![]() |
arduino IDEArduino
|
Line Follower Pcb
The Line Follower Array is a long board consisting of eight IR sensors that have been configured to read as digital bits! We have designed the Line Follower Arrays to follow a dark line of about ? inch width or smaller (spray paint or electrical tape) on a light background. Each array features visible LEDs that point upward when the board is attached (properly) so you can see what the robot sees, brightness control right on the board, and an I2C interface for reading and power control. Here at , the RedBot Shadow Chassis was used as a test platform but really this was designed as an add-on for almost any bot.
The line follower functions by taking an 8-bit reading of reflectance for use with following lines or reading dark/light patterns and can see from about ? to ? inches away. The IR brightness control and indicator can be adjusted with the on-board potentiometer and is capable of showing you the strength of the IR LEDs. Illumination can be turned on and off with software to conserve power, or left on all the time for faster readings. The Line Follower Array requires 5V of power with a supply current range of 25-185mA with strobing disabled and 16-160mA with it enabled. Additionally we have added six mounting holes to the line follower with the two inner holes designed to fit our Shadow Chassis while the other four are general purpose.
Features:
8 sensor eyes (QRE1113, like in our line sensor breakout)
I2C interface
Adjust IR brightness on the fly with a knob
Switch IR on and off with software
Switch visual indicators on and off with software
Invert dark/light sight with software
Based on the SX1509 I/O expander
As explained before the op-amps are needed to convert the IR sensor outputs to TTL logic.
The op-amp I've used is LM358 as its cheap and easily available.The chip contains 2 op-amps so 3 chips are needed for 5 led sensors(2 per chip).
The sensor input goes to the non-inverting terminal(pins 3 and 5).
Pin 1 and 7 of the op-amp are the output pins and are connected to the arduino digital pins.Having indicator LEDs at the output is optional....though it adds a nice effect and helps in debugging :p
Pin 4 goes to ground and 8 to 5V.
Normally a 10k pot is used as an input for the inverting terminal so that the sensitivity of the senor can be changed.However,I've used two 4.7kΩ resistors instead since I tested it and got a decent range.
For the op-amp connections have a look at the ckt diagram provided...the conncetion for each op-amp is identical.
The male header pins are where the output from the IR sensor array is connected and the female header are where the output of the op-amp board is connected to the arduino.
Note : Since my goal is to detect a black line on a white surface I've made the connections accordingly...if you want to detect a white line on a black surface just switch the connections for the inverting and non-inverting pins.
So, we are going to use this property of light to detect the line. To detect light, either LDR (light-dependent resistor) or an IR sensor can be used. For this project, we are going with the IR sensor because of its higher accuracy. To detect the line, we place two IR sensors one on the left and other on the right side of the robot as marked in the diagram below. We then place the robot on the line such that the line lies in the middle of both sensors. We have covered a detailed Arduino IR sensor tutorial which you can check to learn more about the working of IR sensors with Arduino Uno.
Infrared sensors consist of two elements, a transmitter and a receiver. The transmitter is basically an IR LED, which produces the signal and the IR receiver is a photodiode, which senses the signal produced by the transmitter. The IR sensors emits the infrared light on an object, the light hitting the black part gets absorbed thus giving a low output but the light hitting the white part reflects back to the transmitter which is then detected by the infrared receiver, thereby giving an analog output. Using the stated principle, we control the movement of the robot by driving the wheels attached to the motors, the motors are controlled by a microcontroller.
How does a Line Follower Robot Navigates?
A typical line follower robot has two sets of motors, let's call them left motor and right motor. Both motors rotate on the basis of the signal received from the left and the right sensors respectively. The robot needs to perform 4 sets of motion which includes moving forward, turning left, turning right and coming to a halt. The description about the cases are given below.
An infrared sensor emits the light to detect some surroundings. In the infrared spectrum, all the objects radiate some form of thermal radiation that is invisible to our eyes, but an IR sensor can detect these radiations.
Here, IR LED is an emitter, and the IR photodiode is a detector. An IR LED emits the IR light, and the photodiode is sensitive to this IR light. When IR light falls on the photodiode, the output voltages and the resistances will change in proportion to the magnitude of the received IR light.
code
const int rme=10,lme=11; //enable pins
const int rmb=8, rmf=9, lmb=12, lmf=13; //motor signals
const int ir0=2, ir1=3, ir2=4, ir3=5, ir4=6; //ir signals white=1 and black=0
int val0=0, val1=0, val2=0, val3=0, val4=0; //variables void
InALine(bool x) //to move in a line
{ if(x==true)
{ analogWrite(rme,60);
analogWrite(lme,60);
digitalWrite(rmb,LOW);
digitalWrite(rmf,HIGH);
digitalWrite(lmb,LOW);
digitalWrite(lmf,HIGH);
Serial.println("forward"); }
else //move backward
{ analogWrite(rme,50);
analogWrite(lme,50);
digitalWrite(rmb,HIGH);
digitalWrite(rmf,LOW);
digitalWrite(lmb,HIGH);
digitalWrite(lmf,LOW);
Serial.println("backward"); } }
void Turn(bool y) //to turn
{ analogWrite(rme,50);
analogWrite(lme,50);
if (y==true) //move right
{ digitalWrite(rmb,LOW);
digitalWrite(rmf,LOW);
digitalWrite(lmb,LOW);
digitalWrite(lmf,HIGH);
Serial.println("right"); }
else //move left
{ digitalWrite(rmb,LOW);
digitalWrite(rmf,HIGH);
digitalWrite(lmb,LOW);
digitalWrite(lmf,LOW);
Serial.println("left"); } }
void stop()
{ analogWrite(rme,0);
analogWrite(lme,0);
Serial.println("stop"); }
void setup()
{ // put your setup code here, to run once:
pinMode(rmb, OUTPUT);
pinMode(rmf, OUTPUT);
pinMode(lmb, OUTPUT);
pinMode(lmf, OUTPUT);
pinMode(rme, OUTPUT);
pinMode(lme, OUTPUT);
pinMode(ir0, INPUT);
pinMode(ir1, INPUT);
pinMode(ir2, INPUT);
pinMode(ir3, INPUT);
pinMode(ir4, INPUT);
Serial.begin(9600); }
void loop()
{ // put your main code here, to run repeatedly:
val0=digitalRead(ir0);
val1=digitalRead(ir1);
val2=digitalRead(ir2);
val3=digitalRead(ir3);
val4=digitalRead(ir4);
if(val0 && val1 && !val2 && val3 && val4) //forward
{ bool x=true; InALine(x); }
else if(val0 && val1 && val2 && !val3 && !val4) //turn right
{ bool y=true; Turn(y); }
else if(!val0 && !val1 && val2 && val3 && val4) //turn left
{ bool y=false; Turn(y); }
else if(!val0 && !val1 && !val2 && !val3 && !val4) //stop
{ stop(); }
else if(val0 && val1 && val2 && val3 && val4) //circle
{ bool y=false; Turn(y); }
else if(!val0 && !val1 && val2 && !val3 && !val4) //in a t-shape condition turn left
{ bool y=false; Turn(y); }
else if(val0 && val1 && !val2 && !val3 && !val4) //in a forward and left condition turn left
{ bool y=false; Turn(y); }
else if(!val0 && !val1 && !val2 && val3 && val4) //in a forward and right condition go straight
{ bool x=true; InALine(x); }
else { bool x=false; InALine(x); }
delay(1000); }
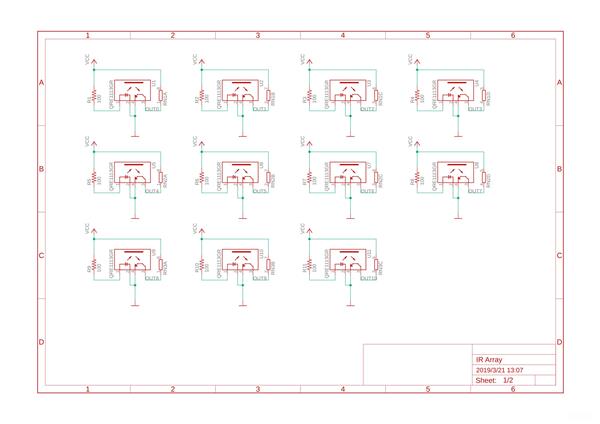
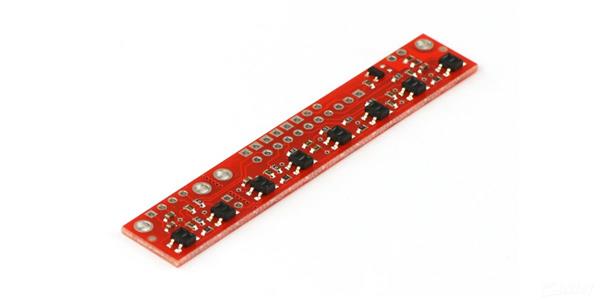
Line Follower Pcb
*PCBWay community is a sharing platform. We are not responsible for any design issues and parameter issues (board thickness, surface finish, etc.) you choose.
- Comments(0)
- Likes(0)
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by Sreeram.zeno
-
Esp12-F Cluster V1.0 The ESP8266 is a low-cost Wi-Fi microchip, with built-in TCP/IP networking software, and microcontro...
-
TB6612FNG Motor Driver The TB6612FNG Motor Driver can control up to two DC motors at a constant current of 1.2A (3.2A peak)...
-
Sunny Buddy Solar Charger v1.0 This is the Sunny Buddy, a maximum power point tracking (MPPT) solar charger for single-cell LiPo ba...
-
Diy 74HC4051 8 Channel Mux Breakout Pcb The 74HC4051; 74HCT4051 is a single-pole octal-throw analog switch (SP8T) suitable for use in analog...
-
Diy RFM97CW Breakout Pcb IntroductionLoRa? (standing for Long Range) is a LPWAN technology, characterized by a long range ass...
-
ProMicro-RP2040 Pcb The RP2040 is a 32-bit dual ARM Cortex-M0+ microcontroller integrated circuit by Raspberry Pi Founda...
-
Serial Basic CH340G Pcb A USB adapter is a type of protocol converter that is used for converting USB data signals to and fr...
-
Mp3 Shield For Arduino Hardware OverviewThe centerpiece of the MP3 Player Shield is a VS1053B Audio Codec IC. The VS1053B i...
-
MRK CAN Shield Arduino The CAN-BUS Shield provides your Arduino or Redboard with CAN-BUS capabilities and allows you to hac...
-
AVR ISP Programmer AVR is a family of microcontrollers developed since 1996 by Atmel, acquired by Microchip Technology ...
-
Diy Arduino mega Pcb The Arduino Mega 2560 is a microcontroller board based on the ATmega2560. It has 54 digital input/ou...
-
Max3232 Breakout Board MAX3232 IC is extensively used for serial communication in between Microcontroller and a computer fo...
-
Line Follower Pcb The Line Follower Array is a long board consisting of eight IR sensors that have been configured to ...
-
HMC6343 Accelerometer Module The HMC6343 is a solid-state compass module with tilt compensation from Honeywell. The HMC6343 has t...
-
RTK2 GPS Module For Arduino USBThe USB C connector makes it easy to connect the ZED-F9P to u-center for configuration and quick ...
-
Arduino Explora Pcb The Arduino Esplora is a microcontroller board derived from the Arduino Leonardo. The Esplora differ...
-
Diy Stepper Motor Easy Driver A motor controller is a device or group of devices that can coordinate in a predetermined manner the...
-
Diy Arduino Pro Mini The Arduino Pro Mini is a microcontroller board based on the ATmega168 . It has 14 digital input/out...
-
-
-
kmMiniSchield MIDI I/O - IN/OUT/THROUGH MIDI extension for kmMidiMini
116 0 0 -
DIY Laser Power Meter with Arduino
165 0 2 -
-
-
Box & Bolt, 3D Printed Cardboard Crafting Tools
156 0 2 -
-
A DIY Soldering Station Perfect for Learning (Floppy Soldering Station 3.0)
569 0 3