make a function called "turn *the side turned*" and use it on your project. make functions to forward(all two wheels running forward), backward (all two wheels running backward), stop( all two wheels stop) and use them on your project. }
#define motor1 4 #define motor2 5 #define motor3 6 #define motor4 7 #define tx 0 #define rx 1 #define trig 2 #define echo 8 #define pir_out 3 #define temp 11 #define ir1 A0 #define ir2 A1 #define sda A4 #define scl A5 void setup() { // put your setup code here, to run once: pinMode(trig,OUTPUT);//setting pin trig to output pinMode(echo,INPUT);//setting pin echo to input } void loop() { // put your main code here, to run repeatedly: digitalWrite(trig,LOW); delayMicroseconds(2); digitalWrite(trig,HIGH); delayMicroseconds(2); digitalWrite(trig,LOW); long t = pulseIn(echo, HIGH);// starting the sensor long inches = t / 74 / 2; long cm = t / 29 / 2; // getiing the real in/cm value if(cm<10){ //whatever you program here will run if the sensor detects something less than 10cm away from it*sensor*. can replace the above "<" with any symbol and make it "less than", "equal", "equal or less than" or "equal or greater than". } }
#define motor1 4 #define motor2 5 #define motor3 6 #define motor4 7 #define tx 0 #define rx 1 #define trig 2 #define echo 8 #define pir_out 3 #define temp 11 #define ir1 A0 #define ir2 A1 #define sda A4 #define scl A5 void setup() { // put your setup code here, to run once: pinMode(pir_out, INPUT); } void loop() { // put your main code here, to run repeatedly: int val = digitalRead(pir_out); if (val == 1){ //put a function here and it will run when sensor detects motion. } if (val == 0){ //put a function here and it will run when sensor doesn't detect any motion. } }
char ble; #define motor1 4 #define motor2 5 #define motor3 6 #define motor4 7 #define tx 0 #define rx 1 #define trig 2 #define echo 8 #define pir_out 3 #define temp 11 #define ir1 A0 #define ir2 A1 #define sda A4 #define scl A5 void setup() { // put your setup code here, to run once: Serial.begin(9600); } void loop() { // put your main code here, to run repeatedly: if(Serial.available()){ ble = Serial.read(); } if (ble == ' //any number you are going to send through bluetooth '){ //anything you code here gonna run when bluetooth module detects the given number. only numbers supported and requires smartphone to send signals. you can use any android Arduino Bluetooth control app and test the signal using the code below. remember when uploading the code remove the bluetooth module and reconnect it when the uploading is finished. or else the code is not gonna upload or it can severely damage your bluetooth module. } }
Bit-Duino All-in-one robotics shield
*PCBWay community is a sharing platform. We are not responsible for any design issues and parameter issues (board thickness, surface finish, etc.) you choose.
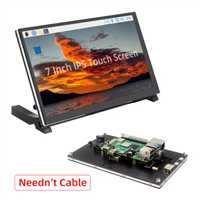
Raspberry Pi 5 7 Inch Touch Screen IPS 1024x600 HD LCD HDMI-compatible Display for RPI 4B 3B+ OPI 5 AIDA64 PC Secondary Screen(Without Speaker)
BUY NOW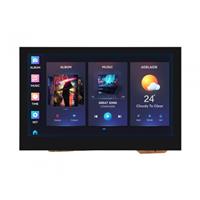
ESP32-S3 4.3inch Capacitive Touch Display Development Board, 800×480, 5-point Touch, 32-bit LX7 Dual-core Processor
BUY NOW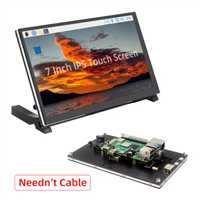
Raspberry Pi 5 7 Inch Touch Screen IPS 1024x600 HD LCD HDMI-compatible Display for RPI 4B 3B+ OPI 5 AIDA64 PC Secondary Screen(Without Speaker)
BUY NOW- Comments(0)
- Likes(2)

- 1 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10

-
10design
-
10usability
-
10creativity
-
10content
More by awesome
-
Arduino Nanuno (Surface Mount version) This is Arduino Nanuno, Using this you can use any type of shield that is compatible with Arduino Un...
-
Arduino Nanuno (Through hole version) This is Arduino Nanuno, Using this you can use any type of shield that is compatible with Arduino Un...
-
Bit-Duino All-in-one robotics shield This Arduino Uno Development shield is made for beginners who can try to program themselves using re...
-
-
-
Modifying a Hotplate to a Reflow Solder Station
752 1 5 -
MPL3115A2 Barometric Pressure, Altitude, and Temperature Sensor
405 0 1 -
-
Nintendo 64DD Replacement Shell
352 0 2 -
V2 Commodore AMIGA USB-C Power Sink Delivery High Efficiency Supply Triple Output 5V ±12V OLED display ATARI compatible shark 100W
974 4 2 -
How to measure weight with Load Cell and HX711
639 0 3