![]() |
Arduino Pro Mini 328 - 5V/16MHz |
x 1 | |
![]() |
604-WP56BID LEDs PTH Red Blinking LED 625nm, 40 mcdKingbright
|
x 5 | |
![]() |
Resistor Through Hole 0.4W 100ohms 0.1% |
x 5 |
![]() |
arduino IDEArduino
|
Make a simple persistence-of-vision (POV) display with an Arduino
Here is a simple project using an Arduino and some LEDs. What we are going to learn here today is how to make a POV display or Persistence-Of-Vision display. It is made out of just 6$ worth of components. This tutorial gives will teach you how to make a simple and cheap Arduino POV display. We can use this display as a pocket-sized portable message showing device and tabletop clock.
How Does the POV Display Work?
What is POV(Persistence of Vision)? It can be defined in simple words. When a person sees an object, its image remains in the retina of the eye for a time interval of 1/16th of a second. This phenomenon is known as the persistence of vision. This phenomenon is used in the POV Display to form images. We turn the LEDs on and off in such a way that the different images overlap each other forming letters. For example:
1 2 3 <-- Time
1 1 1 <-- Bulb 1
1 0 0 <-- Bulb 2
1 1 1 <-- Bulb 3
1 0 0 <-- Bulb 4
1 1 1 <-- Bulb 5
Each row represents the 5 LEDs we use to make the Arduino POV display and each column is a time interval. Each element in the row represents the state of the LED at that given time.
At t = 1 Bulbs 1,2,3,4,5 are ON
At t = 2 Bulbs 1,3,5 are ON
This way we can visually see the letter E formed by the LEDs but the time interval would be very small in milliseconds. Due to the short time intervals and the ability of the LEDs to turn ON and OFF very quickly we can see the letter E as all the 3 images merge. As the motor is spinning and time passes, each LED moves from one position to the next, so all these images merge together.
Parts required:
- Arduino pro mini
- Prefboard
- Female socket pin for Arduino
- LED 5Pcs. (here I showed green led but. red led is better than green led)
- 220ohm Resistor 5Pcs
- Lipo/Old mobile battery
- 100rpm gear motor and wheel (for rotary mechanism)
Circuit diagram:
Cut the perf board to the appropriate size. Then connect the LEDs and solder them as shown in the figure below. Then connect the Arduino case (where Arduino is to be placed). Connect the resistors to the positive pins of the LEDs and connect the negative pins together. Then connect the LEDs to the Arduino. The first LED(on the upper side) connects to the Arduino pin 2. Connect the second LED to the Arduino 3rd pin. Connect all LEDs as shown in the circuit diagram. At last, connect a pin for the mobile battery. For plug and play use, connect a switch. If you don’t have a mobile battery, you can also use a 9-volt battery. In this circuit, I have connected a 9-volt battery to the Arduino with a 3V regulator.
Rotary mechanism:
Here I've used the part of an old UPS as the plastic tin. Make a hole in the centre. Then screw the motor there.
Finally, connect the display to the gear motor wheel. Then you have finished the hardware.
Program the Arduino:
Here I'm programming the Arduino pro mini using the ‘FTDI’ USB serial converter if you don’t have this then you can do it using Arduino UNO board click here to see that.
After uploading the code. Now all you need to do is power up the module and the motor used to turn the whole system. And then you would be able to see "Hello World" lighting up when the motor reaches the correct rpm as shown below:
Output:
My work desk at that time :) (2015)
int delayTime = 1;
int charBreak = 2.1;
int LED1 = 2;
int LED2 = 3;
int LED3 = 4;
int LED4 = 5;
int LED5 = 6;
void setup()
{
pinMode(LED1, OUTPUT);
pinMode(LED2, OUTPUT);
pinMode(LED3, OUTPUT);
pinMode(LED4, OUTPUT);
pinMode(LED5, OUTPUT);
}
int a[] = {1, 6, 26, 6, 1};
int b[] = {31, 21, 21, 10, 0};
int c2[] = {14, 17, 17, 10, 0};
int d[] = {31, 17, 17, 14, 0};
int e[] = {31, 21, 21, 17, 0};
int f[] = {31, 20, 20, 16, 0};
int g[] = {14, 17, 19, 10, 0};
int h[] = {31, 4, 4, 4, 31};
int i[] = {0, 17, 31, 17, 0};
int j[] = {0, 17, 30, 16, 0};
int k[] = {31, 4, 10, 17, 0};
int l[] = {31, 1, 1, 1, 0};
int m[] = {31, 12, 3, 12, 31};
int n[] = {31, 12, 3, 31, 0};
int o[] = {14, 17, 17, 14, 0};
int p[] = {31, 20, 20, 8, 0};
int q[] = {14, 17, 19, 14, 2};
int r[] = {31, 20, 22, 9, 0};
int s[] = {8, 21, 21, 2, 0};
int t[] = {16, 16, 31, 16, 16};
int u[] = {30, 1, 1, 30, 0};
int v[] = {24, 6, 1, 6, 24};
int w[] = {28, 3, 12, 3, 28};
int x[] = {17, 10, 4, 10, 17};
int y[] = {17, 10, 4, 8, 16};
int z[] = {19, 21, 21, 25, 0};
int eos[] = {0, 1, 0, 0, 0};
int excl[] = {0, 29, 0, 0, 0};
int ques[] = {8, 19, 20, 8, 0};
void displayLine(int line)
{
int myline;
myline = line;
if (myline>=16) {digitalWrite(LED1, HIGH); myline-=16;} else {digitalWrite(LED1, LOW);}
if (myline>=8) {digitalWrite(LED2, HIGH); myline-=8;} else {digitalWrite(LED2, LOW);}
if (myline>=4) {digitalWrite(LED3, HIGH); myline-=4;} else {digitalWrite(LED3, LOW);}
if (myline>=2) {digitalWrite(LED4, HIGH); myline-=2;} else {digitalWrite(LED4, LOW);}
if (myline>=1) {digitalWrite(LED5, HIGH); myline-=1;} else {digitalWrite(LED5, LOW);}
}
void displayChar(char c)
{
if (c == 'a'){for (int i = 0; i <5; i++){displayLine(a[i]);delay(delayTime);}displayLine(0);}
if (c == 'b'){for (int i = 0; i <5; i++){displayLine(b[i]);delay(delayTime);}displayLine(0);}
if (c == 'c2'){for (int i = 0; i <5; i++){displayLine(c2[i]);delay(delayTime);}displayLine(0);}
if (c == 'd'){for (int i = 0; i <5; i++){displayLine(d[i]);delay(delayTime);}displayLine(0);}
if (c == 'e'){for (int i = 0; i <5; i++){displayLine(e[i]);delay(delayTime);}displayLine(0);}
if (c == 'f'){for (int i = 0; i <5; i++){displayLine(f[i]);delay(delayTime);}displayLine(0);}
if (c == 'g'){for (int i = 0; i <5; i++){displayLine(g[i]);delay(delayTime);}displayLine(0);}
if (c == 'h'){for (int i = 0; i <5; i++){displayLine(h[i]);delay(delayTime);}displayLine(0);}
if (c == 'i'){for (int it = 0; it <5; it++){displayLine(i[it]);delay(delayTime);}displayLine(0);}
if (c == 'j'){for (int i = 0; i <5; i++){displayLine(j[i]);delay(delayTime);}displayLine(0);}
if (c == 'k'){for (int i = 0; i <5; i++){displayLine(k[i]);delay(delayTime);}displayLine(0);}
if (c == 'l'){for (int i = 0; i <5; i++){displayLine(l[i]);delay(delayTime);}displayLine(0);}
if (c == 'm'){for (int i = 0; i <5; i++){displayLine(m[i]);delay(delayTime);}displayLine(0);}
if (c == 'n'){for (int i = 0; i <5; i++){displayLine(n[i]);delay(delayTime);}displayLine(0);}
if (c == 'o'){for (int i = 0; i <5; i++){displayLine(o[i]);delay(delayTime);}displayLine(0);}
if (c == 'p'){for (int i = 0; i <5; i++){displayLine(p[i]);delay(delayTime);}displayLine(0);}
if (c == 'q'){for (int i = 0; i <5; i++){displayLine(q[i]);delay(delayTime);}displayLine(0);}
if (c == 'r'){for (int i = 0; i <5; i++){displayLine(r[i]);delay(delayTime);}displayLine(0);}
if (c == 's'){for (int i = 0; i <5; i++){displayLine(s[i]);delay(delayTime);}displayLine(0);}
if (c == 't'){for (int i = 0; i <5; i++){displayLine(t[i]);delay(delayTime);}displayLine(0);}
if (c == 'u'){for (int i = 0; i <5; i++){displayLine(u[i]);delay(delayTime);}displayLine(0);}
if (c == 'v'){for (int i = 0; i <5; i++){displayLine(v[i]);delay(delayTime);}displayLine(0);}
if (c == 'w'){for (int i = 0; i <5; i++){displayLine(w[i]);delay(delayTime);}displayLine(0);}
if (c == 'x'){for (int i = 0; i <5; i++){displayLine(x[i]);delay(delayTime);}displayLine(0);}
if (c == 'y'){for (int i = 0; i <5; i++){displayLine(y[i]);delay(delayTime);}displayLine(0);}
if (c == 'z'){for (int i = 0; i <5; i++){displayLine(z[i]);delay(delayTime);}displayLine(0);}
if (c == '!'){for (int i = 0; i <5; i++){displayLine(excl[i]);delay(delayTime);}displayLine(0);}
if (c == '?'){for (int i = 0; i <5; i++){displayLine(ques[i]);delay(delayTime);}displayLine(0);}
if (c == '.'){for (int i = 0; i <5; i++){displayLine(eos[i]);delay(delayTime);}displayLine(0);}
delay(charBreak);
}
void displayString(char* s)
{
for (int i = 0; i<=strlen(s); i++)
{
displayChar(s[i]);
}
}
void loop()
{
displayString("hello world")
}
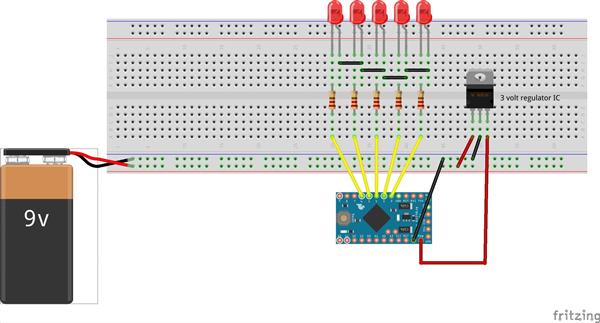
Make a simple persistence-of-vision (POV) display with an Arduino
- Comments(0)
- Likes(3)
-
Orlando Sian Apr 14,2022
-
mohdazhar Apr 13,2022
-
(DIY) C64iSTANBUL Apr 13,2022
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by mohdazhar
-
Automatic Bus Stop Announcement System When we travel by bus ,the route of the bus is unknown to a new passenger. It mostly affects the ill...
-
Earthquake Alert System Based On IOT Many countries have implemented EEW(early earthquake warning) systems to save human lives. The earth...
-
Smart Street Light Smart Street Light spotlights different restrictions and difficulties identified with traditional an...
-
Line Break Detector With Alert System Line Break Detector With Alert System is a mechanism which helps the consumer and KSEB officers to d...
-
Smart Railway Gate Opening System Using IOT The railway crossing accidents are increasing day by day due to human-manned railway crossings. Live...
-
Advanced Biometric Finger Print Scanner We took the case of our hostel mess and concluded that there is no proper way to manage the hostel m...
-
Baby Monitoring System We are very well familiar with the hurdles faced by Parents to nurture their infant and especially i...
-
TOKEN MACHINE AND QUEUE MANAGEMENT SYSTEM FOR HOSPITALS ABSTRACTPatient wait times have a strong influence on patient satisfaction levels. A common scenario...
-
Make your own branded ESP32 development board with PCBWay! What about making your own custom PCB boards with your own branding? Doesn't that sound nice? PCB ma...
-
FOODIE BOT Automation has become an integral part of today's modern life. We are increasingly noticing that mor...
-
A ESP32 BASED BLUETOOTH MINI ROBOT A simple mini robot that you can control with your phone. The robot can be improvised and implemente...
-
IOT smart AC plug A smart plug is a home automation device and is a hot new thing. Several types of smart plugs are av...
-
"A perfectly working line follower robot using arduino" LINE FOLLOWER ROBOT - THE EASIEST!This is a simple tutorial to make a line follower robot using Ardu...
-
IOT PLANT - GROW YOUR PLANTS FROM ANYWHERE AROUND THE WORLD. Watch the video The device and plant setupWhat about growing any plants from anywhere around the wor...
-
HOW TO PROGRAM ESP8266 - 01 In this tutorial, I am going to show how to program ESP8266 - 01 using an Arduino board or using FTD...
-
Make Your First Arduino Robot - the Best Tutorial Out There Smartphone-controlled obstacle avoiding and wall follower robot.Are you a beginner in Arduino and ha...
-
THE ULTIMATE OFFROAD RC ROVER Let's make an all-terrain remote-controlled rover bot. This is a great starter project for hobbyists...
-
MAKE A 3.3 VOLT REGULATOR FOR ESP8266 The 3.3-volt power supply is one of the main issues when we use ESP8266 - 01 as a standalone board. ...
-
TEKTRONIX THS710,THS720,THS730 External Battery Charger with 3D Printed Case
28 1 0 -
-
Atomic Force Microscope - electronic part
96 0 0 -