![]() |
arduino IDEArduino
|
Make Your First Arduino Robot - the Best Tutorial Out There
Smartphone-controlled obstacle avoiding and wall follower robot.
Are you a beginner in Arduino and have a plan to make your first robot then follow these Instructables.
Are you not yet used an Arduino then have a familiar with this by feeling it.
What about making your own robots. It's really cool thing?. But I don't know where to start. Then this instructable is (best) for you. In this tutorial, I will show how to make an Arduino robot with different functionalities. We can learn the use of different programs for different functions, mainly a smartphone controlled robot with Obstacle avoid er, wall follower, or maze solver robot, etc. You can also make it for a single function.
Watch this video to see its final working.
Watch this video to see it's autonomous function.
Download the Bluetooth app from here
Step 1: Materials and Tools.
Materials-
Arduino Uno - Buy here
L293D motor shield - Buy here. "If you are not using the shield but using the l293d IC or module then visit my blog post's last section for the circuit and program."
Hc-sro4 ultrasonic sensor - Buy here
Hc-05 Bluetooth module - Buy here
2 x Gear motor - Buy here
2 x Wheel - Buy here
Caster wheel - Buy here
3 x 9-volt battery
3 x battery clip - Buy here
DC male jack - Buy here
8 x Female to female jumper wire - Buy here
Plastic box (size - 14 cm x 10 cm)
Why choose the l293d motor shield?
It's a low-cost device and easy to use, using a commonly available motor shield that does not require any previous skills. It can be also programmed easily using adafruit library.
Tools-
Screwdriver
Soldering iron
Hot glue gun
Driller
Step 2: Making the Chasis.
'
Make two holes on either side of the box for connecting the motors and three holes on the downside for connecting the caster wheel after measuring and marking the correct diameter. Then connect the motor after soldering the wire to the motor shield and connect the caster wheel.
Step 3: Connecting Battery
Then place two 9-volt batteries inside the box above the caster wheel. Then wire this battery in parallel mode and connect a switch as shown in the above diagram. These are the batteries that give power to the motor shield.
Step 4: Connecting the Arduino and Motor Shield
Connect the motor shield above the Arduino and place it above the battery. Then connect the wires from the motors and battery to the motor shield. Look out for the connection diagram before connecting, as it is very important.
Wiring Instructions -
- Connect the left motor to "M1" of motor shield
- Connect the right motor to "M3" of motor shield
- Connect the the wire from battery to "ext pwr " of motor shield
Don't forget to remove the jumper from the "pwr" of motor shield
Step 5: Make Two Holes and Power the Arduino.
Make two holes for accessing the USB port and DC input port of Arduino. Take another 9-volt battery and connect the battery clip to the dc male jack adaptor, then connect the DC male jack to Arduino DC input port, to power the Arduino. Then place the battery between the motors and Arduino inside the box.
Step 6: Connecting Bluetooth Module and Switch.
Connect the Bluetooth module to Arduino as shown in the connection diagram and place it inside the box. Then make a hole to connect the switch on the backside of the box and connect the switch (here I have changed the switch which is used earlier because of some soldering problem).
Wiring Instruction of Bluetooth module -
- "TX of Bluetooth Module" goes to "RX of Arduino"
- "RX of Bluetooth Module" goes to "TXof Arduino"
- "VCC of Bluetooth Module" goes to "5v of Arduino"
- "GND of Bluetooth Module" goes to "GND of Arduino"
- The State & Key pins of the BT modules are kept unused.
Step 7: Circuit Diagram. Connecting Ultrasonic Sensor and Finishing.
Take the ultrasonic sensor and glue a small 'L' shaped plastic piece to it and glue it over the plastic box(or if not having a glue stick use double sided tape ). Then connect the jumper wires to as shown in the circuit diagram above.
Wiring Instruction of ultrasonic sensor -
- "VCC of Ultra sonic sensor" goes to "5 volt of Arduino"
- "GND of Ultra sonic sensor" goes to "GND of Arduino"
- "Trig pin of Ultra sonic sensor" goes to "Analog pin 1 volt of Arduino"
- "Echo pin of Ultra sonic sensor" goes to "Analog pin 0 of Arduino"
Now we finished the all the connections and it's the time to program.
Step 8: Last Step - Arduino Codes and Bluetooth App Editing
First, download the ARDUINO IDE from Arduino.cc(here) and install it on your PC. Download the above files and Upload it. thus we learned how to program an Arduino easily. I have shown here some programs to work this robot as an obstacle-avoiding robot, Wall follower, and Bluetooth controlled. and at last, I have combined these three functions together. Watch the video for more details and information
You can use the wall follower robot code to function as a maze solver robot.
Install the 'AFmotor library before compiling the program. Copy-paste the AF motor folder to the Arduino libraries folder. Download from here.
Autonomous robot - Obstacle avoiding robot and Wall follower robot code - Video
The Final code- Video
/*
Author: Muhammed Azhar
visit - robotechmaker.com
*/
#include <AFMotor.h>
#define trigPin 9 // define the pins of your sensor
#define echoPin 8
//Objects
AF_DCMotor motorRight(1, MOTOR12_64KHZ); // create motor #1, 64KHz pwm
AF_DCMotor motorLeft(3, MOTOR12_64KHZ); // create motor #3, 64KHz pwm
//Constants and variable
char dataIn = 'S';
char determinant;
char det;
int vel = 255; //Bluetooth Stuff
void setup() {
Serial.begin(9600); // set up Serial library at 9600 bps
pinMode(trigPin, OUTPUT);// set the trig pin to output (Send sound waves)
pinMode(echoPin, INPUT);// set the echo pin to input (recieve sound waves)
//Initalization messages
Serial.println(" Mr.robot");
Serial.println(" Reday for working!");
//turn off motors
motorRight.setSpeed(0);
motorLeft.setSpeed(0);
motorRight.run(RELEASE);
motorLeft.run(RELEASE);
}
void loop() {
det = check(); //call check() subrotine to get the serial code
//serial code analysis
switch (det){
case 'F': // F, move forward
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel);
motorRight.run(FORWARD);
motorLeft.run(FORWARD);
det = check();
break;
case 'B': // B, move back
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel);
motorRight.run(BACKWARD);
motorLeft.run(BACKWARD);
det = check();
break;
case 'L':// L, move wheels left
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel/4);
motorRight.run(FORWARD);
motorLeft.run(FORWARD);
det = check();
break;
case 'R': // R, move wheels right
motorRight.setSpeed(vel/4);
motorLeft.setSpeed(vel);
motorRight.run(FORWARD);
motorLeft.run(FORWARD);
det = check();
break;
case 'I': // I, turn right forward
motorRight.setSpeed(vel/2);
motorLeft.setSpeed(vel);
motorRight.run(FORWARD);
motorLeft.run(FORWARD);
det = check();
break;
case 'J': // J, turn right back
motorRight.setSpeed(vel/2);
motorLeft.setSpeed(vel);
motorRight.run(BACKWARD);
motorLeft.run(BACKWARD);
det = check();
break;
case 'G': // G, turn left forward
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel/2);
motorRight.run(FORWARD);
motorLeft.run(FORWARD);
det = check();
break;
case 'H': // H, turn left back
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel/2);
motorRight.run(BACKWARD);
motorLeft.run(BACKWARD);
det = check();
break;
case 'S':
// S, stop
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel);
motorRight.run(RELEASE);
motorLeft.run(RELEASE);
det = check();
break;
case 'm':
//for wall follower robot.
motorRight.setSpeed(vel); //set the speed of the motors, between 0-255
motorLeft.setSpeed (vel);
long duration, distance; // start the scan
digitalWrite(trigPin, LOW);
delayMicroseconds(2); // delays are required for a succesful sensor operation.
digitalWrite(trigPin, HIGH);
delayMicroseconds(10); //this delay is required as well!
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = (duration/2) / 29.1;// convert the distance to centimeters.
if (distance < 30)/*The distance that need to to keep with the wall */ {
Serial.println ("Wall is ditected!" );
Serial.println (" Started following the wall ");
Serial.println (" Turning !");
motorRight.setSpeed(vel);
motorLeft.setSpeed(0);
motorRight.run(FORWARD);
motorLeft.run(RELEASE);
delay(500); // wait for a second
}
else {
Serial.println ("No Wall detected. turning round");
delay (15);
motorRight.setSpeed(0);
motorLeft.setSpeed(vel);
motorRight.run(RELEASE);
motorLeft.run (FORWARD);
}
break;
case 'b':
//obstacle avoider robot
motorRight.setSpeed(vel); //set the speed of the motors, between 0-255
motorLeft.setSpeed (vel);
long Aduration, Adistance; // start the scan
digitalWrite(trigPin, LOW);
delayMicroseconds(2); // delays are required for a succesful sensor operation.
digitalWrite(trigPin, HIGH);
delayMicroseconds(10); //this delay is required as well!
digitalWrite(trigPin, LOW);
Aduration = pulseIn(echoPin, HIGH);
Adistance = (Aduration/2) / 29.1;// convert the distance to centimeters.
if (Adistance < 25)/*if there's an obstacle 25 centimers, ahead, do the following: */ {
Serial.println ("Close Obstacle detected!" );
Serial.println ("Obstacle Details:");
Serial.print ("Distance From Robot is " );
Serial.print ( Adistance);
Serial.print ( " CM!");// print out the distance in centimeters.
Serial.println (" The obstacle is declared a threat due to close distance. ");
Serial.println (" Turning !");
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel);
motorLeft.run(BACKWARD); // Turn as long as there's an obstacle ahead.
motorRight.run (FORWARD);
}
else {
Serial.println ("No obstacle detected. going forward");
delay (15);
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel);
motorRight.run(FORWARD); //if there's no obstacle ahead, Go Forward!
motorLeft.run(FORWARD);
}
break;
}
}
//get bluetooth code received from serial port
int check(){
if (Serial.available() > 0){// if there is valid data in the serial port
dataIn = Serial.read();// stores data into a varialbe
//check the code
if (dataIn == 'F'){//Forward
determinant = 'F';
}
else if (dataIn == 'B'){//Backward
determinant = 'B';
}
else if (dataIn == 'L'){//Left
determinant = 'L';
}
else if (dataIn == 'R'){//Right
determinant = 'R';
}
else if (dataIn == 'I'){//Froward Right
determinant = 'I';
}
else if (dataIn == 'J'){//Backward Right
determinant = 'J';
}
else if (dataIn == 'G'){//Forward Left
determinant = 'G';
}
else if (dataIn == 'H'){//Backward Left
determinant = 'H';
}
else if (dataIn == 'S'){//Stop
determinant = 'S';
}
else if (dataIn == '0'){//Speed 0
vel = 0;
}
else if (dataIn == '1'){//Speed 25
vel = 25;
}
else if (dataIn == '2'){//Speed 50
vel = 50;
}
else if (dataIn == '3'){//Speed 75
vel = 75;
}
else if (dataIn == '4'){//Speed 100
vel = 100;
}
else if (dataIn == '5'){//Speed 125
vel = 125;
}
else if (dataIn == '6'){//Speed 150
vel = 150;
}
else if (dataIn == '7'){//Speed 175
vel = 175;
}
else if (dataIn == '8'){//Speed 200
vel = 200;
}
else if (dataIn == '9'){//Speed 225
vel = 225;
}
else if (dataIn == 'b'){//Extra On
determinant = 'b';
}
else if (dataIn == 'm'){//Extra On
determinant = 'm';
}
}
return determinant;
}
/*
Author: Marcelo Moraes
This project contains public domain code.
The modification is allowed without notice.
*/
//Libraries
#include <AFMotor.h>
//Objects
AF_DCMotor motorRight(1, MOTOR12_64KHZ); // create motor #1, 64KHz pwm
AF_DCMotor motorLeft(3, MOTOR12_64KHZ); // create motor #3, 64KHz pwm
//Constants and variable
char dataIn = 'S';
char determinant;
char det;
int vel = 0; //Bluetooth Stuff
void setup() {
Serial.begin(9600); // set up Serial library at 9600 bps
//Initalization messages
Serial.println("ArduinoBymyself - ROVERBot");
Serial.println(" AF Motor test!");
//turn off motors
motorRight.setSpeed(0);
motorLeft.setSpeed(0);
motorRight.run(RELEASE);
motorLeft.run(RELEASE);
}
void loop() {
det = check(); //call check() subrotine to get the serial code
//serial code analysis
switch (det){
case 'F': // F, move forward
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel);
motorRight.run(FORWARD);
motorLeft.run(FORWARD);
det = check();
break;
case 'B': // B, move back
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel);
motorRight.run(BACKWARD);
motorLeft.run(BACKWARD);
det = check();
break;
case 'L':// L, move wheels left
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel/4);
motorRight.run(FORWARD);
motorLeft.run(FORWARD);
det = check();
break;
case 'R': // R, move wheels right
motorRight.setSpeed(vel/4);
motorLeft.setSpeed(vel);
motorRight.run(FORWARD);
motorLeft.run(FORWARD);
det = check();
break;
case 'I': // I, turn right forward
motorRight.setSpeed(vel/2);
motorLeft.setSpeed(vel);
motorRight.run(FORWARD);
motorLeft.run(FORWARD);
det = check();
break;
case 'J': // J, turn right back
motorRight.setSpeed(vel/2);
motorLeft.setSpeed(vel);
motorRight.run(BACKWARD);
motorLeft.run(BACKWARD);
det = check();
break;
case 'G': // G, turn left forward
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel/2);
motorRight.run(FORWARD);
motorLeft.run(FORWARD);
det = check();
break;
case 'H': // H, turn left back
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel/2);
motorRight.run(BACKWARD);
motorLeft.run(BACKWARD);
det = check();
break;
case 'S': // S, stop
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel);
motorRight.run(RELEASE);
motorLeft.run(RELEASE);
det = check();
break;
}
}
//get bluetooth code received from serial port
int check(){
if (Serial.available() > 0){// if there is valid data in the serial port
dataIn = Serial.read();// stores data into a varialbe
//check the code
if (dataIn == 'F'){//Forward
determinant = 'F';
}
else if (dataIn == 'B'){//Backward
determinant = 'B';
}
else if (dataIn == 'L'){//Left
determinant = 'L';
}
else if (dataIn == 'R'){//Right
determinant = 'R';
}
else if (dataIn == 'I'){//Froward Right
determinant = 'I';
}
else if (dataIn == 'J'){//Backward Right
determinant = 'J';
}
else if (dataIn == 'G'){//Forward Left
determinant = 'G';
}
else if (dataIn == 'H'){//Backward Left
determinant = 'H';
}
else if (dataIn == 'S'){//Stop
determinant = 'S';
}
else if (dataIn == '0'){//Speed 0
vel = 0;
}
else if (dataIn == '1'){//Speed 25
vel = 25;
}
else if (dataIn == '2'){//Speed 50
vel = 50;
}
else if (dataIn == '3'){//Speed 75
vel = 75;
}
else if (dataIn == '4'){//Speed 100
vel = 100;
}
else if (dataIn == '5'){//Speed 125
vel = 125;
}
else if (dataIn == '6'){//Speed 150
vel = 150;
}
else if (dataIn == '7'){//Speed 175
vel = 175;
}
else if (dataIn == '8'){//Speed 200
vel = 200;
}
else if (dataIn == '9'){//Speed 225
vel = 225;
}
else if (dataIn == 'q'){//Speed 255
vel = 255;
}
else if (dataIn == 'U'){//Back Lights On
determinant = 'U';
}
else if (dataIn == 'u'){//Back Lights Off
determinant = 'u';
}
else if (dataIn == 'W'){//Front Lights On
determinant = 'W';
}
else if (dataIn == 'w'){//Front Lights Off
determinant = 'w';
}
else if (dataIn == 'V'){//Horn On
determinant = 'V';
}
else if (dataIn == 'v'){//Horn Off
determinant = 'v';
}
else if (dataIn == 'X'){//Extra On
determinant = 'X';
}
else if (dataIn == 'x'){//Extra Off
determinant = 'x';
}
}
return determinant;
}
/*
Author: Muhammed Azhar
visit - robotechmaker.com
*/
#include <AFMotor.h>
#define trigPin 9 // define the pins of your sensor
#define echoPin 8
int vel = 255; // Speed of the robot
//Objects
AF_DCMotor motorRight(1, MOTOR12_64KHZ); // create motor #1, 64KHz pwm
AF_DCMotor motorLeft(3, MOTOR12_64KHZ); // create motor #3, 64KHz pwm
void setup() {
Serial.begin(9600); // set up Serial library at 9600 bps
pinMode(trigPin, OUTPUT);// set the trig pin to output (Send sound waves)
pinMode(echoPin, INPUT);// set the echo pin to input (recieve sound waves)
//Initalization messages
Serial.println(" Mr.robot");
Serial.println(" Reday for working!");
//turn off motors
motorRight.setSpeed(0);
motorLeft.setSpeed(0);
motorRight.run(RELEASE);
motorLeft.run(RELEASE);
}
void loop() {
//obstacle avoider robot
motorRight.setSpeed(vel); //set the speed of the motors, between 0-255
motorLeft.setSpeed (vel);
long duration, distance; // start the scan
digitalWrite(trigPin, LOW);
delayMicroseconds(2); // delays are required for a succesful sensor operation.
digitalWrite(trigPin, HIGH);
delayMicroseconds(10); //this delay is required as well!
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = (duration/2) / 29.1;// convert the distance to centimeters.
if (distance < 25)/*if there's an obstacle 25 centimers, ahead, do the following: */ {
Serial.println ("Close Obstacle detected!" );
Serial.println ("Obstacle Details:");
Serial.print ("Distance From Robot is " );
Serial.print ( distance);
Serial.print ( " CM!");// print out the distance in centimeters.
Serial.println (" The obstacle is declared a threat due to close distance. ");
Serial.println (" Turning !");
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel);
motorLeft.run(BACKWARD); // Turn as long as there's an obstacle ahead.
motorRight.run (FORWARD);
}
else {
Serial.println ("No obstacle detected. going forward");
delay (15);
motorRight.setSpeed(vel);
motorLeft.setSpeed(vel);
motorRight.run(FORWARD); //if there's no obstacle ahead, Go Forward!
motorLeft.run(FORWARD);
}
}
/*
Author: Muhammed Azhar
visit - robotechmaker.com
*/
#include <AFMotor.h>
#define trigPin 9 // define the pins of your sensor
#define echoPin 8
int vel = 255; // Speed of the robot
//Objects
AF_DCMotor motorRight(1, MOTOR12_64KHZ); // create motor #1, 64KHz pwm
AF_DCMotor motorLeft(3, MOTOR12_64KHZ); // create motor #3, 64KHz pwm
void setup() {
Serial.begin(9600); // set up Serial library at 9600 bps
pinMode(trigPin, OUTPUT);// set the trig pin to output (Send sound waves)
pinMode(echoPin, INPUT);// set the echo pin to input (recieve sound waves)
//Initalization messages
Serial.println(" Mr.robot");
Serial.println(" Reday for working!");
//turn off motors
motorRight.setSpeed(0);
motorLeft.setSpeed(0);
motorRight.run(RELEASE);
motorLeft.run(RELEASE);
}
void loop() {
//for wall follower robot.
motorRight.setSpeed(vel); //set the speed of the motors, between 0-255
motorLeft.setSpeed (vel);
long duration, distance; // start the scan
digitalWrite(trigPin, LOW);
delayMicroseconds(2); // delays are required for a succesful sensor operation.
digitalWrite(trigPin, HIGH);
delayMicroseconds(10); //this delay is required as well!
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = (duration/2) / 29.1;// convert the distance to centimeters.
if (distance < 30)/*The distance that need to to keep with the wall */ {
Serial.println ("Wall is ditected!" );
Serial.println (" Started following the wall ");
Serial.println (" Turning !");
motorRight.setSpeed(vel);
motorLeft.setSpeed(0);
motorRight.run(FORWARD);
motorLeft.run(RELEASE);
delay(500); // wait for a second
}
else {
Serial.println ("No Wall detected. turning round");
delay (15);
motorRight.setSpeed(0);
motorLeft.setSpeed(vel);
motorRight.run(RELEASE);
motorLeft.run (FORWARD);
}
}
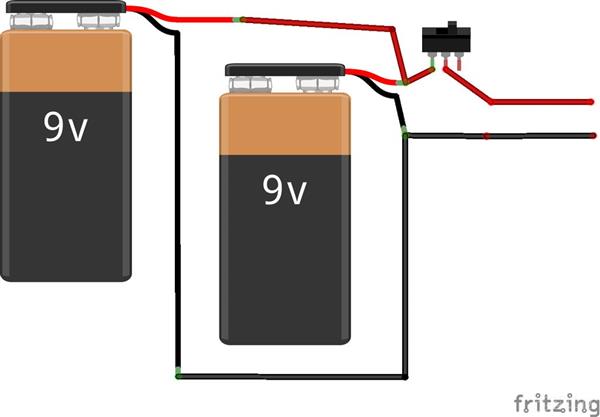
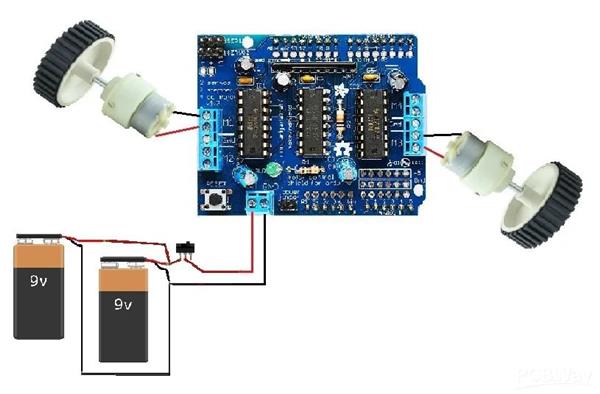
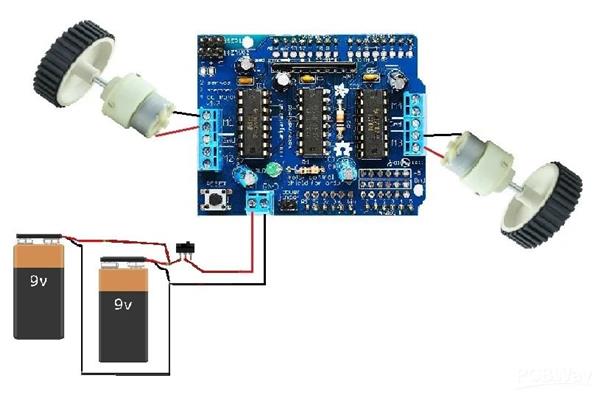
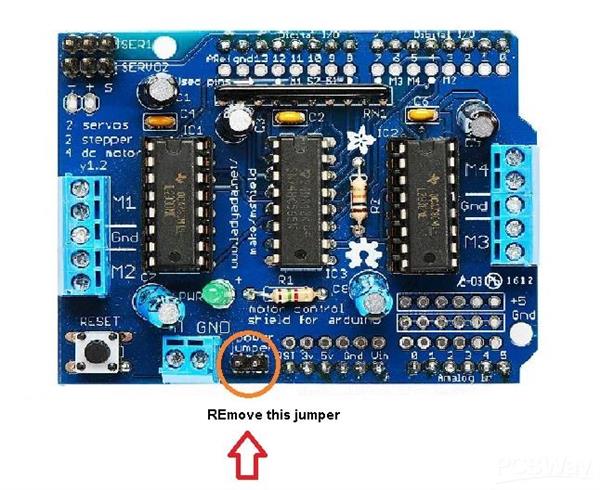
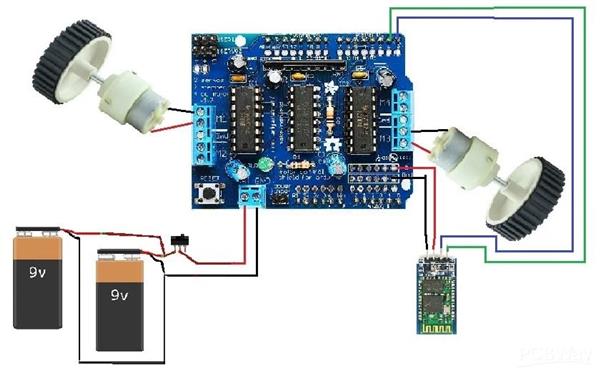
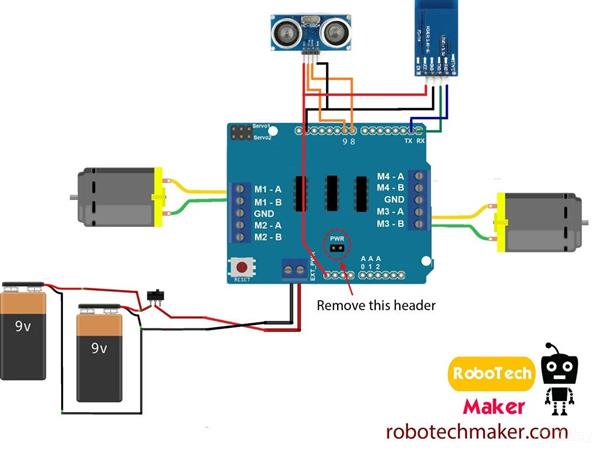
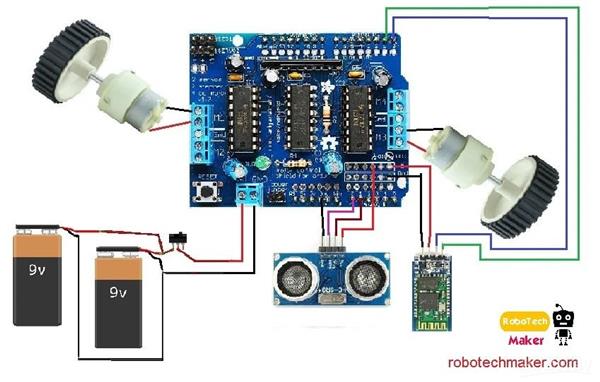
Make Your First Arduino Robot - the Best Tutorial Out There
- Comments(0)
- Likes(0)
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by mohdazhar
-
Automatic Bus Stop Announcement System When we travel by bus ,the route of the bus is unknown to a new passenger. It mostly affects the ill...
-
Earthquake Alert System Based On IOT Many countries have implemented EEW(early earthquake warning) systems to save human lives. The earth...
-
Smart Street Light Smart Street Light spotlights different restrictions and difficulties identified with traditional an...
-
Line Break Detector With Alert System Line Break Detector With Alert System is a mechanism which helps the consumer and KSEB officers to d...
-
Smart Railway Gate Opening System Using IOT The railway crossing accidents are increasing day by day due to human-manned railway crossings. Live...
-
Advanced Biometric Finger Print Scanner We took the case of our hostel mess and concluded that there is no proper way to manage the hostel m...
-
Baby Monitoring System We are very well familiar with the hurdles faced by Parents to nurture their infant and especially i...
-
TOKEN MACHINE AND QUEUE MANAGEMENT SYSTEM FOR HOSPITALS ABSTRACTPatient wait times have a strong influence on patient satisfaction levels. A common scenario...
-
Make your own branded ESP32 development board with PCBWay! What about making your own custom PCB boards with your own branding? Doesn't that sound nice? PCB ma...
-
FOODIE BOT Automation has become an integral part of today's modern life. We are increasingly noticing that mor...
-
A ESP32 BASED BLUETOOTH MINI ROBOT A simple mini robot that you can control with your phone. The robot can be improvised and implemente...
-
IOT smart AC plug A smart plug is a home automation device and is a hot new thing. Several types of smart plugs are av...
-
"A perfectly working line follower robot using arduino" LINE FOLLOWER ROBOT - THE EASIEST!This is a simple tutorial to make a line follower robot using Ardu...
-
IOT PLANT - GROW YOUR PLANTS FROM ANYWHERE AROUND THE WORLD. Watch the video The device and plant setupWhat about growing any plants from anywhere around the wor...
-
HOW TO PROGRAM ESP8266 - 01 In this tutorial, I am going to show how to program ESP8266 - 01 using an Arduino board or using FTD...
-
Make Your First Arduino Robot - the Best Tutorial Out There Smartphone-controlled obstacle avoiding and wall follower robot.Are you a beginner in Arduino and ha...
-
THE ULTIMATE OFFROAD RC ROVER Let's make an all-terrain remote-controlled rover bot. This is a great starter project for hobbyists...
-
MAKE A 3.3 VOLT REGULATOR FOR ESP8266 The 3.3-volt power supply is one of the main issues when we use ESP8266 - 01 as a standalone board. ...
-
-
-
kmMiniSchield MIDI I/O - IN/OUT/THROUGH MIDI extension for kmMidiMini
125 0 0 -
DIY Laser Power Meter with Arduino
173 0 2 -
-
-
Box & Bolt, 3D Printed Cardboard Crafting Tools
164 0 2 -