![]() |
arduino IDEArduino
|
Earthquake Alert System Based On IOT
Many countries have implemented EEW(early earthquake warning) systems to save human lives. The earthquake early warning and rapid response system in Istanbul represents one of the few examples of an operational earthquake warning system. It is impossible to know where and when a seismic event can happen, thus to alleviate damages to infrastructure and human lives, early detection where a real-time architecture and efficient communication become a requirement. An Earthquake alert system can be implemented by means of an IOT in a wireless sensor network (WSN). As the P wave travels faster than the Swave and reaches the sensor it helps in cause an early warning signal. Sensor values are connected to the database by NodeMcu and it is stored in the ThingSpeak account. ThingSpeak account allows to cluster, aggregate and visualize the live data streams in the cloud. With an acceleration sensor, the realistic value of earthquakes can be recorded and warning signals could be sent to registered stations with the help of a wi-fi module on a web server.
PROBLEM STATEMENT
The sudden arrival of earthquake will threaten the lives of people and causes several potential damages. Hence early detection of earthquakes is essential. This will helps people and authorities to resist this disaster.
MATERIALS AND METHODS
Hardware
- Arduino Uno
- Lcd Display 16x4
- MPU6050 Sensor
- ESP8266
- Buzzer
- Led
Software
- Arduino Ide
- Fritzing
- ThinkSpeak
Block Diagram
Flowchart
Step 1 : Follow The Circuit And Construct
Step 2 : Follow The Codes Provided
Code for Sensor
#include <LiquidCrystal.h>
#include <Wire.h>
#include <MPU6050.h>
#define minval -5
#define maxval 3
MPU6050 mpu;
#define led 10
#define buzzer 9
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
void setup()
{ lcd.begin(16, 2);
Serial.begin(115200);
pinMode(led,OUTPUT);
pinMode(buzzer,OUTPUT);
lcd.print(" EarthQuake ");
lcd.setCursor(0, 1);
lcd.print(" Detector");
delay (2000);
lcd.clear();
// Initialize MPU6050
Serial.println("Initialize MPU6050");
while(!mpu.begin(MPU6050_SCALE_2000DPS, MPU6050_RANGE_2G))
{ Serial.println("Could not find a valid MPU6050 sensor, check wiring!");
delay(500);}
mpu.setThreshold(3);
// Check settings
checkSettings();
}
void checkSettings()
{
Serial.println();
Serial.print(" * Sleep Mode: ");
Serial.println(mpu.getSleepEnabled() ? "Enabled" : "Disabled");
Serial.print(" * Clock Source: ");
switch(mpu.getClockSource())
{case MPU6050_CLOCK_KEEP_RESET: Serial.println("Stops the clock and keeps the timing generator in reset"); break;
case MPU6050_CLOCK_EXTERNAL_19MHZ: Serial.println("PLL with external 19.2MHz reference"); break;
case MPU6050_CLOCK_EXTERNAL_32KHZ: Serial.println("PLL with external 32.768kHz reference"); break;
case MPU6050_CLOCK_PLL_ZGYRO: Serial.println("PLL with Z axis gyroscope reference"); break;
case MPU6050_CLOCK_PLL_YGYRO: Serial.println("PLL with Y axis gyroscope reference"); break;
case MPU6050_CLOCK_PLL_XGYRO: Serial.println("PLL with X axis gyroscope reference"); break;
case MPU6050_CLOCK_INTERNAL_8MHZ: Serial.println("Internal 8MHz oscillator"); break;
}
Serial.print(" * Gyroscope: ");
switch(mpu.getScale())
{case MPU6050_SCALE_2000DPS: Serial.println("2000 dps"); break;
case MPU6050_SCALE_1000DPS: Serial.println("1000 dps"); break;
case MPU6050_SCALE_500DPS: Serial.println("500 dps"); break;
case MPU6050_SCALE_250DPS: Serial.println("250 dps"); break;}
Serial.print(" * Gyroscope offsets: ");
Serial.print(mpu.getGyroOffsetX());
Serial.print(" / ");
Serial.print(mpu.getGyroOffsetY());
Serial.print(" / ");
Serial.println(mpu.getGyroOffsetZ());
Serial.println();}
void loop()
{ Vector rawGyro = mpu.readRawGyro();
Vector normGyro = mpu.readNormalizeGyro();
Serial.print(" Xraw = ");
Serial.print(rawGyro.XAxis);
Serial.print(" Yraw = ");
Serial.print(rawGyro.YAxis);
Serial.print(" Zraw = ");
Serial.println(rawGyro.ZAxis);
if(normGyro.XAxis > maxval || normGyro.XAxis < minval && normGyro.YAxis > maxval || normGyro.YAxis < minval && normGyro.ZAxis > maxval || normGyro.ZAxis < minval)
{ digitalWrite(led,HIGH);
digitalWrite(buzzer,HIGH);
delay(300);
digitalWrite(led,HIGH);
digitalWrite(buzzer,HIGH);
delay(300);
lcd.clear();
lcd.print("***EarthQuake***");
lcd.setCursor(0, 2);
String value = "Dt:";
value += normGyro.XAxis;
value.remove(value.length()-4, 3);
value+=", ";
value += normGyro.YAxis;
value.remove(value.length()-4, 3);
value+=", ";
value += normGyro.ZAxis;
value.remove(value.length()-4, 3);
value+=", ";
lcd.print(value);
delay (1000);
lcd.clear();}
else{digitalWrite(led,LOW);
digitalWrite(buzzer,LOW);}
Serial.print(" Xnorm = ");
Serial.print(normGyro.XAxis);
Serial.print(" Ynorm = ");
Serial.print(normGyro.YAxis);
Serial.print(" Znorm = ");
Serial.println(normGyro.ZAxis);
delay(10);}
Code for ThinkSpeak
#include "ThingSpeak.h"
#include <ESP8266WiFi.h>
char networkname[] = "iot"; // network name
char passcode[] = "123456789"; // passcode
WiFiClient client;
unsigned long tsChannelID =1813484; // ThingSpeak Channel ID
const char * tsWriteAPIKey = "QT6XI1AUPMC34JNO"; //ThingSpeak Write API Key
int warning = 0;
const int fieldOne = 1;
const int buttonPin = 5; // the number of the pushbutton pin
int buttonState = 0; // variable for reading the pushbutton status
void setup()
{
Serial.begin(115200);
WiFi.mode(WIFI_STA);//client
ThingSpeak.begin(client);
connectWifi();
pinMode(buttonPin, INPUT);
Serial.println("");
Serial.println("Wifi connected");
}
void loop()
{
buttonState = digitalRead(buttonPin);
if(buttonState == HIGH){
Serial.println("EARTH QUAKE");
warning = 1;
pushData();
delay(15000);
warning = 0;
pushData();
delay(15000);
}
}
void connectWifi()
{
if (WiFi.status() != WL_CONNECTED)
{
Serial.print("Connecting wifi..");
while (WiFi.status() != WL_CONNECTED)
{
WiFi.begin(networkname, passcode);
Serial.print(".");
delay(5000);
}
}
}
void pushData()
{
int getData = ThingSpeak.writeField(tsChannelID, fieldOne, warning, tsWriteAPIKey);
if (getData != 200)
{
Serial.println("Error");
delay(15000);
pushData();
} else {Serial.println("Updated");}
warning = 0;
}
#include <LiquidCrystal.h> #include <Wire.h> #include <MPU6050.h> #define minval -5 #define maxval 3 MPU6050 mpu; #define led 10 #define buzzer 9 LiquidCrystal lcd(12, 11, 5, 4, 3, 2); void setup() { lcd.begin(16, 2); Serial.begin(115200); pinMode(led,OUTPUT); pinMode(buzzer,OUTPUT); lcd.print(" EarthQuake "); lcd.setCursor(0, 1); lcd.print(" Detector"); delay (2000); lcd.clear(); // Initialize MPU6050 Serial.println("Initialize MPU6050"); while(!mpu.begin(MPU6050_SCALE_2000DPS, MPU6050_RANGE_2G)) { Serial.println("Could not find a valid MPU6050 sensor, check wiring!"); delay(500);} mpu.setThreshold(3); // Check settings checkSettings(); } void checkSettings() { Serial.println(); Serial.print(" * Sleep Mode: "); Serial.println(mpu.getSleepEnabled() ? "Enabled" : "Disabled"); Serial.print(" * Clock Source: "); switch(mpu.getClockSource()) {case MPU6050_CLOCK_KEEP_RESET: Serial.println("Stops the clock and keeps the timing generator in reset"); break; case MPU6050_CLOCK_EXTERNAL_19MHZ: Serial.println("PLL with external 19.2MHz reference"); break; case MPU6050_CLOCK_EXTERNAL_32KHZ: Serial.println("PLL with external 32.768kHz reference"); break; case MPU6050_CLOCK_PLL_ZGYRO: Serial.println("PLL with Z axis gyroscope reference"); break; case MPU6050_CLOCK_PLL_YGYRO: Serial.println("PLL with Y axis gyroscope reference"); break; case MPU6050_CLOCK_PLL_XGYRO: Serial.println("PLL with X axis gyroscope reference"); break; case MPU6050_CLOCK_INTERNAL_8MHZ: Serial.println("Internal 8MHz oscillator"); break; } Serial.print(" * Gyroscope: "); switch(mpu.getScale()) {case MPU6050_SCALE_2000DPS: Serial.println("2000 dps"); break; case MPU6050_SCALE_1000DPS: Serial.println("1000 dps"); break; case MPU6050_SCALE_500DPS: Serial.println("500 dps"); break; case MPU6050_SCALE_250DPS: Serial.println("250 dps"); break;} Serial.print(" * Gyroscope offsets: "); Serial.print(mpu.getGyroOffsetX()); Serial.print(" / "); Serial.print(mpu.getGyroOffsetY()); Serial.print(" / "); Serial.println(mpu.getGyroOffsetZ()); Serial.println();} void loop() { Vector rawGyro = mpu.readRawGyro(); Vector normGyro = mpu.readNormalizeGyro(); Serial.print(" Xraw = "); Serial.print(rawGyro.XAxis); Serial.print(" Yraw = "); Serial.print(rawGyro.YAxis); Serial.print(" Zraw = "); Serial.println(rawGyro.ZAxis); if(normGyro.XAxis > maxval || normGyro.XAxis < minval && normGyro.YAxis > maxval || normGyro.YAxis < minval && normGyro.ZAxis > maxval || normGyro.ZAxis < minval) { digitalWrite(led,HIGH); digitalWrite(buzzer,HIGH); delay(300); digitalWrite(led,HIGH); digitalWrite(buzzer,HIGH); delay(300); lcd.clear(); lcd.print("***EarthQuake***"); lcd.setCursor(0, 2); String value = "Dt:"; value += normGyro.XAxis; value.remove(value.length()-4, 3); value+=", "; value += normGyro.YAxis; value.remove(value.length()-4, 3); value+=", "; value += normGyro.ZAxis; value.remove(value.length()-4, 3); value+=", "; lcd.print(value); delay (1000); lcd.clear();} else{digitalWrite(led,LOW); digitalWrite(buzzer,LOW);} Serial.print(" Xnorm = "); Serial.print(normGyro.XAxis); Serial.print(" Ynorm = "); Serial.print(normGyro.YAxis); Serial.print(" Znorm = "); Serial.println(normGyro.ZAxis); delay(10);}
#include "ThingSpeak.h" #include <ESP8266WiFi.h> char networkname[] = "iot"; // network name char passcode[] = "123456789"; // passcode WiFiClient client; unsigned long tsChannelID =1813484; // ThingSpeak Channel ID const char * tsWriteAPIKey = "QT6XI1AUPMC34JNO"; //ThingSpeak Write API Key int warning = 0; const int fieldOne = 1; const int buttonPin = 5; // the number of the pushbutton pin int buttonState = 0; // variable for reading the pushbutton status void setup() { Serial.begin(115200); WiFi.mode(WIFI_STA);//client ThingSpeak.begin(client); connectWifi(); pinMode(buttonPin, INPUT); Serial.println(""); Serial.println("Wifi connected"); } void loop() { buttonState = digitalRead(buttonPin); if(buttonState == HIGH){ Serial.println("EARTH QUAKE"); warning = 1; pushData(); delay(15000); warning = 0; pushData(); delay(15000); } } void connectWifi() { if (WiFi.status() != WL_CONNECTED) { Serial.print("Connecting wifi.."); while (WiFi.status() != WL_CONNECTED) { WiFi.begin(networkname, passcode); Serial.print("."); delay(5000); } } } void pushData() { int getData = ThingSpeak.writeField(tsChannelID, fieldOne, warning, tsWriteAPIKey); if (getData != 200) { Serial.println("Error"); delay(15000); pushData(); } else {Serial.println("Updated");} warning = 0; }
Earthquake Alert System Based On IOT
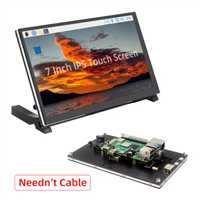
Raspberry Pi 5 7 Inch Touch Screen IPS 1024x600 HD LCD HDMI-compatible Display for RPI 4B 3B+ OPI 5 AIDA64 PC Secondary Screen(Without Speaker)
BUY NOW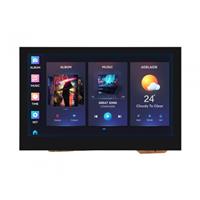
ESP32-S3 4.3inch Capacitive Touch Display Development Board, 800×480, 5-point Touch, 32-bit LX7 Dual-core Processor
BUY NOW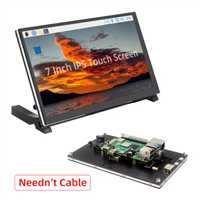
Raspberry Pi 5 7 Inch Touch Screen IPS 1024x600 HD LCD HDMI-compatible Display for RPI 4B 3B+ OPI 5 AIDA64 PC Secondary Screen(Without Speaker)
BUY NOW- Comments(0)
- Likes(2)

-
Engineer Oct 01,2024
-
Sebastian Mackowiak Mar 21,2023
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by mohdazhar
-
"A perfectly working line follower robot using arduino" LINE FOLLOWER ROBOT - THE EASIEST!This is a simple tutorial to make a line follower robot using Ardu...
-
IOT PLANT - GROW YOUR PLANTS FROM ANYWHERE AROUND THE WORLD. Watch the video The device and plant setupWhat about growing any plants from anywhere around the wor...
-
HOW TO PROGRAM ESP8266 - 01 In this tutorial, I am going to show how to program ESP8266 - 01 using an Arduino board or using FTD...
-
Make Your First Arduino Robot - the Best Tutorial Out There Smartphone-controlled obstacle avoiding and wall follower robot.Are you a beginner in Arduino and ha...
-
THE ULTIMATE OFFROAD RC ROVER Let's make an all-terrain remote-controlled rover bot. This is a great starter project for hobbyists...
-
MAKE A 3.3 VOLT REGULATOR FOR ESP8266 The 3.3-volt power supply is one of the main issues when we use ESP8266 - 01 as a standalone board. ...
-
Automatic Bus Stop Announcement System When we travel by bus ,the route of the bus is unknown to a new passenger. It mostly affects the ill...
-
Earthquake Alert System Based On IOT Many countries have implemented EEW(early earthquake warning) systems to save human lives. The earth...
-
Smart Street Light Smart Street Light spotlights different restrictions and difficulties identified with traditional an...
-
Line Break Detector With Alert System Line Break Detector With Alert System is a mechanism which helps the consumer and KSEB officers to d...
-
Smart Railway Gate Opening System Using IOT The railway crossing accidents are increasing day by day due to human-manned railway crossings. Live...
-
Advanced Biometric Finger Print Scanner We took the case of our hostel mess and concluded that there is no proper way to manage the hostel m...
-
Baby Monitoring System We are very well familiar with the hurdles faced by Parents to nurture their infant and especially i...
-
TOKEN MACHINE AND QUEUE MANAGEMENT SYSTEM FOR HOSPITALS ABSTRACTPatient wait times have a strong influence on patient satisfaction levels. A common scenario...
-
Make your own branded ESP32 development board with PCBWay! What about making your own custom PCB boards with your own branding? Doesn't that sound nice? PCB ma...
-
FOODIE BOT Automation has become an integral part of today's modern life. We are increasingly noticing that mor...
-
A ESP32 BASED BLUETOOTH MINI ROBOT A simple mini robot that you can control with your phone. The robot can be improvised and implemente...
-
IOT smart AC plug A smart plug is a home automation device and is a hot new thing. Several types of smart plugs are av...
-
"A perfectly working line follower robot using arduino" LINE FOLLOWER ROBOT - THE EASIEST!This is a simple tutorial to make a line follower robot using Ardu...
-
IOT PLANT - GROW YOUR PLANTS FROM ANYWHERE AROUND THE WORLD. Watch the video The device and plant setupWhat about growing any plants from anywhere around the wor...
-
HOW TO PROGRAM ESP8266 - 01 In this tutorial, I am going to show how to program ESP8266 - 01 using an Arduino board or using FTD...
-
Make Your First Arduino Robot - the Best Tutorial Out There Smartphone-controlled obstacle avoiding and wall follower robot.Are you a beginner in Arduino and ha...
-
THE ULTIMATE OFFROAD RC ROVER Let's make an all-terrain remote-controlled rover bot. This is a great starter project for hobbyists...
-
MAKE A 3.3 VOLT REGULATOR FOR ESP8266 The 3.3-volt power supply is one of the main issues when we use ESP8266 - 01 as a standalone board. ...
-
Automatic Bus Stop Announcement System When we travel by bus ,the route of the bus is unknown to a new passenger. It mostly affects the ill...
-
Earthquake Alert System Based On IOT Many countries have implemented EEW(early earthquake warning) systems to save human lives. The earth...
-
Smart Street Light Smart Street Light spotlights different restrictions and difficulties identified with traditional an...
-
Line Break Detector With Alert System Line Break Detector With Alert System is a mechanism which helps the consumer and KSEB officers to d...
-
Smart Railway Gate Opening System Using IOT The railway crossing accidents are increasing day by day due to human-manned railway crossings. Live...
-
Advanced Biometric Finger Print Scanner We took the case of our hostel mess and concluded that there is no proper way to manage the hostel m...
-
Modifying a Hotplate to a Reflow Solder Station
176 0 2 -
MPL3115A2 Barometric Pressure, Altitude, and Temperature Sensor
132 0 1 -
-
Nintendo 64DD Replacement Shell
184 0 1 -
V2 Commodore AMIGA USB-C Power Sink Delivery High Efficiency Supply Triple Output 5V ±12V OLED display ATARI compatible shark 100W
377 4 1 -
How to measure weight with Load Cell and HX711
423 0 3 -
-
Instrumentation Input, high impedance with 16 bit 1MSPS ADC for SPI
531 0 0