![]() |
arduino IDEArduino
|
Esp32 Smart Door
Controlling Esp32 Wirelessly with Blynk App
Blynk is an IoT Platform to control Arduino, Raspberry Pi, NodeMCU and other microcontrollers over the Internet. Blynk app can be downloaded from Google play store or Apple store.
Blynk app provides a digital dashboard where you can build a graphic interface for any IoT based project by simply dragging and dropping widgets. It's simple and easy to use IoT platform to build complex applications. Blynk is not bounded to some specific board or platform but it can be used with any microcontroller, provided that the microcontroller is connected to internet. Raspberry Pi has inbuilt Wi-Fi and other microcontroller like Arduino can be connected to internet using some Wi-Fi module like ESP8266 etc. We previously used Blynk App with Raspberry Pi to control its GPIO pin.
test code with blynk
#define BLYNK_PRINT Serial
#include <WiFi.h>
#include <WiFiClient.h>
#include <BlynkSimpleEsp32.h>
int pin = 2;
char auth[] = " "; // You should get Auth Token in the Blynk App.
char ssid[] = " "; // Your Wi-Fi Credentials
char pass[] = " ";
void setup() {
pinMode(pin, OUTPUT);
pinMode(pin, HIGH);
Serial.begin(115200);
delay(10);
Serial.print("Connecting to ");
Serial.println(ssid);
WiFi.begin(ssid, pass);
int wifi_ctr = 0;
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("WiFi connected");
Blynk.begin("Your auth. Token", ssid, pass);
}
void loop(){
Blynk.run();
}
Arduino is a great platform for beginners into the World of Microcontrollers and Embedded Systems. With a lot of cheap sensors and modules, you can make several projects either as a hobby or even commercial.
As technology advanced, new project ideas and implementations came into play and one particular concept is the Internet of Things or IoT. It is a connected platform, where several “things” or devices are connected over internet for exchange of information.
In DIY community, the IOT projects are mainly focused on Home Automation and Smart Home applications but commercial and industrial IoT projects have far complex implementations like Machine Learning, Artificial Intelligence, Wireless Sensor Networks etc.
The important thing in this brief intro is whether it is a small DIY project by a hobbyist or a complex industrial project, any IoT project must have connectivity to Internet. This is where the likes of ESP8266 and ESP32 come into picture.
If you want to add Wi-Fi connectivity to your projects, then ESP8266 is a great option. But if you want build a complete system with Wi-Fi connectivity, Bluetooth connectivity, high resolution ADCs, DAC, Serial Connectivity and many other features, then ESP32 is the ultimate choice.
What is ESP32?
ESP32 is a low-cost System on Chip (SoC) Microcontroller from Espressif Systems, the developers of the famous ESP8266 SoC. It is a successor to ESP8266 SoC and comes in both single-core and dual-core variations of the Tensilica’s 32-bit Xtensa LX6 Microprocessor with integrated Wi-Fi and Bluetooth.
The good thing about ESP32, like ESP8266 is its integrated RF components like Power Amplifier, Low-Noise Receive Amplifier, Antenna Switch, Filters and RF Balun. This makes designing hardware around ESP32 very easy as you require very few external components
Another important thing to know about ESP32 is that it is manufactured using TSMC’s ultra-low-power 40 nm technology. So, designing battery operated applications like wearables, audio equipment, baby monitors, smart watches, etc., using ESP32 should be very easy.
Specifications of ESP32
ESP32 has a lot more features than ESP8266 and it is difficult to include all the specifications in this Getting Started with ESP32 guide. So, I made a list of some of the important specifications of ESP32 here. But for complete set of specifications, I strongly suggest you to refer to the Datasheet.
Single or Dual-Core 32-bit LX6 Microprocessor with clock frequency up to 240 MHz.
520 KB of SRAM, 448 KB of ROM and 16 KB of RTC SRAM.
Supports 802.11 b/g/n Wi-Fi connectivity with speeds up to 150 Mbps.
Support for both Classic Bluetooth v4.2 and BLE specifications.
34 Programmable GPIOs.
Up to 18 channels of 12-bit SAR ADC and 2 channels of 8-bit DAC
Serial Connectivity include 4 x SPI, 2 x I2C, 2 x I2S, 3 x UART.
Ethernet MAC for physical LAN Communication (requires external PHY).
1 Host controller for SD/SDIO/MMC and 1 Slave controller for SDIO/SPI.
Motor PWM and up to 16-channels of LED PWM.
Secure Boot and Flash Encryption.
Cryptographic Hardware Acceleration for AES, Hash (SHA-2), RSA, ECC and RNG.
Different Ways to Program
A good hardware like ESP32 will be more user friendly if it can be programmed (writing code) in more than one way. And not surprisingly, the ESP32 supports multiple programming environments.
Some of the commonly used programming environments are:
Arduino IDE
PlatformIO IDE (VS Code)
LUA
MicroPython
Espressif IDF (IoT Development Framework)
JavaScript
As Arduino IDE is already a familiar environment, we will use the same to program ESP32 in our upcoming projects. But you can definitely try out others as well.
ESP32 DevKit – The ESP32 Development Board
Espressif Systems released several modules based on ESP32 and one of the popular options is the ESP-WROOM-32 Module. It consists of ESP32 SoC, a 40 MHz crystal oscillator, 4 MB Flash IC and some passive components.
Layout
We will see what a typical ESP32 Development Board consists of by taking a look at the layout of one of the popular low-cost ESP Boards available in the market called the ESP32 DevKit Board.
The following image shows the layout of an ESP32 Development Board which I have.
IMPORTANT NOTE: There are many ESP32 Boards based on ESP-WROOM-32 Module available in the market. The layout, pinout and features vary from board to board.
The board which I have has 30 Pins (15 pins on each side). There are some board with 36 Pins and some with slightly less Pins. So, double check the pins before making connections or even powering up the board.
An interesting point about the USB-to-UART IC is that its DTR and RTS pins are used to automatically set the ESP32 in to programming mode (whenever required) and also rest the board after programming.
Security
RSA-3072-based trusted application boot
AES256-XTS-based flash encryption to protect sensitive data at rest
4096-bit eFUSE memory with 2048 bits available for application
Digital signature peripheral for secure storage of private keys and generation of RSA signatures
Optimal Power Consumption
ESP32-S2 supports fine-resolution power-control through a selection of clock frequency, duty cycle, Wi-Fi operating modes and individual power control of its internal components.
When Wi-Fi is enabled, the chip automatically powers on or off the RF transceiver only when needed, thereby reducing the overall power consumption of the system.
ULP co-processor with less than 5 uA idle mode and 24 uA at 1% duty-cycle current consumption. Improved Wi-Fi-connected and MCU-idle-mode power consumption.
Software
ESP32-S2 supports Espressif’s software development framework (ESP-IDF), which is a mature and production-ready platform. Availability of common cloud connectivity agents and common product features shortens the time to market.
Applications
ESP32-S2 offers a universal Wi-Fi connectivity solution for a variety of applications, ranging from consumer to industrial use-cases. Furthermore, the computing power and memory expandability also makes it a suitable solution for simple ML-on-edge applications.
While it can support a large number of use-cases, the main target application use-cases are listed below:
Smart-Home Connectivity
Ranges from simple solutions like light bulbs, smart door-locks, smart sockets to white goods and kitchen appliances, over-the-top (OTT) devices and video streaming devices like security cameras
Supports Mesh Network, which can be applied to large-scale commercial lighting and smart-home network solutions.
Allows efficient interfacing with a wide range of sensors, which is suitable for the needs of different smart-home scenarios.
Battery-operated devices
Connected Wi-Fi sensors, Wi-Fi enabled toys, wearable and healthcare devices
Small 7 mm ? 7 mm QFN package, which is ideal for wearable devices
Low power consumption, in hibernation mode, of less than 5 uA enables application in battery-operated devices or long standby-time devices
QSPI/OPI supports multiple flash/SRAM chips for flexible configuration of NVM and volatile data storage
Industrial automation
Industrial automation includes wireless control and robotics, smart lighting, HVAC control, which can ensure high-quality technology development and a long life-cycle for products.
With its high RF performance and security features, it can meet strict requirements and high standards of reliability and efficiency for electronic control.
Retail & Catering Applications
POS machines and service robots
Advanced security features enable the protection of sensitive data on the chip and the flash device
Small form factor
With 14 highly sensitive touch sensors and an LCD interface, ESP32-S2 targets low-cost securely connected HMI devices, such as POS machines
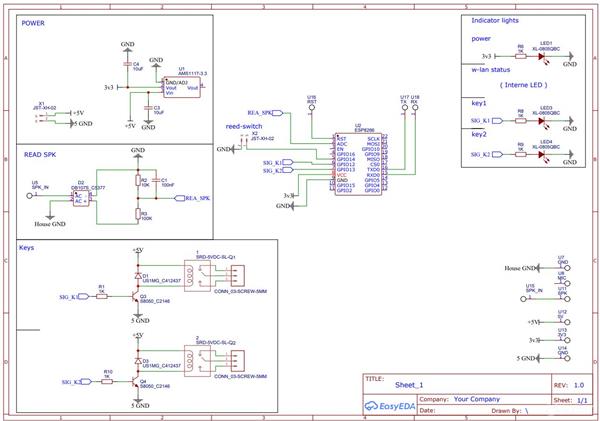
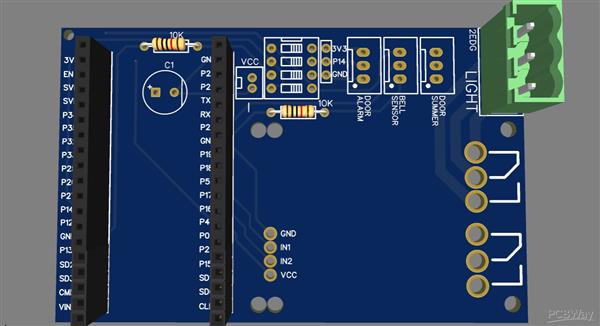
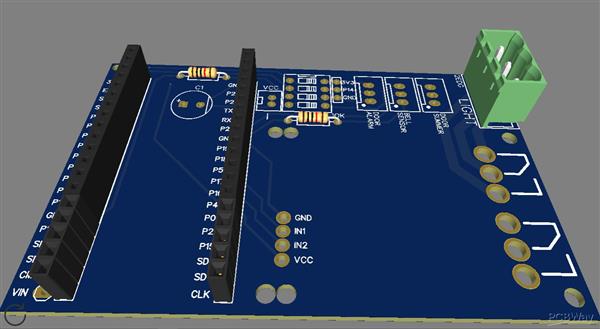
Esp32 Smart Door
*PCBWay community is a sharing platform. We are not responsible for any design issues and parameter issues (board thickness, surface finish, etc.) you choose.
- Comments(0)
- Likes(0)
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by Sreeram.zeno
-
Esp12-F Cluster V1.0 The ESP8266 is a low-cost Wi-Fi microchip, with built-in TCP/IP networking software, and microcontro...
-
TB6612FNG Motor Driver The TB6612FNG Motor Driver can control up to two DC motors at a constant current of 1.2A (3.2A peak)...
-
Sunny Buddy Solar Charger v1.0 This is the Sunny Buddy, a maximum power point tracking (MPPT) solar charger for single-cell LiPo ba...
-
Diy 74HC4051 8 Channel Mux Breakout Pcb The 74HC4051; 74HCT4051 is a single-pole octal-throw analog switch (SP8T) suitable for use in analog...
-
Diy RFM97CW Breakout Pcb IntroductionLoRa? (standing for Long Range) is a LPWAN technology, characterized by a long range ass...
-
ProMicro-RP2040 Pcb The RP2040 is a 32-bit dual ARM Cortex-M0+ microcontroller integrated circuit by Raspberry Pi Founda...
-
Serial Basic CH340G Pcb A USB adapter is a type of protocol converter that is used for converting USB data signals to and fr...
-
Mp3 Shield For Arduino Hardware OverviewThe centerpiece of the MP3 Player Shield is a VS1053B Audio Codec IC. The VS1053B i...
-
MRK CAN Shield Arduino The CAN-BUS Shield provides your Arduino or Redboard with CAN-BUS capabilities and allows you to hac...
-
AVR ISP Programmer AVR is a family of microcontrollers developed since 1996 by Atmel, acquired by Microchip Technology ...
-
Diy Arduino mega Pcb The Arduino Mega 2560 is a microcontroller board based on the ATmega2560. It has 54 digital input/ou...
-
Max3232 Breakout Board MAX3232 IC is extensively used for serial communication in between Microcontroller and a computer fo...
-
Line Follower Pcb The Line Follower Array is a long board consisting of eight IR sensors that have been configured to ...
-
HMC6343 Accelerometer Module The HMC6343 is a solid-state compass module with tilt compensation from Honeywell. The HMC6343 has t...
-
RTK2 GPS Module For Arduino USBThe USB C connector makes it easy to connect the ZED-F9P to u-center for configuration and quick ...
-
Arduino Explora Pcb The Arduino Esplora is a microcontroller board derived from the Arduino Leonardo. The Esplora differ...
-
Diy Stepper Motor Easy Driver A motor controller is a device or group of devices that can coordinate in a predetermined manner the...
-
Diy Arduino Pro Mini The Arduino Pro Mini is a microcontroller board based on the ATmega168 . It has 14 digital input/out...
-
-
-
kmMiniSchield MIDI I/O - IN/OUT/THROUGH MIDI extension for kmMidiMini
128 0 0 -
DIY Laser Power Meter with Arduino
190 0 2 -
-
-
Box & Bolt, 3D Printed Cardboard Crafting Tools
171 0 2 -