![]() |
arduino IDEArduino
|
|
|
CLP Arduino SketchLegion2
|
|
![]() |
ESP-PROGEspressif
|
Home Weather Station
Home Weather Station
Introduction
The home Weather Station is a DIY weather Station that collects the following data and uploads the data to firebase:-
Air Quality Index
Compressed Natural Gas (CNG)
Liquid Petroleum Gas (LPG)
Smoke
Rain
Temperature
Humidity
Heat Index
A weather station is a facility, either on land or sea, with instruments and equipment for measuring atmospheric conditions to provide information for weather forecasts and to study the weather and climate. The measurements taken include temperature, atmospheric pressure, humidity, wind speed, wind direction, and precipitation amounts. Wind measurements are taken with as few other obstructions as possible, while temperature and humidity measurements are kept free from direct solar radiation, or insolation. Manual observations are taken at least once daily, while automated measurements are taken at least once an hour.
Weather conditions out at sea are taken by ships and buoys, which measure slightly different meteorological quantities such as sea surface temperature (SST), wave height, and wave period. Drifting weather buoys outnumber their moored versions by a significant amount.
Personal weather station
Roof-mounted weather station instruments
A personal weather station is a set of weather measuring instruments operated by a private individual, club, association, or business (where obtaining and distributing weather data is not a part of the entity's business operation). Personal weather stations have become more advanced and can include many different sensors to measure weather conditions. These sensors can vary between models but most measure wind speed, wind direction, outdoor and indoor temperatures, outdoor and indoor humidity, barometric pressure, rainfall, and UV or solar radiation. Other available sensors can measure soil moisture, soil temperature, and leaf wetness. The quality, number of instruments, and placement of personal weather stations can vary widely, making the determination of which stations collect accurate, meaningful, and comparable data difficult. There are a comprehensive number of retail weather stations available.
Personal weather stations typically involve a digital console that provides readouts of the data being collected. These consoles may interface to a personal computer where data can be displayed, stored, and uploaded to websites or data ingestion/distribution systems. Open-source weather stations are available that are designed to be fully customizable by users.
Personal weather stations may be operated solely for the enjoyment and education of the owner, while some owners share their results with others. They do this by manually compiling data and distributing it, distributing data over the Internet, or sharing data via amateur radio. The Citizen Weather Observer Program (CWOP) is a service which facilitates the sharing of information from personal weather stations. This data is submitted through use of software, a personal computer, and internet connection (or amateur radio) and are utilized by groups such as the National Weather Service (NWS) when generating forecast models. Each weather station submitting data to CWOP will also have an individual Web page that depicts the data submitted by that station. The Weather Underground Internet site is another popular destination for the submittal and sharing of data with others around the world. As with CWOP, each station submitting data to Weather Underground has a unique Web page displaying their submitted data. The UK Met Office's Weather Observations Website (WOW) also allows such data to be shared and displayed.
Features
Collects AQI, CNG, LPG, smoke, temperature, humidty, rain presence and heat index. The values from gas sensors are converted to PPM. Thank you for Bharath Kinnera for this Gas sensor PPM conversion video.
Stores the data in a log file and Firebase.
Dispays the data in a LCD display.
A telegram bot for fetching values.
Folder Structure
arduino_nano_code: Contains the embedded C code for flashing to the arduino nano board.
arduino_uno_code: Contains the embedded C code for flashing to the arduino uno board.
docs: Contains images of the product.
heroku_code/telegram-bot: Telegram bot python code for Heroku server.
telebot
init.py: Library initialisation.
app.py: Main program for telegram bot.
Procfile: Procfile is for Heruku.
requirements.txt: Library names required for running telegram bot program.
pi_code: Contains the code to be run on the Raspberry Pi Zero W.
dataRetriver.py: Python program that gets values from sensor and stores the data in log file and firebase.
lcdDisplay.py: Python program to display data on LCD screen.
requirements.txt: Required libraries for the python programs in this folder.
RPi_I2C_driver.py: LCD display driver.
sampleENV: A sample env that lists all the env variables used by the programs.
Components Used
Arduino Nano - Tomson Electronics
Arduino UNO and programming cable - Tomson Electronics
MQ-2 gas sensor - Tomson Electronics
MQ-4 - Amazon
MQ-5 - Tomson Electronics
MQ-135 - Tomson Electronics
Rain sensor - Tomson Electronics
DHT22 - Tomson Electronics
Raspberry Pi Zero W - Tomson Electronics
LCD module - Tomson Electronics
LCD I2C module - Tomson Electronics
SD card class 10 16 GB - Amazon
220V to 12V DC Adapter - Amazon
Buck converter - Tomson Electronics
Male headers - Tomson Electronics
Female headers - Tomson Electronics
Dotted PCB - Robo Elements
Ribbon cable - Amazon
USB extension cable - Amazon
Powered USB hub - Amazon
Pole, Umbrella, wires, tapes and zip ties
Pre-Installation Steps
Connect the sensors as per circuit diagram.
Flash the nano and uno code respectively.
Clone the project in Raspberry Pi Zero W.
Rename sampleENV as .env.
Create Realtime database in firebase.
add the necessary edits to .env file
Run the python programs inside pi_code folder.
create a python inside heroku_code/telegram-bot with name credentials.py with following contents:-
bot_token = "Bot Token"
bot_user_name = "Bot Username"
URL = "Heroku app URL"
firebase_token = "Firebase token"
firebase_authDomain = "Firebase authDomain"
firebase_databaseURL = "Firebase database URL"
firebase_storageBucket = "Firebase Storage Bucket"
Images
Database
arduino mega code
#include "ArduinoJson.h"
// Software Serial
#include <SoftwareSerial.h> // Library for Software Serial
SoftwareSerial s_serial_to_esp(3, 2); //RX, TX
// DHT22
#include "DHT.h"
#define DHTPIN 5
#define DHTTYPE DHT22
DHT dht(DHTPIN, DHTTYPE);
// BMP280
#include <Wire.h>
#include <SPI.h>
#include <Adafruit_BMP280.h>
Adafruit_BMP280 bmp;
// Rain Sensor
#define RAINSENSORANALOGPIN A7
#define RAINSENSORDIGITALPIN 22
// Rain Guage
#define RAINGUAGEPIN 7
int rain_guage_counter = 0;
int rain_guage_flag = 0;
int rain_guage_data = 0;
// Wind Speed
#define WINDSPEEDPIN 23
// Wind Direction
#define WINDDIRN 25
#define WINDDIRNE 27
#define WINDDIRE 37
#define WINDDIRSE 39
#define WINDDIRS 41
#define WINDDIRSW 43
#define WINDDIRW 45
#define WINDDIRNW 47
// RTC Module
#include <RTClib.h>
RTC_DS1307 rtc;
// Micro SD card Module
#include <SPI.h>
#include <SD.h>
File sd_card;
int sdcard_chip_select = 53;
// LEDs
#define SDREADLEDPIN 29
#define BMPERRLEDPIN 33
#define RTCERRLEDPIN 31
#define SDCRDERRLEDPIN 35
// Global counter
int counter = 0;
bool sdcard_flag = false;
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
Serial.println("-------------------------");
Serial.println(" Weather Station Cluster ");
Serial.println("-------------------------");
Serial.println("Sensor Initialisation \n");
// Initialise LEDs
pinMode(SDREADLEDPIN, OUTPUT);
pinMode(BMPERRLEDPIN, OUTPUT);
pinMode(RTCERRLEDPIN, OUTPUT);
pinMode(SDCRDERRLEDPIN, OUTPUT);
// Initialise LED to off
digitalWrite(SDREADLEDPIN, LOW);
digitalWrite(BMPERRLEDPIN, LOW);
digitalWrite(RTCERRLEDPIN, LOW);
digitalWrite(SDCRDERRLEDPIN, LOW);
// Initialise DHT22
Serial.print("1. Initialising DHT22");
dht.begin();
Serial.println("\t Success");
// Initialise BMP280
Serial.print("2. Initialising BMP280");
int bmp_lib = bmp.begin();
if(!bmp_lib){
digitalWrite(BMPERRLEDPIN, HIGH);
Serial.println("\t Failed");
Serial.flush();
abort();
}
else {
Serial.println("\t Success");
}
// Initialise RTC module
Serial.print("3. Initialising RTC module");
if (! rtc.begin()) {
digitalWrite(RTCERRLEDPIN, HIGH);
Serial.println("\t Failed");
Serial.flush();
abort();
}
else {
Serial.println("\t Success");
}
Serial.print("4. Initialising Micro SD card module");
pinMode(sdcard_chip_select, OUTPUT);
if (!SD.begin()) {
digitalWrite(SDCRDERRLEDPIN, HIGH);
Serial.println("\t Failed");
while (1);
}
else {
Serial.println("\t Success");
}
Serial.print("5. Initialising Rain sensor");
// Initialise rain sensor
pinMode(INPUT, RAINSENSORANALOGPIN);
pinMode(INPUT, RAINSENSORDIGITALPIN);
Serial.println("\t Success");
Serial.print("6. Initialising Rain guage");
// Initialise rain guage
pinMode(INPUT, RAINGUAGEPIN);
Serial.println("\t Success");
// Initialise Wind speed
Serial.print("7. Initialising Wind speed sensor");
pinMode(INPUT, WINDSPEEDPIN);
Serial.println("\t Success");
// Initialise Wind direction
Serial.print("8. Initialising Wind direction sensor");
pinMode(INPUT, WINDDIRN);
pinMode(INPUT, WINDDIRNE);
pinMode(INPUT, WINDDIRE);
pinMode(INPUT, WINDDIRSE);
pinMode(INPUT, WINDDIRS);
pinMode(INPUT, WINDDIRSW);
pinMode(INPUT, WINDDIRW);
pinMode(INPUT, WINDDIRNW);
Serial.println("\t Success");
// Begin software Serial
Serial.print("9. Initialising software serial");
s_serial_to_esp.begin(4800);
Serial.println("\t Success");
Serial.println("\ninitialiaation complete");
Serial.println("-------------------------\n");
Serial.println("Post Initialisation steps");
Serial.println("-------------------------\n");
// BMP post initialisation
Serial.println("Setting BMP settings");
bmp.setSampling(Adafruit_BMP280::MODE_NORMAL, /* Operating Mode. */
Adafruit_BMP280::SAMPLING_X2, /* Temp. oversampling */
Adafruit_BMP280::SAMPLING_X16, /* Pressure oversampling */
Adafruit_BMP280::FILTER_X16, /* Filtering. */
Adafruit_BMP280::STANDBY_MS_500); /* Standby time. */
// RTC module
if (! rtc.isrunning()) {
Serial.println("RTC is NOT running, let's set the time!");
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
}
}
void loop() {
// put your main code here, to run repeatedly:
// Read data from DHT22
float dht_humidity = dht.readHumidity();
float dht_temperature = dht.readTemperature();
float dht_heat_index = dht.computeHeatIndex(dht_temperature, dht_humidity, false);
// Read data from BMP280
double bmp_temperature = bmp.readTemperature();
/* bmp_pressure = pressure_value / 100 + const
* const = 1018.33 - (get pressure value of your location from https://en.allmetsat.com/metar-taf/)
* Thiruvananthapuram, Kerala, India - https://en.allmetsat.com/metar-taf/india-sri-lanka.php?icao=VOTV
*/
double bmp_pressure = bmp.readPressure() / 100.00 + (1018.33 - 1012);
double bmp_altitude = bmp.readAltitude(bmp_pressure);
// Read data from RTC module
DateTime now = rtc.now();
String rtc_day = (now.day()>9)?String(now.day()):"0" + String(now.day());
String rtc_month = (now.month()>9)?String(now.month()):"0" + String(now.month());
String rtc_year = String(now.year());
String rtc_hour = (now.hour()>9)?String(now.hour()):"0" + String(now.hour());
String rtc_minutes = (now.minute()>9)?String(now.minute()):"0" +String(now.minute());
String rtc_seconds = (now.second()>9)?String(now.second()):"0" +String(now.second());
// Read data from Rain Sensor
int rain_sensor_data_analog = analogRead(RAINSENSORANALOGPIN);
int rain_sensor_data_digital = digitalRead(RAINSENSORDIGITALPIN);
int rain_guage_output = digitalRead(RAINGUAGEPIN);
if(rain_guage_flag != rain_guage_output) {
rain_guage_data ++;
rain_guage_flag = rain_guage_output;
}
// Reset rain guage every midnight
if (now.hour() == 0 && now.minute() == 00) {
rain_guage_data = 0;
}
// Get wind direction
int wind_direction_n = digitalRead(WINDDIRN);
int wind_direction_ne = digitalRead(WINDDIRNE);
int wind_direction_e = digitalRead(WINDDIRE);
int wind_direction_se = digitalRead(WINDDIRSE);
int wind_direction_s = digitalRead(WINDDIRS);
int wind_direction_sw = digitalRead(WINDDIRSW);
int wind_direction_w = digitalRead(WINDDIRW);
int wind_direction_nw = digitalRead(WINDDIRNW);
String wind_direction_data;
if (wind_direction_n == HIGH) {
wind_direction_data = "North";
}
else if (wind_direction_ne == HIGH) {
wind_direction_data = "North EAST";
}
else if (wind_direction_e == HIGH) {
wind_direction_data = "EAST";
}
else if (wind_direction_se == HIGH) {
wind_direction_data = "South East";
}
else if (wind_direction_s == HIGH) {
wind_direction_data = "South";
}
else if (wind_direction_sw == HIGH) {
wind_direction_data = "South West";
}
else if (wind_direction_w == HIGH) {
wind_direction_data = "West";
}
else if (wind_direction_nw == HIGH) {
wind_direction_data = "North West";
}
// Create JSON data
DynamicJsonDocument doc(1024);
doc["rtc_day"] = rtc_day;
doc["rtc_month"] = rtc_month;
doc["rtc_year"] = rtc_year;
doc["rtc_hour"] = rtc_hour;
doc["rtc_minutes"] = rtc_minutes;
doc["rtc_seconds"] = rtc_seconds;
doc["dht_humidity"] = dht_humidity;
doc["dht_temperature"] = dht_temperature;
doc["dht_heat_index"] = dht_heat_index;
doc["bmp_temperature"] = bmp_temperature;
doc["bmp_pressure"] = bmp_pressure;
doc["bmp_altitude"] = bmp_altitude;
doc["rain_sensor_data_analog"] = rain_sensor_data_analog;
doc["rain_sensor_data_digital"] = rain_sensor_data_digital;
doc["rain_guage_data"] = rain_guage_data;
//doc["wind_speed"] =
doc["wind_direction"] = wind_direction_data;
// Send JSON via Serial
serializeJson(doc, s_serial_to_esp);
doc.clear();
// Store data in micro sdcard every 5 seconds
if (counter % 30 == 0) {
String final_string = rtc_day + "-" + rtc_month + "-" + rtc_year + "," +
rtc_hour + ":" + rtc_minutes + ":" + rtc_seconds + "," +
dht_humidity + "," + dht_temperature + "," + dht_heat_index + "," +
bmp_temperature + "," + bmp_pressure + "," + bmp_altitude + "," +
rain_sensor_data_analog + "," + rain_sensor_data_digital + "," +
rain_guage_data;
sd_card = SD.open("sdt.txt", FILE_WRITE);
sd_card.println(final_string);
sd_card.close();
counter = 0;
sdcard_flag = true;
}
counter ++;
if (sdcard_flag == true) {
if (counter == 1) {
digitalWrite(SDREADLEDPIN, HIGH);
}
else if (counter == 2) {
digitalWrite(SDREADLEDPIN, LOW);
sdcard_flag = false;
}
}
// Serial print for debugging
Serial.println("-----------------------------------");
Serial.print("1. RTC Date: "); Serial.print(rtc_day);Serial.print("-");Serial.print(rtc_month);Serial.print("-");Serial.println(rtc_year);
Serial.print("2. RTC Time: "); Serial.print(rtc_hour);Serial.print(":");Serial.print(rtc_minutes);Serial.print(":");Serial.println(rtc_seconds);
Serial.print("3. DHT22 Humidity: "); Serial.print(dht_humidity); Serial.println(" %");
Serial.print("4. DHT22 Temperature: "); Serial.print(dht_temperature); Serial.println(" °C");
Serial.print("5. DHT22 Heat Index: "); Serial.print(dht_heat_index); Serial.println(" °C");
Serial.print("6. BMP280 Temperature: "); Serial.print(bmp_temperature); Serial.println(" °C");
Serial.print("7. BMP280 Pressure: "); Serial.print(bmp_pressure); Serial.println(" mbar");
Serial.print("8. BMP Altitude: "); Serial.print(bmp_altitude); Serial.println(" m");
Serial.print("9. Rain Sensor (Analog): "); Serial.println(rain_sensor_data_analog);
Serial.print("10. Rain Sensor (Digital): "); Serial.println(rain_sensor_data_digital);
Serial.print("11. Rain Guage: "); Serial.println(rain_guage_data);
Serial.print("12. Wind Speed: "); Serial.print(""); Serial.println(" m/s");
Serial.print("13. Wind direction: "); Serial.println();
Serial.println("-----------------------------------\n");
delay(1000);
}
nodemcu code
#include <WiFiManager.h>
WiFiManager wifiManager;
// For OTA updates
#include <ArduinoOTA.h>
// Micro SD card Module
#include <SPI.h>
#include <SD.h>
File sd_card;
#define CS_PIN 15
// JSON connection
#include "ArduinoJson.h"
// Software Serial
#include <SoftwareSerial.h> // Library for Software Serial
SoftwareSerial s_serial_to_mega(2,0); //RX, TX
// LED - D4 - GPIO2
#define SDCRDERRLEDPIN 2
int counter = 0;
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
Serial.println("-------------------------");
Serial.println(" Weather Station Cluster ");
Serial.println("-------------------------");
Serial.println("Sensor Initialisation \n");
// Initialise LED
pinMode(SDCRDERRLEDPIN, OUTPUT);
// Initialise LED to off
digitalWrite(SDCRDERRLEDPIN, LOW);
Serial.print("1. Initialising SD card module");
if (!SD.begin(CS_PIN)) {
digitalWrite(SDCRDERRLEDPIN, HIGH);
Serial.println("\t Failed");
while (1);
}
else {
Serial.println("\t Success");
}
Serial.print("2. OTA updater initialised");
wifiManager.autoConnect("Weather Station Cluster");
ArduinoOTA.setPort(8266);
ArduinoOTA.setHostname("Weather Station Cluster");
ArduinoOTA.onStart([]() {
String type;
if (ArduinoOTA.getCommand() == U_FLASH) {
type = "sketch";
} else { // U_FS
type = "filesystem";
}
// NOTE: if updating FS this would be the place to unmount FS using FS.end()
Serial.println("Start updating " + type);
});
ArduinoOTA.onEnd([]() {
Serial.println("\nEnd");
});
ArduinoOTA.onProgress([](unsigned int progress, unsigned int total) {
Serial.printf("Progress: %u%%\r", (progress / (total / 100)));
});
ArduinoOTA.onError([](ota_error_t error) {
Serial.printf("Error[%u]: ", error);
if (error == OTA_AUTH_ERROR) {
Serial.println("Auth Failed");
} else if (error == OTA_BEGIN_ERROR) {
Serial.println("Begin Failed");
} else if (error == OTA_CONNECT_ERROR) {
Serial.println("Connect Failed");
} else if (error == OTA_RECEIVE_ERROR) {
Serial.println("Receive Failed");
} else if (error == OTA_END_ERROR) {
Serial.println("End Failed");
}
});
ArduinoOTA.begin();
Serial.println("\t Success");
s_serial_to_mega.begin(4800);
Serial.println("Initialisation complete");
Serial.println("--------------------------------");
Serial.println("Fetch data from SDcard");
if (SD.exists("host.txt")) {
Serial.println("host.txt folder already exists.");
}
else {
Serial.println("host.txt folder doesn't exist");
Serial.flush();
abort();
}
}
void loop() {
// put your main code here, to run repeatedly:
if (s_serial_to_mega.available()) {
DynamicJsonDocument doc(1024);
DeserializationError err = deserializeJson(doc, s_serial_to_mega);
if (err == DeserializationError::Ok) {
float dht_humidity = doc["dht_humidity"];
float dht_temperature = doc["dht_temperature"];
float dht_heat_index = doc["dht_heat_index"];
double bmp_temperature = doc["bmp_temperature"];
double bmp_pressure = doc["bmp_pressure"];
double bmp_altitude = doc["bmp_altitude"];
String rtc_day = doc["rtc_day"];
String rtc_month = doc["rtc_month"];
String rtc_year = doc["rtc_year"];
String rtc_hour = doc["rtc_hour"];
String rtc_minutes = doc["rtc_minutes"];
String rtc_seconds = doc["rtc_seconds"];
int rain_sensor_data_analog = doc["rain_sensor_data_analog"];
int rain_sensor_data_digital = doc["rain_sensor_data_digital"];
int rain_guage_data = doc["rain_guage_data"];
if (WiFi.status() == WL_CONNECTED) {
while(WiFi.status() == WL_CONNECTED){
ArduinoOTA.handle();
}
}
Serial.println("--------------------------------------------");
Serial.print("1. Humidity (DHT22): ");Serial.println(dht_humidity);
Serial.print("2. Temperature (DHT22): ");Serial.println(dht_temperature);
Serial.print("3. Heat Index (DHT22): ");Serial.println(dht_heat_index);
Serial.print("4. Temperature (BMP280): ");Serial.println(bmp_temperature);
Serial.print("5. Pressure (BMP280): ");Serial.println(bmp_pressure);
Serial.print("6. Altitude (BMP280): ");Serial.println(bmp_altitude);
Serial.print("7. Day (RTC): ");Serial.println(rtc_day);
Serial.print("8. Month (RTC): ");Serial.println(rtc_month);
Serial.print("9. Year (RTC): ");Serial.println(rtc_year);
Serial.print("10. Hour (RTC): ");Serial.println(rtc_hour);
Serial.print("11. Minutes (RTC): ");Serial.println(rtc_minutes);
Serial.print("12. Seconds (RTC): ");Serial.println(rtc_seconds);
Serial.print("13. Rain Sensor (Analog): ");Serial.println(rain_sensor_data_analog);
Serial.print("14. Rain Sesnor (Digital): ");Serial.println(rain_sensor_data_digital);
Serial.print("15. Rain Guage: ");Serial.println(rain_guage_data);
if(counter%30 == 0) {
Serial.println("Code to send data");
}
counter++;
}
else {
// Print error to the "debug" serial port
Serial.print("deserializeJson() returned ");
Serial.println(err.c_str());
// Flush all bytes in the "link" serial port buffer
while (s_serial_to_mega.available() > 0)
s_serial_to_mega.read();
}
}
}
Old Protoype
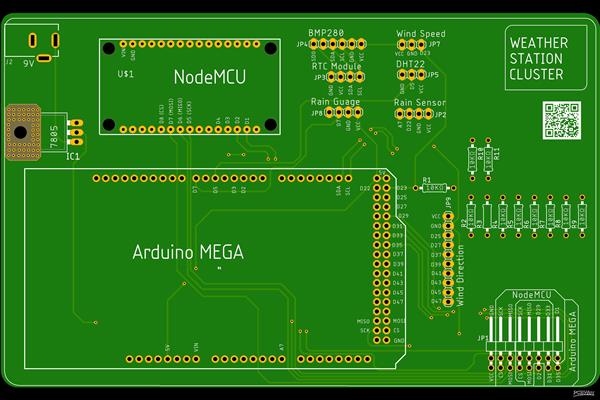
Home Weather Station
*PCBWay community is a sharing platform. We are not responsible for any design issues and parameter issues (board thickness, surface finish, etc.) you choose.
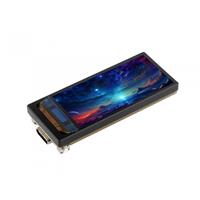
ESP32-S3 1.91inch AMOLED Display Development Board, 240×536, 32-bit LX7 Dual-core Processor, Up to 240MHz Frequency, Accelerometer And Gyroscope Sensor, ESP32 With Display
BUY NOW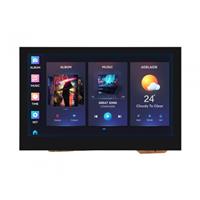
ESP32-S3 4.3inch Capacitive Touch Display Development Board, 800×480, 5-point Touch, 32-bit LX7 Dual-core Processor
BUY NOW- Comments(2)
- Likes(1)
-
Zhuxuan Ma Apr 05,2022
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by Sreeram.zeno
-
Esp12-F Cluster V1.0 The ESP8266 is a low-cost Wi-Fi microchip, with built-in TCP/IP networking software, and microcontro...
-
TB6612FNG Motor Driver The TB6612FNG Motor Driver can control up to two DC motors at a constant current of 1.2A (3.2A peak)...
-
Sunny Buddy Solar Charger v1.0 This is the Sunny Buddy, a maximum power point tracking (MPPT) solar charger for single-cell LiPo ba...
-
Diy 74HC4051 8 Channel Mux Breakout Pcb The 74HC4051; 74HCT4051 is a single-pole octal-throw analog switch (SP8T) suitable for use in analog...
-
Diy RFM97CW Breakout Pcb IntroductionLoRa? (standing for Long Range) is a LPWAN technology, characterized by a long range ass...
-
ProMicro-RP2040 Pcb The RP2040 is a 32-bit dual ARM Cortex-M0+ microcontroller integrated circuit by Raspberry Pi Founda...
-
Serial Basic CH340G Pcb A USB adapter is a type of protocol converter that is used for converting USB data signals to and fr...
-
Mp3 Shield For Arduino Hardware OverviewThe centerpiece of the MP3 Player Shield is a VS1053B Audio Codec IC. The VS1053B i...
-
MRK CAN Shield Arduino The CAN-BUS Shield provides your Arduino or Redboard with CAN-BUS capabilities and allows you to hac...
-
AVR ISP Programmer AVR is a family of microcontrollers developed since 1996 by Atmel, acquired by Microchip Technology ...
-
Diy Arduino mega Pcb The Arduino Mega 2560 is a microcontroller board based on the ATmega2560. It has 54 digital input/ou...
-
Max3232 Breakout Board MAX3232 IC is extensively used for serial communication in between Microcontroller and a computer fo...
-
Line Follower Pcb The Line Follower Array is a long board consisting of eight IR sensors that have been configured to ...
-
HMC6343 Accelerometer Module The HMC6343 is a solid-state compass module with tilt compensation from Honeywell. The HMC6343 has t...
-
RTK2 GPS Module For Arduino USBThe USB C connector makes it easy to connect the ZED-F9P to u-center for configuration and quick ...
-
Arduino Explora Pcb The Arduino Esplora is a microcontroller board derived from the Arduino Leonardo. The Esplora differ...
-
Diy Stepper Motor Easy Driver A motor controller is a device or group of devices that can coordinate in a predetermined manner the...
-
Diy Arduino Pro Mini The Arduino Pro Mini is a microcontroller board based on the ATmega168 . It has 14 digital input/out...
-
-
-
-
-
-
Dvorak 2024 and 'Ye Olde Qwerty' Human-Factored Compact Two-Hand Keyboards
76 0 0