|
ArgonParticle.io
|
x 1 | |
|
Micro OLEDSparkfun
|
x 1 |
|
Particle Web IDEParticle.io
|
Coronavirus - India cases tracker
Today everyone is gazing at their phone to keep track of the coronavirus in their country but we tend to forget that it is just increasing internet consumption and worry. So I thought to solve this problem using Particle devices which will use a negligible amount of data to deliver the tracking of coronavirus cases easily. This project will help you to be up to date about the coronavirus India outbreak or can be adapted for your country too. The data gets updated automatically and the device can be used to add more functionality.
The data is collected by the website www.worldometers.info/coronavirus/
Hardware:
I have used Particle Argon and Sparkfun I2C micro-OLED. The particle device comes with a flexible IDE to add lots of integrations or webhooks without hassles.
1 / 3
Particle Argon
Software:
Particle online IDE is used to program the device. Here are the steps for creating integrations for fetching data from websites.
1 / 4 ? I made it for my country India, you can select a different one.
I made it for my country India, you can select a different one.
You need to make a new ThingHTTP. Mine is already done.
After this step, you will get a URL which will also contain your ThingSpeak API key, save that for particle webhook
Circuit Diagram:
The device in action:1 / 4
/**--------------------------------------------------------------------------------------------------------------------------------------* * India COVID-19 live status using custom ThingSpeak HTTP web communication integration * * ---------------------------------------------------------------------------------- * * * * Author: Sumit @ HackElectronics * * Date: April 1, 2020 * * Version: 1.0.0v * * Language: C/C++ * * Contact: sumit.0x0ptimus@yahoo.com * * Difficulty: Begineer/Intermediate * * * * Feel like supporting my work? Let's join to hack the change with Sumit aka @vilaksh. * * * * This program shows how to use thingspeak integration and ThingsHttp requests to fetch data from the below website, * * https://www.worldometers.info/coronavirus/country/india/. Also this shows how to display battery status as a bar on top * * * * Hardware used: * * 1. Particle Argon x1 * * 2. Sparkfun QUIIC MicroOLED * * -------------------------------------------------------------------------------------------------------------------------------------**/ // This #include statement was automatically added by the Particle IDE. #include <SFE_MicroOLED.h> #include "Particle.h" #include <Wire.h> // Include Wire if you're using I2C ////////////////////////// // MicroOLED Definition // ////////////////////////// //The library assumes a reset pin is necessary. The Qwiic OLED has RST hard-wired, so pick an arbitrarty IO pin that is not being used #define PIN_RESET 9 //The DC_JUMPER is the I2C Address Select jumper. Set to 1 if the jumper is open (Default), or set to 0 if it's closed. #define DC_JUMPER 1 ////////////////////////////////// // MicroOLED Object Declaration // ////////////////////////////////// MicroOLED oled(PIN_RESET, DC_JUMPER); // I2C declaration int displayTime(); int covidIndia(); int index1 = 0; int index2 = 0; int batHealth = 0; String data = ""; String values = ""; String deaths = ""; String recovered = ""; String cases = ""; float voltage = analogRead(BATT) * 0.0011224; void setup() { Particle.subscribe("hook-response/IndiaCase", myHandler, MY_DEVICES); //Particle.subscribe("Calls",myCalls); delay(100); Wire.begin(); oled.begin(); // Initialize the OLED oled.clear(ALL); // Clear the display's internal memory oled.display(); // Display what's in the buffer (splashscreen) delay(1000); // Delay 1000 ms oled.clear(PAGE); // Clear the buffer. } void loop() { displayTime(); data = ""; // Trigger the integration Particle.publish("IndiaCase", data, PRIVATE); covidIndia(); //lines(); } int displayTime() { // Reading the voltage and convering into pixels to be rendered on screen voltage = analogRead(BATT) * 0.0011224; voltage = voltage / 3.3; batHealth = (int) (voltage * 63.0); //set zone as per UTC in hours format, also set it in 12 hours format Time.zone(+5.5); oled.clear(PAGE); oled.setFontType(1); oled.setCursor(12,12); oled.print(Time.hourFormat12()); oled.print(":"); oled.print(Time.minute()); // display date as per calendar oled.setFontType(0); oled.setCursor(6,30); oled.print(Time.day()); oled.print("/"); oled.print(Time.month()); oled.print("/"); oled.print(Time.year()); oled.line(0,0,batHealth,0); oled.display(); delay(4000); } /**void myCalls(const char *event, const char *data) { }**/ void myHandler(const char *event, const char *data) { /** the coming data is in this format *<span style=\"color:#aaa\">1,590 </span>\n\n<span>45</span>\n\n<span>148</span> **/ values = data; cases = ""; deaths = ""; recovered = ""; //finding index of ">" and "<" and extracting inbetween numbers for active cases index1 = values.indexOf(">"); index2 = values.indexOf("<", index1); cases = values.substring(index1+1,index2).trim(); //shortening the string for next set of number extractions values = values.remove(0,index2); //replacing new line escape sequences and html tags all together using space character values = values.replace("</span>"," "); values = values.replace("<span>"," "); values = values.replace("\n"," "); values = values.trim(); // removing trailing whitespaces /**By now we have made our data look like this 45____148 from <span style=\"color:#aaa\">1,590 </span>\n\n<span>45</span>\n\n<span>148</span>**/ Serial.println(values); // you may try another logic too but keep in mind we will get different numbers from integration response each time there //is new COVID India case index1 = values.indexOf(" "); // find first space character and extract all digits before it index2 = values.lastIndexOf(" "); // find last space character and extract all digits after it deaths = values.substring(0,index1).trim(); recovered = values.substring(index2+1).trim(); // just for debugging nothing else Serial.print(cases); Serial.print(deaths); Serial.print(recovered); delay(4000); } int covidIndia() { // display India total COVID cases oled.clear(PAGE); oled.setFontType(0); oled.setCursor(18,12); oled.print("INDIA"); oled.setFontType(1); oled.setCursor(12,30); oled.print(cases); oled.line(0,0,batHealth,0); oled.display(); delay(4000); // display India COVID deaths oled.clear(PAGE); oled.setFontType(0); oled.setCursor(15,12); oled.print("DEATHS"); oled.setFontType(1); oled.setCursor(22,30); oled.print(deaths); oled.line(0,0,batHealth,0); oled.display(); delay(4000); // display India COVID recovers oled.clear(PAGE); oled.setFontType(0); oled.setCursor(5,12); oled.print("Recovered"); oled.setFontType(1); oled.setCursor(20,30); oled.print(recovered); oled.line(0,0,batHealth,0); oled.display(); delay(4000); } // just for OLED trial and testing void lines() { oled.clear(PAGE); int middleX = oled.getLCDWidth() / 2; int middleY = oled.getLCDHeight() / 2; int xEnd, yEnd; int lineWidth = min(middleX, middleY); for (int i=0; i<1; i++) { for (int deg=0; deg<360; deg+=15) { xEnd = lineWidth * cos(deg * PI / 180.0); yEnd = lineWidth * sin(deg * PI / 180.0); oled.line(middleX, middleY, middleX + xEnd, middleY + yEnd); oled.display(); delay(3); } for (int deg=0; deg<360; deg+=15) { xEnd = lineWidth * cos(deg * PI / 180.0); yEnd = lineWidth * sin(deg * PI / 180.0); oled.line(middleX, middleY, middleX + xEnd, middleY + yEnd, BLACK, NORM); oled.display(); delay(3); } } }
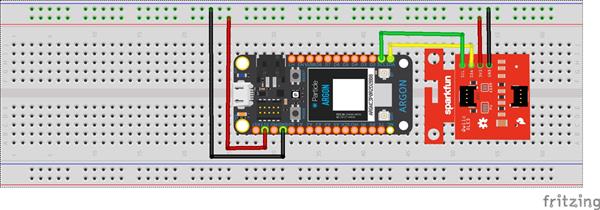
Coronavirus - India cases tracker
- Comments(0)
- Likes(0)

- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by vilaksh01
-
CliSensio - Climate Sensing and Insect Infestation Control Overview:Climate Change, is a highly debated high school topic, but do we really care about it? We s...
-
AI For truck APS Failure Detection on a $4 MCU IntroductionThe automotive industry is among the pioneers to adopt cutting-edge technologies, machin...
-
New Era Farming with TensorFlow on Lowest Power Consumption Overview:This project demonstrates how to build a device using TensorFlow and Artemis module to solv...
-
TinyML Gearbox Fault Prediction on a $4 MCU StoryIs it possible to make an AI-driven system that predicts gearbox failure on a simple $4 MCU? Ho...
-
AREC - Agricultural Records On Electronic Contracts Objective:One of the biggest reasons behind the growth of fake products in the agrochemical industry...
-
AquaMon - Aquaponics and Fish Tank Monitoring with Tuya IoT IntroductionDo you love sea creatures? I don't think I would find a single person who would not fall...
-
Predictive Maintenance Of Compressor Water Pumps An ordinary resident of a metropolis rarely thinks about how water enters taps or central heating pi...
-
Unlock Passwords With Tinyml-based Digit Recognition Story? IntroductionThe active development of smart gadgets with touch interfaces is trending nowaday...
-
Handmade Drawing Recognition Interface As From My Smartphone StoryIntroductionI was inspired by such features on our smartphones. My smartphone “Vivo V7” has a f...
-
Recognizing MNIST-based Handwritten Digits on M5Stack Core2 IntroductionIn my previous experiment, I had great fun adding handmade drawing gestures for our M5St...
-
Tuya Link SDK IoT Smart Environment Sensing And Humidifier IntroductionI had been exploring the Tuya IoT platform for the past few months and they never fail t...
-
Tuya IoT Cloud Smart Weather Lamp IntroductionHello everybody, ever felt like having a smart home device to visually show/signal you t...
-
Getting Started With Arduino IoT Control With Tuya Introduction:I have always enjoyed using Arduino UNO since it was my first programmable board but it...
-
Coronavirus - India cases tracker Today everyone is gazing at their phone to keep track of the coronavirus in their country but we ten...
-
CliSensio - Climate Sensing and Insect Infestation Control Overview:Climate Change, is a highly debated high school topic, but do we really care about it? We s...
-
AI For truck APS Failure Detection on a $4 MCU IntroductionThe automotive industry is among the pioneers to adopt cutting-edge technologies, machin...
-
New Era Farming with TensorFlow on Lowest Power Consumption Overview:This project demonstrates how to build a device using TensorFlow and Artemis module to solv...
-
TinyML Gearbox Fault Prediction on a $4 MCU StoryIs it possible to make an AI-driven system that predicts gearbox failure on a simple $4 MCU? Ho...
-
AREC - Agricultural Records On Electronic Contracts Objective:One of the biggest reasons behind the growth of fake products in the agrochemical industry...
-
AquaMon - Aquaponics and Fish Tank Monitoring with Tuya IoT IntroductionDo you love sea creatures? I don't think I would find a single person who would not fall...
-
Predictive Maintenance Of Compressor Water Pumps An ordinary resident of a metropolis rarely thinks about how water enters taps or central heating pi...
-
Unlock Passwords With Tinyml-based Digit Recognition Story? IntroductionThe active development of smart gadgets with touch interfaces is trending nowaday...
-
Handmade Drawing Recognition Interface As From My Smartphone StoryIntroductionI was inspired by such features on our smartphones. My smartphone “Vivo V7” has a f...
-
Recognizing MNIST-based Handwritten Digits on M5Stack Core2 IntroductionIn my previous experiment, I had great fun adding handmade drawing gestures for our M5St...
-
Tuya Link SDK IoT Smart Environment Sensing And Humidifier IntroductionI had been exploring the Tuya IoT platform for the past few months and they never fail t...
-
Tuya IoT Cloud Smart Weather Lamp IntroductionHello everybody, ever felt like having a smart home device to visually show/signal you t...
-
-
Instrumentation Input, high impedance with 16 bit 1MSPS ADC for SPI
251 0 0 -
RGB LED Matrix input module for the Framework Laptop 16
445 0 2 -
-
📦 StackBox: Modular MDF Storage Solution 📦
271 0 2 -
-