![]() |
arduino IDEArduino
|
HMC6343 Accelerometer Module
The HMC6343 is a solid-state compass module with tilt compensation from Honeywell. The HMC6343 has three axis of magneto, three axis of accelerometer, and a PIC core running all the calculations. What you get is a compass heading over I2C that stays the same even as you tilt the board. Solid-state (and many classic water based) compasses fail badly when not held flat. The tilt compensated HMC6343 is crucial for those real-world compass applications
As part of my PhD work I have produced a modified version of the Second Life viewer, which I have dubbed Pangolin after the Open Virtual Worlds research group’s previous Mongoose, Armadillo & Chimera projects, that allows;
connecting a serial device to the viewer
controlling movement of the avatar according to GPS readings
controlling the camera according to accelerometer & magnetometer readings
The combination of these features allows you to do things like connect an accelerometer, magnetometer & GPS receiver to an Arduino, have it dump readings into the viewer & have the viewer use them to control the avatar & camera. The motivation behind this was to address the ‘vacancy problem’ by creating a mobile cross reality interface; allowing a user to experience simultaneous presence in a real environment & an equivalent synthetic environment, using their physical position & orientation as an implicit method of control for their synthetic representation. I’m presenting a paper about this at the iED 2013 Boston summit in June – if I can secure funding to actually get me there!
Getting Code & Building
The Pangolin source code is available on Bitbucket, with my additions & modifications licensed under the GNU General Public License. The serial IO functionality uses Terraneo Federico’s AsyncSerial class which is licensed under the Boost Software License. The viewer codebase was forked from Linden Lab’s viewer-release before the removal of the --loginuri flag so Pangolin is compatible with OpenSim grids/servers.
Instructions for building the viewer are available on the Second Life wiki. I build using 32-bit Debian GNU/Linux (specifically by chrooting into a 32-bit debootstrap install from a 64-bit Arch Linux host, see my instructions) which produces a binary that runs on 32-bit Linux & on 64-bit Linux with 32-bit compatibility libraries installed.
The serial connectivity makes use of Boost.Asio. The Linden-provided Boost pre-built library is missing some of the features that my modifications make use of, so I build with LightDrake’s alternative; his public libraries are available here. To use these libraries, edit the corresponding entry in the autobuild.xml file in the root of the codebase. You’re looking for this section, here I’ve commented out the original library & hash for the Linux version & replaced it with LightDrake’s;
Linux 1.45.0 version; if you try the more recent Linux version or the Windows/Darwin versions let me know how it goes! Using this new library leads to a rather nasty namespace collision (at least with the Linux 1.45.0 version) for which I have uploaded a fix.
I’ve also put an example Arduino sketch on Bitbucket, which uses an HMC6343 accelerometer/magnetometer & a u-blox MAX-6 GPS receiver, which I wrote about previously.
Binary
I’ve uploaded a 32-bit Linux binary to Bitbucket if you just want to try it out without the rigmarole of successfully setting up the (rather particular) build environment.
Usage
Start the viewer & login as normal, then take a look at the Serial menu which contains a single entry Serial Monitor. Click this & you will see something like this;
Put the path to the serial device & the baudrate into the fields in the Device settings section at the top & click [Connect]. For me using an Arduino on Linux boxes the serial device normally appears at /dev/ttyACM0 or /dev/ttyS0 if it’s the first serial device, /dev/ttyACM1 or /dev/ttyS1 if it’s the second serial device, etc. If you’re using an Arduino & are having trouble finding it, just start the Arduino IDE & look at the Serial Port entry in the Tools menu.
Pangolin expects messages, separated by newline characters, in the following format;
1
<bearing> <pitch> <roll> <latitude> <longitude>
For example;
1
183.90 75.80 -59.30 56.339991 -2.7875334
So make sure that your serial device adheres to this message format; the example Arduino sketch linked above does this & might be a useful starting point for you.
The fields in the Anchor settings section are for entering the location of a single point for which you know both the real world latitude/longitude & the corresponding virtual world coordinates along with the scale of the virtual world to the real world – eg if 1m in the real world is represented by 1.2m in the virtual world then enter 1.2 into the Scale field.
Sim X & Sim Y of the anchor point are global; this is necessary to allow Pangolin to work across multiple regions (such as mega regions). Because regions are 256x256m you can easily calculate the global coordinate of a point by doing (256 * region position) + local coordinate. For example, if my anchor point is at 127,203,23 in a region at 1020,1043, then the global X coordinate of the anchor point is (1020 * 256) + 127 = 261247 & the global Y coordinate of the anchor point is (1043 * 256) + 203 = 267211. Height isn’t implemented (vertical accuracy of GPS is substantially worse than horizontal) so just pick a Sim Z of around your sim’s ground level. The latitude & longitude fields have 6 decimal places of accuracy.
Once you have input all of the anchor settings, click [Set] & if everything is okay you should see the data from the serial device in the Received data section & the processed position values in the Calculated data section. The Pause checkbox will stop the fields from updating so you can copy the values, etc. If you get garbled received data then you have probably set the baudrate incorrectly – Pangolin will ignore this data instead of trying to process it & sending your avatar’s movement haywire.
To enable/disable control of the camera & your avatar’s movement from the received/calculated data use the Orientation control & Position control checkboxes in the Controls section. The various spinners in this section allow you to alter the smoothing, high pass filters & frequency of updates. Good values for these will depend upon what hardware/sensors you are using, the scale of your sim, etc.
#include <Wire.h>
#define HMC6343_ADDRESS 0x19
#define HMC6343_HEADING_REG 0x50
// data structure as defined by the joystick firmeware
struct {
int8_t x;
int8_t y;
uint8_t buttons;
uint8_t rfu;
} joyReport;
void setup() {
Wire.begin(); // initialize the I2C bus
Serial.begin(115200); // initialize the serial bus
}
void loop() {
byte highByte, lowByte;
Wire.beginTransmission(HMC6343_ADDRESS); // start communication with HMC6343
Wire.write(0x74); // set HMC6343 orientation
Wire.write(HMC6343_HEADING_REG); // send the address of the register to read
Wire.endTransmission();
Wire.requestFrom(HMC6343_ADDRESS, 6); // request six bytes of data from the HMC6343
while(Wire.available() < 1); // busy wait while there is no byte to receive
highByte = Wire.read();
lowByte = Wire.read();
float heading = ((highByte << 8) + lowByte) / 10.0; // heading in degrees
highByte = Wire.read();
lowByte = Wire.read();
float pitch = ((highByte << 8) + lowByte) / 10.0; // pitch in degrees
highByte = Wire.read();
lowByte = Wire.read();
float roll = ((highByte << 8) + lowByte) / 10.0; // roll in degrees
joyReport.buttons = 0;
joyReport.rfu = 0;
joyReport.x = constrain(((int)(map(roll, -90, 90, -100, 100))), -100, 100);
joyReport.y = constrain(((int)(map(pitch, -90, 90, -100, 100))), -100, 100);
Serial.write((uint8_t *)&joyReport, 4);
delay(100); // do this at approx 10Hz
}
Does it work?
The code itself works nicely. I tested it walking around the ruins of the cathedral at St Andrews, for which the Open Virtual Worlds group has produced an OpenSim reconstruction, using a MSI WindPad 110W tablet computer. This sim is accessible on our own grid & on OSgrid (search for regions called ‘StAndrewsOVW’) though the OSgrid version is usually older than our local grid.
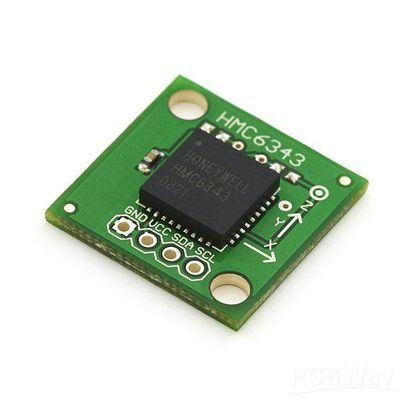
HMC6343 Accelerometer Module
*PCBWay community is a sharing platform. We are not responsible for any design issues and parameter issues (board thickness, surface finish, etc.) you choose.
- Comments(0)
- Likes(0)
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by Sreeram.zeno
-
Esp12-F Cluster V1.0 The ESP8266 is a low-cost Wi-Fi microchip, with built-in TCP/IP networking software, and microcontro...
-
TB6612FNG Motor Driver The TB6612FNG Motor Driver can control up to two DC motors at a constant current of 1.2A (3.2A peak)...
-
Sunny Buddy Solar Charger v1.0 This is the Sunny Buddy, a maximum power point tracking (MPPT) solar charger for single-cell LiPo ba...
-
Diy 74HC4051 8 Channel Mux Breakout Pcb The 74HC4051; 74HCT4051 is a single-pole octal-throw analog switch (SP8T) suitable for use in analog...
-
Diy RFM97CW Breakout Pcb IntroductionLoRa? (standing for Long Range) is a LPWAN technology, characterized by a long range ass...
-
ProMicro-RP2040 Pcb The RP2040 is a 32-bit dual ARM Cortex-M0+ microcontroller integrated circuit by Raspberry Pi Founda...
-
Serial Basic CH340G Pcb A USB adapter is a type of protocol converter that is used for converting USB data signals to and fr...
-
Mp3 Shield For Arduino Hardware OverviewThe centerpiece of the MP3 Player Shield is a VS1053B Audio Codec IC. The VS1053B i...
-
MRK CAN Shield Arduino The CAN-BUS Shield provides your Arduino or Redboard with CAN-BUS capabilities and allows you to hac...
-
AVR ISP Programmer AVR is a family of microcontrollers developed since 1996 by Atmel, acquired by Microchip Technology ...
-
Diy Arduino mega Pcb The Arduino Mega 2560 is a microcontroller board based on the ATmega2560. It has 54 digital input/ou...
-
Max3232 Breakout Board MAX3232 IC is extensively used for serial communication in between Microcontroller and a computer fo...
-
Line Follower Pcb The Line Follower Array is a long board consisting of eight IR sensors that have been configured to ...
-
HMC6343 Accelerometer Module The HMC6343 is a solid-state compass module with tilt compensation from Honeywell. The HMC6343 has t...
-
RTK2 GPS Module For Arduino USBThe USB C connector makes it easy to connect the ZED-F9P to u-center for configuration and quick ...
-
Arduino Explora Pcb The Arduino Esplora is a microcontroller board derived from the Arduino Leonardo. The Esplora differ...
-
Diy Stepper Motor Easy Driver A motor controller is a device or group of devices that can coordinate in a predetermined manner the...
-
Diy Arduino Pro Mini The Arduino Pro Mini is a microcontroller board based on the ATmega168 . It has 14 digital input/out...
-
-
-
-
-
-
3D printed Enclosure Backplate for Riden RD60xx power supplies
153 1 1 -
-