![]() |
arduino IDEArduino
|
IOT PLANT - GROW YOUR PLANTS FROM ANYWHERE AROUND THE WORLD.
Watch the video
The device and plant setup
What about growing any plants from anywhere around the world without your actual presence? Yes, it is possible through this project called 'IoT Plant'.
You can water your plant through different modes available in the android application and you will get the real time reading of moister, temperature, and light that is available for the plant on your smartphone. And also you will get an email when your plant require water, manure, etc...
Let's read more about it -
What is IoT (internet of things)? - simple definition
The Internet of Things is the ecosystem of connected physical devices that are accessible through the internet.
Features of IoT Plant
Cheap price (~4000rs)
You can monitor the real time requirement of the plant.
Different modes of water supply for different types of plants
What you can monitor?
The humidity of the soil.
Light is available to the plant leaves and the temperature of the atmosphere.
Different modes of water supply
Manual mode - You can manually supply the water.
Timer mode - Can be used to supply water at regular intervals of time or at a particular time every day.
Automatic mode - Automatically supply water as per the requirement of the plant. Suitable for the plants which always need water.(eg: Paddy cultivation)
Technical details
This project was made using the microcontroller called Arduino. - It is a cheap open-source board that can be used for different applications by programming using C++ language through the Arduino IDE software. And an IoT board called EP8266 revolutionized the Internet of things. For a mere dollar, you can add a whole world wireless capability to your projects. That is the reason why we are choosing ESP8266 for making this project.
It is used some sensors such as soil moister sensor, temperature sensor, and light sensor to monitor the real-time environment of the plant. Also, a water pump and relay to pump the water to the plant.
For this project, I used an IoT application called 'Blynk'. Through this application, we are controlling the water pump and see the environment or the water requirement of the plant through different types of graphs.
Parts used
Mechanical parts
Arduino nano
ESP8266
ESP8266 programmer
LCD display
Relay module
Plastic box (17 x 11 cm wide)
Ac to DC 12 volt 2 AH regulator.
Water pump
Switch
Circuit diagram
Arduino code (C++ language)
This is the program uploaded to the Arduino board
const int mPin = A1;
const int lPin = A2;
const int tPin = A3;
int mValue = 0;
int lValue = 0;
int tValue = 0;
int moist = 0;
int light = 0;
int temp = 0;
//--
byte datas1 = 0;
byte datas2 = 0;
byte datas3 = 0;
//--
const int motor_in = 2;
const int auto_in = 4;
const int motor_out = 7;
int mstate = 0;
int astate = 0;
//-------LCD--------
#include <Wire.h>
#include <LCD.h>
#include <LiquidCrystal_I2C.h>
#define I2C_ADDR 0x3F // <<----- Add your address here. Find it from I2C Scanner
#define BACKLIGHT_PIN 3
#define En_pin 2
#define Rw_pin 1
#define Rs_pin 0
#define D4_pin 4
#define D5_pin 5
#define D6_pin 6
#define D7_pin 7
LiquidCrystal_I2C lcd(I2C_ADDR,En_pin,Rw_pin,Rs_pin,D4_pin,D5_pin,D6_pin,D7_pin);
//-------LCD--------
void setup() {
Serial.begin(115200);
//-------LCD--------
lcd.begin (16,2); // <<----- My LCD was 16x2
// Switch on the backlight
lcd.setBacklightPin(BACKLIGHT_PIN,POSITIVE);
lcd.setBacklight(HIGH);
lcd.home (); // go home
lcd.print("** IOT PLANT **");
delay(1000);
//-------LCD--------
pinMode(motor_in, INPUT);
pinMode(auto_in, INPUT);
pinMode(motor_out, OUTPUT);
}
void loop() {
// read the analog in value:
mValue = analogRead(mPin);
lValue = analogRead(lPin);
tValue = analogRead(tPin);
moist = map(mValue, 300, 1023, 100, 0);
light = map(lValue, 0, 1023, 100, 0);
temp = (5.0 * tValue * 100.0) / 1024;
mstate = digitalRead(motor_in);
astate = digitalRead(auto_in);
//---------- manuel mode ----
if (mstate == HIGH && astate == LOW)
{
digitalWrite(motor_out, HIGH);
lcd.clear();//***************
lcd.setCursor (0,0); // go to start of 1nd line
lcd.print("Water is pumping");
lcd.setCursor (0,1); // go to start of 2nd line
lcd.print("Moisture:");
lcd.setCursor (9,1);
lcd.print(moist);
lcd.setCursor (11,1);
lcd.print("%");
delay(1000);
}
//---------- automatic mode ----
else if (mstate == LOW && astate == HIGH)
{
if (moist < 25)
{
digitalWrite(motor_out, HIGH);
lcd.clear();//***************
lcd.setCursor (0,0); // go to start of 1nd line
lcd.print("Water is pumping");
lcd.setCursor (0,1); // go to start of 2nd line
lcd.print("Moisture:");
lcd.setCursor (9,1);
lcd.print(moist);
lcd.setCursor (11,1);
lcd.print("%");
delay(1000);
}
else
{
digitalWrite(motor_out, LOW);
lcd.clear();//***************
lcd.setCursor (0,0); // go to start of 1nd line
lcd.print("Water is excess");
lcd.setCursor (0,1); // go to start of 1nd line
lcd.print("motor turned off");
delay(1000);
}
}
else
{
digitalWrite(motor_out, LOW);
//-------------------------------------------
lcd.clear();//*******
lcd.setCursor (0,0); // go to start of 1nd line
lcd.print("Moisture:");
lcd.setCursor (9,0);
lcd.print(moist);
lcd.setCursor (11,0);
lcd.print("%");
lcd.setCursor (0,1); // go to start of 2nd line
lcd.print("Temperature:");
lcd.setCursor (12,1);
lcd.print(temp);
lcd.setCursor (15,1);
lcd.print("C");
delay(1000);
lcd.clear();//***************
lcd.setCursor (0,0); // go to start of 1nd line
lcd.print("Moisture:");
lcd.setCursor (9,0);
lcd.print(moist);
lcd.setCursor (11,0);
lcd.print("%");
lcd.setCursor (0,1); // go to start of 2nd line
lcd.print("Light:");
lcd.setCursor (6,1);
lcd.print(light);
lcd.setCursor (8,1);
lcd.print("%");
delay(1000);
//------------------------------------------------
}
//----------
datas1 = moist & 0xFF;
Serial.write(0x8);
Serial.write(datas1);
datas2 = light & 0xFF;
Serial.write(0x7);
Serial.write(datas2);
datas3 = temp & 0xFF;
Serial.write(0x6);
Serial.write(datas3);
delay(5);
}
ESP8266 code (C++ language)
This is the program uploaded to ESP8266 board.
#include <ESP8266WiFi.h>
#include <BlynkSimpleEsp8266.h>
byte ID = 0;
int data = 0;
// You should get Auth Token in the Blynk App.
// Go to the Project Settings (nut icon).
char auth[] = "49f8b849e38141d48366b93170b7a529";
// Your WiFi credentials.
// Set password to "" for open networks.
char ssid[] = "iotplant";
char pass[] = "12345678";
#define moistpin V1
#define lightpin V5
#define temppin V2
BlynkTimer timer;
BLYNK_CONNECTED() {
Blynk.syncAll();
}
void myTimerEvent()
{
if(Serial.available()>0)
{
ID = Serial.read();
data = Serial.read();
if(ID == 0x6)
{
Blynk.virtualWrite(moistpin, data);
if (data<25)
{
Blynk.email("Subject:* IOT PLANT *", "Plant need water");
Blynk.notify("YOUR PLANT IS THURSTY!");
}
}
if(ID == 0x7)
{
Blynk.virtualWrite(lightpin, data);
}
if(ID == 0x8)
{
Blynk.virtualWrite(temppin, data);
}
}
else
{
Blynk.virtualWrite(V1, 0);
Blynk.virtualWrite(V5, 0);
Blynk.virtualWrite(V2, 0);
}
}
void setup()
{
Serial.begin(115200);
Blynk.begin(auth, ssid, pass);
timer.setInterval(1000L, myTimerEvent);
}
void loop()
{
Blynk.run();
timer.run(); // Initiates BlynkTimer
}
Future plans/updates
Adding a camera to monitor the growth of the plant
Adding a sensor to monitor the nitrogen content in the soil, to supply the manure for the plant.
Future plans/updates
Adding a camera to monitor the growth of the plant
Adding a sensor to monitor the nitrogen content in the soil, to supply the manure for the plant.
Arduino code (C++ language)
This is the program uploaded to the Arduino board
const int mPin = A1;
const int lPin = A2;
const int tPin = A3;
int mValue = 0;
int lValue = 0;
int tValue = 0;
int moist = 0;
int light = 0;
int temp = 0;
//--
byte datas1 = 0;
byte datas2 = 0;
byte datas3 = 0;
//--
const int motor_in = 2;
const int auto_in = 4;
const int motor_out = 7;
int mstate = 0;
int astate = 0;
//-------LCD--------
#include <Wire.h>
#include <LCD.h>
#include <LiquidCrystal_I2C.h>
#define I2C_ADDR 0x3F // <<----- Add your address here. Find it from I2C Scanner
#define BACKLIGHT_PIN 3
#define En_pin 2
#define Rw_pin 1
#define Rs_pin 0
#define D4_pin 4
#define D5_pin 5
#define D6_pin 6
#define D7_pin 7
LiquidCrystal_I2C lcd(I2C_ADDR,En_pin,Rw_pin,Rs_pin,D4_pin,D5_pin,D6_pin,D7_pin);
//-------LCD--------
void setup() {
Serial.begin(115200);
//-------LCD--------
lcd.begin (16,2); // <<----- My LCD was 16x2
// Switch on the backlight
lcd.setBacklightPin(BACKLIGHT_PIN,POSITIVE);
lcd.setBacklight(HIGH);
lcd.home (); // go home
lcd.print("** IOT PLANT **");
delay(1000);
//-------LCD--------
pinMode(motor_in, INPUT);
pinMode(auto_in, INPUT);
pinMode(motor_out, OUTPUT);
}
void loop() {
// read the analog in value:
mValue = analogRead(mPin);
lValue = analogRead(lPin);
tValue = analogRead(tPin);
moist = map(mValue, 300, 1023, 100, 0);
light = map(lValue, 0, 1023, 100, 0);
temp = (5.0 * tValue * 100.0) / 1024;
mstate = digitalRead(motor_in);
astate = digitalRead(auto_in);
//---------- manuel mode ----
if (mstate == HIGH && astate == LOW)
{
digitalWrite(motor_out, HIGH);
lcd.clear();//***************
lcd.setCursor (0,0); // go to start of 1nd line
lcd.print("Water is pumping");
lcd.setCursor (0,1); // go to start of 2nd line
lcd.print("Moisture:");
lcd.setCursor (9,1);
lcd.print(moist);
lcd.setCursor (11,1);
lcd.print("%");
delay(1000);
}
//---------- automatic mode ----
else if (mstate == LOW && astate == HIGH)
{
if (moist < 25)
{
digitalWrite(motor_out, HIGH);
lcd.clear();//***************
lcd.setCursor (0,0); // go to start of 1nd line
lcd.print("Water is pumping");
lcd.setCursor (0,1); // go to start of 2nd line
lcd.print("Moisture:");
lcd.setCursor (9,1);
lcd.print(moist);
lcd.setCursor (11,1);
lcd.print("%");
delay(1000);
}
else
{
digitalWrite(motor_out, LOW);
lcd.clear();//***************
lcd.setCursor (0,0); // go to start of 1nd line
lcd.print("Water is excess");
lcd.setCursor (0,1); // go to start of 1nd line
lcd.print("motor turned off");
delay(1000);
}
}
else
{
digitalWrite(motor_out, LOW);
//-------------------------------------------
lcd.clear();//*******
lcd.setCursor (0,0); // go to start of 1nd line
lcd.print("Moisture:");
lcd.setCursor (9,0);
lcd.print(moist);
lcd.setCursor (11,0);
lcd.print("%");
lcd.setCursor (0,1); // go to start of 2nd line
lcd.print("Temperature:");
lcd.setCursor (12,1);
lcd.print(temp);
lcd.setCursor (15,1);
lcd.print("C");
delay(1000);
lcd.clear();//***************
lcd.setCursor (0,0); // go to start of 1nd line
lcd.print("Moisture:");
lcd.setCursor (9,0);
lcd.print(moist);
lcd.setCursor (11,0);
lcd.print("%");
lcd.setCursor (0,1); // go to start of 2nd line
lcd.print("Light:");
lcd.setCursor (6,1);
lcd.print(light);
lcd.setCursor (8,1);
lcd.print("%");
delay(1000);
//------------------------------------------------
}
//----------
datas1 = moist & 0xFF;
Serial.write(0x8);
Serial.write(datas1);
datas2 = light & 0xFF;
Serial.write(0x7);
Serial.write(datas2);
datas3 = temp & 0xFF;
Serial.write(0x6);
Serial.write(datas3);
delay(5);
}
ESP8266 code (C++ language)
This is the program uploaded to ESP8266 board.
#include <ESP8266WiFi.h>
#include <BlynkSimpleEsp8266.h>
byte ID = 0;
int data = 0;
// You should get Auth Token in the Blynk App.
// Go to the Project Settings (nut icon).
char auth[] = "49f8b849e38141d48366b93170b7a529";
// Your WiFi credentials.
// Set password to "" for open networks.
char ssid[] = "iotplant";
char pass[] = "12345678";
#define moistpin V1
#define lightpin V5
#define temppin V2
BlynkTimer timer;
BLYNK_CONNECTED() {
Blynk.syncAll();
}
void myTimerEvent()
{
if(Serial.available()>0)
{
ID = Serial.read();
data = Serial.read();
if(ID == 0x6)
{
Blynk.virtualWrite(moistpin, data);
if (data<25)
{
Blynk.email("Subject:* IOT PLANT *", "Plant need water");
Blynk.notify("YOUR PLANT IS THURSTY!");
}
}
if(ID == 0x7)
{
Blynk.virtualWrite(lightpin, data);
}
if(ID == 0x8)
{
Blynk.virtualWrite(temppin, data);
}
}
else
{
Blynk.virtualWrite(V1, 0);
Blynk.virtualWrite(V5, 0);
Blynk.virtualWrite(V2, 0);
}
}
void setup()
{
Serial.begin(115200);
Blynk.begin(auth, ssid, pass);
timer.setInterval(1000L, myTimerEvent);
}
void loop()
{
Blynk.run();
timer.run(); // Initiates BlynkTimer
}
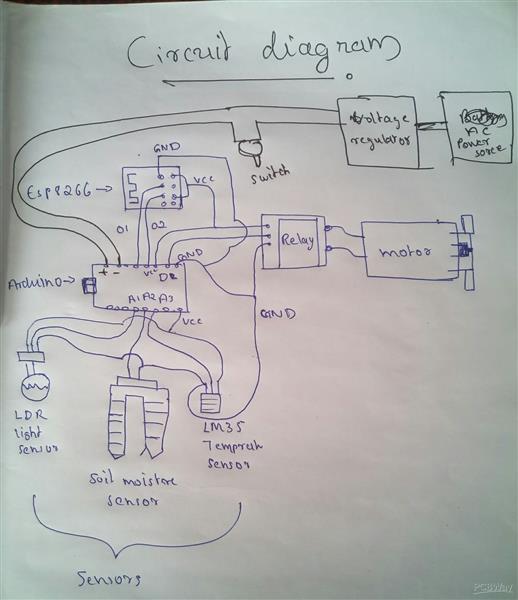
IOT PLANT - GROW YOUR PLANTS FROM ANYWHERE AROUND THE WORLD.
- Comments(0)
- Likes(1)
-
Engineer May 23,2023
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by mohdazhar
-
Automatic Bus Stop Announcement System When we travel by bus ,the route of the bus is unknown to a new passenger. It mostly affects the ill...
-
Earthquake Alert System Based On IOT Many countries have implemented EEW(early earthquake warning) systems to save human lives. The earth...
-
Smart Street Light Smart Street Light spotlights different restrictions and difficulties identified with traditional an...
-
Line Break Detector With Alert System Line Break Detector With Alert System is a mechanism which helps the consumer and KSEB officers to d...
-
Smart Railway Gate Opening System Using IOT The railway crossing accidents are increasing day by day due to human-manned railway crossings. Live...
-
Advanced Biometric Finger Print Scanner We took the case of our hostel mess and concluded that there is no proper way to manage the hostel m...
-
Baby Monitoring System We are very well familiar with the hurdles faced by Parents to nurture their infant and especially i...
-
TOKEN MACHINE AND QUEUE MANAGEMENT SYSTEM FOR HOSPITALS ABSTRACTPatient wait times have a strong influence on patient satisfaction levels. A common scenario...
-
Make your own branded ESP32 development board with PCBWay! What about making your own custom PCB boards with your own branding? Doesn't that sound nice? PCB ma...
-
FOODIE BOT Automation has become an integral part of today's modern life. We are increasingly noticing that mor...
-
A ESP32 BASED BLUETOOTH MINI ROBOT A simple mini robot that you can control with your phone. The robot can be improvised and implemente...
-
IOT smart AC plug A smart plug is a home automation device and is a hot new thing. Several types of smart plugs are av...
-
"A perfectly working line follower robot using arduino" LINE FOLLOWER ROBOT - THE EASIEST!This is a simple tutorial to make a line follower robot using Ardu...
-
IOT PLANT - GROW YOUR PLANTS FROM ANYWHERE AROUND THE WORLD. Watch the video The device and plant setupWhat about growing any plants from anywhere around the wor...
-
HOW TO PROGRAM ESP8266 - 01 In this tutorial, I am going to show how to program ESP8266 - 01 using an Arduino board or using FTD...
-
Make Your First Arduino Robot - the Best Tutorial Out There Smartphone-controlled obstacle avoiding and wall follower robot.Are you a beginner in Arduino and ha...
-
THE ULTIMATE OFFROAD RC ROVER Let's make an all-terrain remote-controlled rover bot. This is a great starter project for hobbyists...
-
MAKE A 3.3 VOLT REGULATOR FOR ESP8266 The 3.3-volt power supply is one of the main issues when we use ESP8266 - 01 as a standalone board. ...
-
-
-
-
-
-
3D printed Enclosure Backplate for Riden RD60xx power supplies
160 1 1 -
-