|
ARDUINO_NANO |
x 1 | |
|
I2C 16x2 Arduino LCD Display Module |
x 1 | |
|
button |
x 4 | |
|
resistors |
x 6 | |
|
capacitor 470µF |
x 1 |
![]() |
arduino IDEArduino
|
|
![]() |
Soldering Iron Kit |
How to make Arduino LED Tester + Resistor Calculator
Light Emitting Diode as an electronic component are very often used in electronic devices. There are many different types with specific characteristics such as forward voltage and maximum current. Most often it is necessary to connect them to a higher voltage where it is necessary to connect a series resistor with a certain value.
The device described in this video is a useful tool for testing, and determining characteristics of LEDs, as well as a calculator for calculating the series resistor depending on the connected voltage.
The idea for this project was taken from Dave Cook's model, processed with an Arduino from Jaycar Electronics, and I made some changes to the code where uses an I2C LCD display, and dedicated buttons to control the device, instead of an LCD keypad shield.
The device is very simple to build, and consists of several components:
- Arduino Nano microlontroller
- 16x2 LCD Display with I2C communication protocol
- 7 Resistors
- capacitor
- and four Buttons
If you want to make a PCB for this project, or for any other electronic project, PCBway is a great choice for you. PCBway is one of the most experienced PCB manufacturing company in China in field of PCB prototype and fabrication. They provide completed PCB assembly service with worldwide free shipping , and ISO9001 quality control system. Also, on their site there is an online gerber viewer where you can upload your gerber and drill files to render your board.
And now let's see how the tester works in real conditions. When turning on the display appear previously defined values for the current and voltage to which the LED would be connected, namely a current of 10mA and a voltage of 14V. With no LED attached, it will not show a resistor value, and current value blinking.
The upper buttons can be used to set the current that will flow through the LED in the range from 1 to 20mA. These are values that in most cases cannot cause damage to the LED. The lower buttons set the value of the voltage to which the diode would be connected through a series resistor in the real circuit. This voltage can be set from 1 to 99 V, and the display then shows the required resistor value depending on the selected voltage and current. Also on the lower right part of the display appears the code of that resistor under which we can buy it in Jaycar Electronic shop. If the resistor would dissipate more than half a watt, a P flashes on the top line to let you know you might need to choose a more powerful resistor. If by mistake we connect the diode with reverse polarity, the diode remains functional, it just won't light up.
And finally a short conclusion. The device presented in this project is very simple to build, but it is a really useful instrument in the laboratory, especially due to the fact that many different types of LEDs are used nowadays. The device is embedded in a suitable box made of PVC material and lined with self-adhesive colored wallpaper
/*
Arduino LED Tester
DPIN--39R--+--10R---TESTLED---GND
| | |
470u ATOP ABOT
|
GND
Measures LED characteristics by charging up the cap to deliver target current and find forward voltage
From target current, we can calculate R to be used with a design supply voltage and a matching part number.
*/
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
//lcd update interval
#define LCDINT 500
//key check interval
#define KEYINT 200
//pin for buttons
#define KEYPIN A0
//button constants
#define btnRIGHT 6
#define btnUP 5
#define btnDOWN 4
#define btnLEFT 3
#define btnSELECT 2
#define btnNONE (-1)
LiquidCrystal_I2C lcd(0x27,16,2); // set the LCD adress
//Globals for display
long itest=10; //test current, in mA
long vset =14000; //display voltage in mV
long vled,vrr,irr,pset; //LED voltage, Resistor voltage, resistor current, display power
//resistors in Jaycar 1/2 W range, part nos start at RR0524 for 10R
#define RCOUNT 121
long rvals[]={10,11,12,13,15,16,18,20,22,24,27,30,33,36,39,43,47,51,56,62,68,75,82,91,100,110,120,130,150,160,180,200,220,240,270,300,330,360,390,430,470,510,560,620,680,750,820,910,1000,1100,1200,1300,1500,1600,1800,2000,2200,2400,2700,3000,3300,3600,3900,4300,4700,5100,5600,6200,6800,7500,8200,9100,10000,11000,12000,13000,15000,16000,18000,20000,22000,24000,27000,30000,33000,36000,39000,43000,47000,51000,56000,62000,68000,75000,82000,91000,100000,110000,120000,130000,150000,160000,180000,200000,220000,240000,270000,300000,330000,360000,390000,430000,470000,510000,560000,620000,680000,750000,820000,910000,1000000};
long lastlcd=0; //time of last lcd update
long lastkey=0; //time of last key check
int lcdflash=0; //lcd flashing phase variable
long pdes;
long rval; //calculated resistor value for display
long rindex; //index of selected resistor in rvals[]
int pwmout=0; //pwm output of current driver
int rvalid=0; //flag if resistor value is valid
//pins for test interface
#define ATOP A2
#define ABOT A3
#define DPIN 3
#define OSAMP 16
void setup() {
Serial.begin(9600);
// lcd.begin(16, 2); //lcd
lcd.begin();
lcd.clear();
lcd.backlight();
pinMode(DPIN,OUTPUT); //pwm pin
}
void loop() {
long atop,abot,arr; //analog sample values
rvalid=0; //set flag to not valid
atop=analogoversample(ATOP,OSAMP)/OSAMP;
abot=analogoversample(ABOT,OSAMP)/OSAMP;
arr=atop-abot; //this is the analog value across the 10R sense resistor
if(arr<0){arr=0;} //sanity check
vled=abot*5000/1023; //5000mV=1023 => voltage across LED
vrr=arr*5000/1023; //voltage across sense resistor
irr=vrr/10; //led and resistor current in mA (cos it's a 10R resistor)
if(irr<itest){pwmout++;if(pwmout>255){pwmout=255;}} //ramp up current if too low
if(irr>itest){pwmout--;if(pwmout<0){pwmout=0;}} //ramp down if too high
if(irr>24){pwmout=pwmout-5;if(pwmout<0){pwmout=0;}} //ramp down quick if too too high
if(irr>itest*3){pwmout=pwmout-5;if(pwmout<0){pwmout=0;}} //ramp down quick if too too high
analogWrite(DPIN,pwmout); //output new PWM
rval=(vset-vled)/itest; //mV/mV => ohms resistance of display resistor
for(int i=0;i<RCOUNT;i++){ //find next highest E24 value
if(rvals[i]>=rval){rindex=i;rval=rvals[rindex];i=RCOUNT+1;rvalid=1;}
}
if(abs(irr-itest)>(itest/5)+1){rvalid=0;} //has current settled within 20%?
if(vled>vset){rvalid=0;} //if vled>vset, no valid resistor exists
pset=0; //work out dissipation in ballast resistor if valid)
if(rvalid){pset=itest*itest*rval;} //this will be in microwatts (milliamps squared)
if(millis()-lastlcd>LCDINT){ //check if display due to be updated
lastlcd=millis();
dolcd(); //update display
lcdflash=1-lcdflash; //toggle flash variable
}
if(millis()-lastkey>KEYINT){ //check if keys due to be checked
lastkey=millis();
dobuttons();
}
delay(1);
} //end of loop
void dolcd(){
lcd.setCursor(0,0); //first line
//milliamps
if(lcdflash||rvalid){ //show if correct if on flashing phase
if(itest>9){lcd.write(((itest/10)%10)+'0');}else{lcd.write(' ');} //blank tens if zero
lcd.write((itest%10)+'0');
}
else{ //blank if not
lcd.write(' ');lcd.write(' ');
}
lcd.write('m');lcd.write('A');lcd.write(' ');
//VLED
lcd.write(((vled/1000)%10)+'0');
lcd.write('.');
lcd.write(((vled/100)%10)+'0');
lcd.write('V');lcd.write(' ');
//actual LED current
if(irr>9){lcd.write(((irr/10)%10)+'0');}else{lcd.write(' ');} //blank tens if zero
lcd.write((irr%10)+'0');
lcd.write('m');lcd.write('A');lcd.write(' ');
if((pset>499999)&&(lcdflash)){lcd.write('P');}else{lcd.write(' ');} //flash P if power above 1/2 watt
lcd.setCursor(0,1); //second line
//
if(vset>9999){lcd.write(((vset/10000)%10)+'0');}else{lcd.write(' ');} //tens of vset, blank if zero
lcd.write(((vset/1000)%10)+'0'); //units of vset
lcd.write('V');lcd.write(' ');
if(rvalid){
lcdprintrval(rval); //resistor value (4 characters)
lcd.write(' ');
lcdprintpartno(rindex); //resistor part no (6 characters)
if(pset>499999){lcd.write('!');}else{lcd.write(' ');} //show ! if power above 1/2 watt
}else{
lcd.write(' ');
lcd.write('-');
lcd.write('-');
lcd.write('-');
lcd.write(' ');
lcd.write('-');
lcd.write('-');
lcd.write('-');
lcd.write('-');
lcd.write('-');
lcd.write('-');
lcd.write(' ');
}
}
void lcdprintpartno(int index){
//part number
lcd.write('R');
lcd.write('R');
lcd.write('0');
lcd.write((((index+524)/100)%10)+'0'); //part no's start at RR0524 for 10R
lcd.write((((index+524)/10)%10)+'0');
lcd.write((((index+524))%10)+'0');
}
void lcdprintrval(long rval){ //print a value in 10k0 format, always outputs 4 characters
long mult=1;
long modval;
if(rval>999){mult=1000;}
if(rval>999999){mult=1000000;}
modval=(10*rval)/mult; //convert to final format, save a decimal place
if(modval>999){ //nnnM
lcd.write(((modval/1000)%10)+'0');
lcd.write(((modval/100)%10)+'0');
lcd.write(((modval/10)%10)+'0');
lcdprintmult(mult);
}else{
if(modval>99){ //nnMn
lcd.write(((modval/100)%10)+'0');
lcd.write(((modval/10)%10)+'0');
lcdprintmult(mult);
lcd.write(((modval)%10)+'0');
}else{ //_nMn
lcd.write(' ');
lcd.write(((modval/10)%10)+'0');
lcdprintmult(mult);
lcd.write(((modval)%10)+'0');
}
}
}
void lcdprintmult(long mult){ //helper function to print multiplier
switch (mult){
case 1: lcd.print('R');break;
case 1000: lcd.print('k');break;
case 1000000: lcd.print('M');break;
default: lcd.print('?');break;
}
}
int read_LCD_buttons(){
int adc_key_in = 0;
adc_key_in = analogRead(KEYPIN); // read the value from the sensor
delay(5); //switch debounce delay. Increase this delay if incorrect switch selections are returned.
int k = (analogRead(KEYPIN) - adc_key_in); //gives the button a slight range to allow for a little contact resistance noise
if (5 < abs(k)) return btnNONE; // double checks the keypress. If the two readings are not equal +/-k value after debounce delay, it tries again.
// my buttons when read are centered at these valies: 0, 144, 329, 504, 741
// we add approx 50 to those values and check to see if we are close
if (adc_key_in > 1000) return btnNONE; // We make this the 1st option for speed reasons since it will be the most likely result
if (adc_key_in < 50) return btnRIGHT;
if (adc_key_in < 195) return btnUP;
if (adc_key_in < 380) return btnDOWN;
if (adc_key_in < 555) return btnLEFT;
if (adc_key_in < 790) return btnSELECT;
return btnNONE; // when all others fail, return this...
}
void dobuttons(){ //updates variables. debounces by only sampling at intervals
int key;
key = read_LCD_buttons();
if(key==btnLEFT){itest=itest-1;if(itest<1){itest=1;}}
if(key==btnRIGHT){itest=itest+1;if(itest>20){itest=20;}}
if(key==btnUP){vset=vset+1000;if(vset>99000){vset=99000;}}
if(key==btnDOWN){vset=vset-1000;if(vset<0){vset=0;}}
}
long analogoversample(int pin,int samples){ //read pin samples times and return sum
long n=0;
for(int i=0;i<samples;i++){
n=n+analogRead(pin);
}
return n;
}
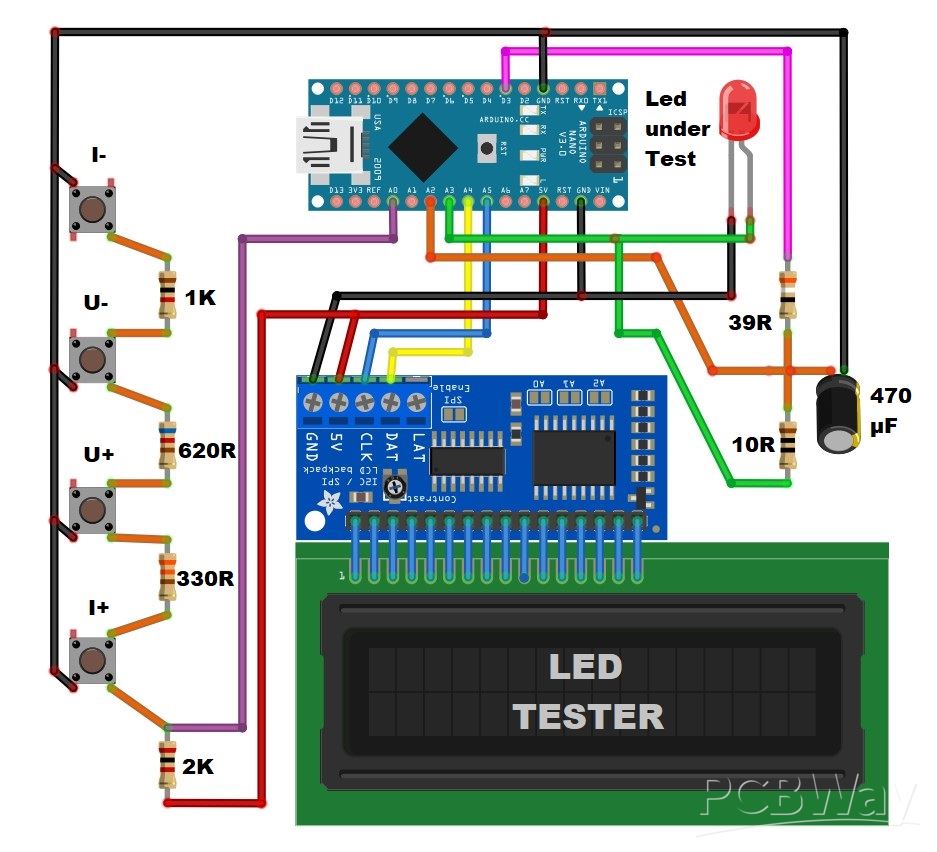
How to make Arduino LED Tester + Resistor Calculator
- Comments(0)
- Likes(0)
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by Mirko Pavleski
-
How to make simplest possible autorange Capacitance meter The capacity measurement option is usually only available in more expensive multimeters, so it is d...
-
How to turn mini Tesla Coil from Aliexpress into a Real Powerful SSTC with big spark Some time ago I bought a mini tesla coil kit from an online store that cost less than $4. It's a ve...
-
DIY Arduino ultrasonic Sonar - Radar on TFT display Ultrasonic sonar is devices that use sound waves with frequencies higher than the upper audible lim...
-
Simple ESP32 Internet radio on VFD Display Internet radio, also known as online radio or streaming radio, refers to the broadcasting of audio ...
-
Short review of small music Tesla Coil with Bluetooth A Solid State Tesla Coil (SSTC) is a type of Tesla coil that uses solid-state electronic components...
-
Testing 2500W Large Induction Heater An induction heater is a device that uses electromagnetic induction to heat objects. It consists of...
-
Arduino FFT Audio Spectrum analyzer on 8x32 color matrix WS2812B The spectrum analyzer displays the amplitude of signals as a function of frequency, allowing engine...
-
Unusual Led Ring Arduino Clock, Temperature, and Humidity meter There are many arduino clock projects with temperature and humidity readings where the results are ...
-
How to make simple portable PEMF Magnetic Pulser PEMF (Pulsed Electromagnetic Field Therapy), which is also known as magnetic pulse therapy uses ele...
-
Single Mosfet Mini SSTC Tesla coil with 10 + cm Spark A Solid State Tesla Coil (SSTC) is a type of Tesla coil that uses solid-state components such as tr...
-
How to Make EM84 (6E2) Vacuum Tube Stereo VU Meter A VU meter, also known as a Volume Unit meter, is a device used to display the audio signal level ...
-
Universal Arduino Staccato controller for SSTC and VTCC Tesla Coils The VTTC Staccato Controller was developed in the attempt to create longer sparks from VTTCs while ...
-
DIY extremly Sensitive and cheap Arduino Seismometer A seismometer, is an instrument used to detect and record ground motion caused by seismic waves, su...
-
DIY Extremly Sensitive and cheap Geophone sensor for Earthquakes detecting A geophone is a device used in geophysics to detect ground movement. It is specifically designed to...
-
Arduino FFT Spectrum analyzer on VFD display GP1287 An audio spectrum analyzer is a device that visualizes the frequency content of an audio signal. It...
-
DIY simple Spark Gap Tesla Coil Tesla coil is a type of resonant transformer circuit invented by Nikola Tesla around 1891. It is a ...
-
DIY PC controlled high power PEMF Therapy Device PEMF or Pulsed Electromagnetic Field therapy is a type of therapy that uses electromagnetic fields ...
-
DIY Precise Arduino Miliohmmeter In practice, we very often need to measure low resistances. Measuring low-value resistances, especi...
-
Creative Micro Designs Inc. CMD FD-2000 / FD-4000 3D Printable Case
198 0 0 -
Creative Micro Designs Inc. CMD FD-2000 / FD-4000 Metal Case
259 0 0 -
-
-
IoT Indoor system with ESP32 to monitor Temperature, Humidity, Pressure, and Air Quality
744 0 3 -
Naruto Multi-color PCB printed with UV technology
263 2 1 -
-