![]() |
arduino IDEArduino
|
THE ULTIMATE OFFROAD RC ROVER
Let's make an all-terrain remote-controlled rover bot. This is a great starter project for hobbyists. In this tutorial, I am showing how to make an all-terrain robot using Arduino with two modes of control. Using a smartphone and the other one is, using our own designed RF remote. It is easier and more flexible to use and also low cost. The total cost of making this robot is less than 60$.
Areas or fields of its application(with the updated version 2.0)
- Disaster management.
- Spying agent for military purposes
- Rainwater drainage system cleaning robot
- Repairing pipelines that humans can't access
- In nuclear plant disasters
Controlling mods
This is the remote controller of this robot. It is made up of a low-cost NRF2401 RF transceiver to transmit the data from the remote to the robot.
2. The Bluetooth controlling.
In this option, we can use our smartphone for controlling the robot.
Watch this video to see the V2.0 of the robot.(Control using a smartphone)
Step 1: Materials Needed
Electronic parts
- Arduino Uno - Amazon.in or Amazon.com
- Dual DC motor driver 20A - robokits.co.in or robokitsworld.com
- NRF2401 - Amazon.in or Amazon.com
- HC-05 Bluetooth module (Only for Bluetooth controlling option) - Amazon.in or Amazon.com
- Jumper wires - Amazon.in or Amazon.com
Mechanical parts
- 2 x Metal gear motor - robokits.co.in or robokitsworld.com
- 2 x Gear motor - robokits.co.in or robokitsworld.com
- 4 x Wheel - robokits.co.in
- SwitchLIPO 2600 mah battery - Amazon.in or Amazon.com
- 3 x 'L' shaped clamp for plastic gear motor - robokits.co.in or robokitsworld.com
- 2 x 'L' shaped clamp for metal gear motor - robokits.co.in or robokitsworld.com
- Aluminium sheet 2 mm (from a local store)
Tools
- Screwdriver
- Wirecutter
- Cutter tool
- Soldering iron
- Drilling machine(not important)
Step 2: Making the Chassis.
Let's make the chassis of this rover. I am using two '3mm thick' & 20x15 cm aluminium sheets to make the chassis.
First, drill the holes for placing the 'L' shaped clamp for connecting the motors. Here I used two 200 rpm metal gear motors (for the backside of the rover) and two 200 rpm plastic gear motors (on the front side). Then connect the 4 motors and take out wires from the motors.
"Download and Zoom in the circuit diagram for a clear view"
Wiring instruction' NRF2401' >> 'ARDUINO'
- VCC >> VCC
- GND >> GND
- MISO >> PIN 12
- MOSI >> PIN 11
- SCN >> PIN10
- SCK >> PIN 13
- CE >> PIN 9
- IRQ (not in use)
If you are using the Bluetooth controlling option then,
Wiring instruction of HC-05 Bluetooth module >> Arduino
- TX of HC-05 >> RX of Arduino
- RX of HC-05 >> TX of Arduino
- VCC of HC-05 >> 5volt of arduino
- GND of HC-05 >> GND of Arduino
Read about the motor controller in the next step.
Step 4: Connecting All Parts Together and Testing.
Then connect all the parts together as shown in the circuit diagram, before attaching this item to the chassis make sure everything is working fine. You can find the codes needed for the rover in the next step of this tutorial.
The motor controller used
The motor driver used here was purchased from the robotkits.co.in. We can control it either by analog input or by PWM input. Which is a low cost and 6V - 18V compatible 20A capable Dual DC motor driver. It is ideal for this application where two motors (I am using 4 motors, by the parallel connection of two motors on the same side) are required for up to 20 Amperes of current during startup and during normal operations. Read more about this motor driver in this PDF here.
Step 5: Last Step Adding a Switch and Finishing. (Arduino Code)
In this last step, we are connecting the battery and an on/off switch for the rover. After connecting the switch and battery as shown in the figure. Close the upper side using another 20x15 cm size aluminium sheet and at last connect the wheels also. Now we have finished the making of the rover bot.
Battery used
In this robot, I use a LiPo 11.1 volt 2800mah 30 C battery. For choosing your own battery read this article. This is my first LiPo battery therefore I don't know much about it.
Updates in the rover
I updated this robot later by adding a 3 DOF robotic arm and an ultrasonic sensor. To see it's working watch the video below. And the tutorial of that will be posted soon on my blog.
Arduino codes
Here you can download the codes. The first code is for controlling the robot using the custom made remote. For the code used in the transmitter(Remote) visit the tutorial on making a remote -Click here to visit that tutorial. In this Arduino code, there is a library called print.h. For using that library in the Arduino code you need to add the print.h file and Arduino code in the same folder that I have provided here.
The second code is for Bluetooth controlling option, using this application - Click here to download the application.
#include <Servo.h> #include <SPI.h> #include "nRF24L01.h" #include "RF24.h" #include "printf.h" // Set up nRF24L01 radio on SPI bus plus pins 9 & 10 RF24 radio(9,10); int stop1=2; int stop2=4; int back1=5; int back2=7; int pwm1=6; int pwm2=3; // Single radio pipe address for the 2 nodes to communicate. const uint64_t pipe = 0xE8E8F0F0E1LL; // // Payload // uint8_t received_data[2]; uint8_t num_received_data =sizeof(received_data); // // Setup // void setup(void) { delay(2000); //wait until the esc starts in case of Arduino got power first pinMode(6, OUTPUT); pinMode(3, OUTPUT); pinMode(stop1, OUTPUT); pinMode(stop2, OUTPUT); pinMode(back1, OUTPUT); pinMode(back2, OUTPUT); // // Print preamble // Serial.begin(57600); printf_begin(); // // Setup and configure rf radio // radio.begin(); //Begin operation of the chip. // This simple sketch opens a single pipes for these two nodes to communicate // back and forth. One listens on it, the other talks to it. radio.openReadingPipe(1,pipe); radio.startListening(); // // Dump the configuration of the rf unit for debugging // radio.printDetails(); } void Stop(void) { analogWrite(pwm1, 0); analogWrite(pwm2, 0); } void Forward1(void) { analogWrite(pwm1, 255); digitalWrite(back1, LOW); digitalWrite(back2, LOW); } void Forward2(void){ analogWrite(pwm2, 255); digitalWrite(back1, LOW); digitalWrite(back2, LOW); } void Backward1(void){ analogWrite(pwm1, 255); digitalWrite(back1, HIGH); } void Backward2(void){ analogWrite(pwm2, 255); digitalWrite(back2, HIGH); } void loop(void) { // if there is data ready if ( radio.available() ) { bool done = false; int value1; int value2; while (!done) { // Fetch the payload, and see if this was the last one. done = radio.read( received_data, num_received_data ); value1=received_data[0]; //Multiplication by 10 because the ESC operates for vlues around 1500 and the nRF24L01 can transmit maximum of 255 per packet value2=received_data[1]; //Multiplication by 10 because the ESC operates for vlues around 1500 and the nRF24L01 can transmit maximum of 255 per packet //analogWrite(pwm2,value2); Serial.println("value2="); Serial.println(value2); Serial.println("value1="); Serial.println(value1); if(value1<170 && value1>140 || value2>70 && value2<100){ Stop(); } if(value1>170 && value1!=0){ Forward1(); } if(value2<70 && value2!=0){ Forward2(); } if(value1<140 && value1!=0){ Backward1(); } if(value2>100 && value2!=0){ Backward2(); } } } }
#include <Servo.h> //Constants and variable int stop1=2; int stop2=4; int back1=5; int back2=7; int pwm1=6; int pwm2=3; char dataIn = 'S'; char determinant; char det; int vel = 255; //Bluetooth Stuff void setup() { Serial.begin(9600); // set up Serial library at 9600 bps pinMode(6, OUTPUT); pinMode(3, OUTPUT); pinMode(stop1, OUTPUT); pinMode(stop2, OUTPUT); pinMode(back1, OUTPUT); pinMode(back2, OUTPUT); //Initalization messages Serial.println("ArduinoBymyself - ROVERBot"); Serial.println(" AF Motor test!"); } void loop() { det = check(); //call check() subrotine to get the serial code //serial code analysis switch (det){ case 'F': // F, move forward analogWrite(pwm1,vel); digitalWrite(back1, LOW); digitalWrite(back2, LOW); analogWrite(pwm2, vel); digitalWrite(back1, LOW); digitalWrite(back2, LOW); det = check(); break; //------- case 'B': // B, move back analogWrite(pwm1, vel); digitalWrite(back1, HIGH); analogWrite(pwm2, vel); digitalWrite(back2, HIGH); det = check(); break; //----- case 'L':// L, move wheels left analogWrite(pwm1,vel); digitalWrite(back1, LOW); analogWrite(pwm2, vel); digitalWrite(back2, HIGH); det = check(); break; //---------- case 'R': // R, move wheels right analogWrite(pwm2, vel); digitalWrite(back2, LOW); analogWrite(pwm1, vel); digitalWrite(back1, HIGH); det = check(); break; //----- case 'I': // I, turn right forward analogWrite(pwm1,0); digitalWrite(back1, LOW); digitalWrite(back2, LOW); analogWrite(pwm2, vel); digitalWrite(back1, LOW); digitalWrite(back2, LOW); det = check(); break; case 'J': // J, turn right back analogWrite(pwm1,vel); digitalWrite(back1, HIGH); analogWrite(pwm2,0); digitalWrite(back2, HIGH); det = check(); break; case 'G': // G, turn left forward analogWrite(pwm1,vel); digitalWrite(back1, LOW); digitalWrite(back2, LOW); analogWrite(pwm2,0); digitalWrite(back1, LOW); digitalWrite(back2, LOW); det = check(); break; case 'H': // H, turn left back analogWrite(pwm1,0); digitalWrite(back1, HIGH); analogWrite(pwm2, vel); digitalWrite(back2, HIGH); det = check(); break; case 'S': // R, move wheels right analogWrite(pwm1, 0); analogWrite(pwm2, 0); digitalWrite(back1, LOW); digitalWrite(back2, LOW); det = check(); break; } } //get bluetooth code received from serial port int check(){ if (Serial.available() > 0){// if there is valid data in the serial port dataIn = Serial.read();// stores data into a varialbe //check the code if (dataIn == 'F'){//Forward determinant = 'F'; } else if (dataIn == 'B'){//Backward determinant = 'B'; } else if (dataIn == 'L'){//Left determinant = 'L'; } else if (dataIn == 'R'){//Right determinant = 'R'; } else if (dataIn == 'I'){//Froward Right determinant = 'I'; } else if (dataIn == 'J'){//Backward Right determinant = 'J'; } else if (dataIn == 'G'){//Forward Left determinant = 'G'; } else if (dataIn == 'H'){//Backward Left determinant = 'H'; } else if (dataIn == 'S'){//Stop determinant = 'S'; } else if (dataIn == '0'){//Speed 0 vel = 0; } else if (dataIn == '1'){//Speed 25 vel = 25; } else if (dataIn == '2'){//Speed 50 vel = 50; } else if (dataIn == '3'){//Speed 75 vel = 75; } else if (dataIn == '4'){//Speed 100 vel = 100; } else if (dataIn == '5'){//Speed 125 vel = 125; } else if (dataIn == '6'){//Speed 150 vel = 150; } else if (dataIn == '7'){//Speed 175 vel = 175; } else if (dataIn == '8'){//Speed 200 vel = 200; } else if (dataIn == '9'){//Speed 225 vel = 225; } else if (dataIn == 'A'){//Speed 255 vel = 255; } else if (dataIn == 'X'){//Extra On determinant = 'X'; } } return determinant; }
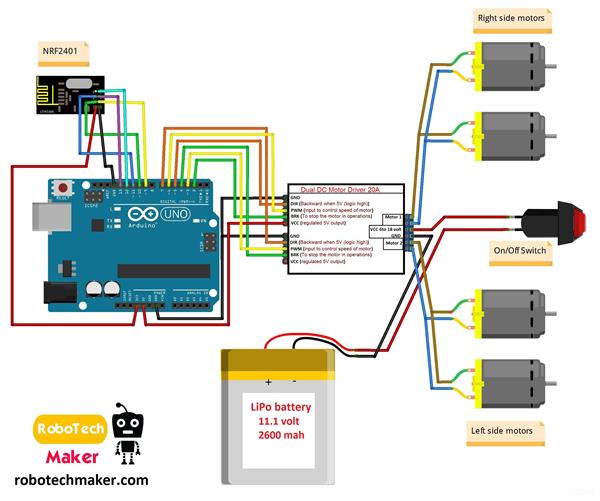
THE ULTIMATE OFFROAD RC ROVER
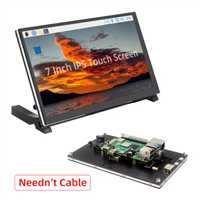
Raspberry Pi 5 7 Inch Touch Screen IPS 1024x600 HD LCD HDMI-compatible Display for RPI 4B 3B+ OPI 5 AIDA64 PC Secondary Screen(Without Speaker)
BUY NOW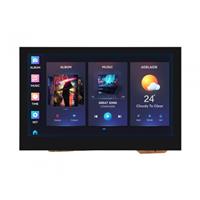
ESP32-S3 4.3inch Capacitive Touch Display Development Board, 800×480, 5-point Touch, 32-bit LX7 Dual-core Processor
BUY NOW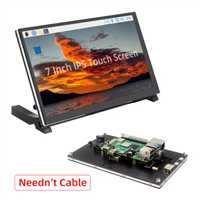
Raspberry Pi 5 7 Inch Touch Screen IPS 1024x600 HD LCD HDMI-compatible Display for RPI 4B 3B+ OPI 5 AIDA64 PC Secondary Screen(Without Speaker)
BUY NOW- Comments(0)
- Likes(0)

- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by mohdazhar
-
"A perfectly working line follower robot using arduino" LINE FOLLOWER ROBOT - THE EASIEST!This is a simple tutorial to make a line follower robot using Ardu...
-
IOT PLANT - GROW YOUR PLANTS FROM ANYWHERE AROUND THE WORLD. Watch the video The device and plant setupWhat about growing any plants from anywhere around the wor...
-
HOW TO PROGRAM ESP8266 - 01 In this tutorial, I am going to show how to program ESP8266 - 01 using an Arduino board or using FTD...
-
Make Your First Arduino Robot - the Best Tutorial Out There Smartphone-controlled obstacle avoiding and wall follower robot.Are you a beginner in Arduino and ha...
-
THE ULTIMATE OFFROAD RC ROVER Let's make an all-terrain remote-controlled rover bot. This is a great starter project for hobbyists...
-
MAKE A 3.3 VOLT REGULATOR FOR ESP8266 The 3.3-volt power supply is one of the main issues when we use ESP8266 - 01 as a standalone board. ...
-
Automatic Bus Stop Announcement System When we travel by bus ,the route of the bus is unknown to a new passenger. It mostly affects the ill...
-
Earthquake Alert System Based On IOT Many countries have implemented EEW(early earthquake warning) systems to save human lives. The earth...
-
Smart Street Light Smart Street Light spotlights different restrictions and difficulties identified with traditional an...
-
Line Break Detector With Alert System Line Break Detector With Alert System is a mechanism which helps the consumer and KSEB officers to d...
-
Smart Railway Gate Opening System Using IOT The railway crossing accidents are increasing day by day due to human-manned railway crossings. Live...
-
Advanced Biometric Finger Print Scanner We took the case of our hostel mess and concluded that there is no proper way to manage the hostel m...
-
Baby Monitoring System We are very well familiar with the hurdles faced by Parents to nurture their infant and especially i...
-
TOKEN MACHINE AND QUEUE MANAGEMENT SYSTEM FOR HOSPITALS ABSTRACTPatient wait times have a strong influence on patient satisfaction levels. A common scenario...
-
Make your own branded ESP32 development board with PCBWay! What about making your own custom PCB boards with your own branding? Doesn't that sound nice? PCB ma...
-
FOODIE BOT Automation has become an integral part of today's modern life. We are increasingly noticing that mor...
-
A ESP32 BASED BLUETOOTH MINI ROBOT A simple mini robot that you can control with your phone. The robot can be improvised and implemente...
-
IOT smart AC plug A smart plug is a home automation device and is a hot new thing. Several types of smart plugs are av...
-
"A perfectly working line follower robot using arduino" LINE FOLLOWER ROBOT - THE EASIEST!This is a simple tutorial to make a line follower robot using Ardu...
-
IOT PLANT - GROW YOUR PLANTS FROM ANYWHERE AROUND THE WORLD. Watch the video The device and plant setupWhat about growing any plants from anywhere around the wor...
-
HOW TO PROGRAM ESP8266 - 01 In this tutorial, I am going to show how to program ESP8266 - 01 using an Arduino board or using FTD...
-
Make Your First Arduino Robot - the Best Tutorial Out There Smartphone-controlled obstacle avoiding and wall follower robot.Are you a beginner in Arduino and ha...
-
THE ULTIMATE OFFROAD RC ROVER Let's make an all-terrain remote-controlled rover bot. This is a great starter project for hobbyists...
-
MAKE A 3.3 VOLT REGULATOR FOR ESP8266 The 3.3-volt power supply is one of the main issues when we use ESP8266 - 01 as a standalone board. ...
-
Automatic Bus Stop Announcement System When we travel by bus ,the route of the bus is unknown to a new passenger. It mostly affects the ill...
-
Earthquake Alert System Based On IOT Many countries have implemented EEW(early earthquake warning) systems to save human lives. The earth...
-
Smart Street Light Smart Street Light spotlights different restrictions and difficulties identified with traditional an...
-
Line Break Detector With Alert System Line Break Detector With Alert System is a mechanism which helps the consumer and KSEB officers to d...
-
Smart Railway Gate Opening System Using IOT The railway crossing accidents are increasing day by day due to human-manned railway crossings. Live...
-
Advanced Biometric Finger Print Scanner We took the case of our hostel mess and concluded that there is no proper way to manage the hostel m...
-
-
Commodore 64 1541-II 1581 Floppy Disk Drive C64 Power Supply Unit USB-C 5V 12V DIN connector 5.25
277 1 3 -
Easy to print simple stacking organizer with drawers
106 0 0 -
-
-
-
-
-
-
Modifying a Hotplate to a Reflow Solder Station
1195 1 6 -
MPL3115A2 Barometric Pressure, Altitude, and Temperature Sensor
679 0 1 -