|
Wio Rp2040 Mini DEV BoardSeeedstudio
|
x 1 | |
|
CircuitpythonAdafruit
|
x 1 | |
|
Adafruit Circuitpython ESP32_SPI LibraryAdafruit
|
x 1 |
The Adventures of Porting Circuitpython to Wio RP2040
I have been developing some electronic props solutions for Escape Rooms. And periodically I usually acquire new controllers to study the feasibility of some solutions. Recently, I'm porting some of my solutions in C++ (Arduino) to Circuitpython, for the ease of use and maintenance of the code.
In my recent research, I found this solution from Seeed Studio with a cost-benefit ratio that I found quite interesting. This is the WIO RP2040, a solution that combines RP2040 and a Wifi co-processor.
Well, some questions were left unanswered even in the documentation and product description.
Specification:
Raspberry Pi Foundation's RP2040 microcontroller
- Dual Cortex M0+ processors, up to 133 MHz
- 264 kB of embedded SRAM in 6 banks
- 2MB QSPI Flash Memory
- On-board USB-C Connector for programming
- USB 1.1 Host/Device functionality
- 20x multifunctional GPIO Pins
2.4GHz Wireless connectivity (which co-processor? )
- Support IEEE802.11 b/g/n
- Support 2.4 ~ 2.4835 GHz
- Support Ap & Station mode
Buttons
Boot
Reset(RUN)
LEDs
Power
User-programmable LED(GP13)
Power Supply(2 methods)
5V via USB-C port(default)
VIN(5V~3.6V) and GND header pins
Dimensions: 25.8 x 45.5mm
Support programming languages
- MicroPython
- C/C++
For example, what is the wifi coprocessor and how is it connected to the RP 2040? Although Seeed has made available a library for Eagle, a library for Arduino, firmware for use with Micropython and a series of tutorials on its Wiki, developers are lacking crucial information to port other firmware to their board. This post is about my investigations in this regard.
The proposal is for this post to be updated as soon as the information I'm looking for is validated by Seeed, myself or the openSource community.
First question: What is the wifi co-processor?
At the time of writing this post, SeeedStudio has at least updated the product page, indicating that the ESP8285 is the Wifi co-processor, as you can see from the link: https://www.seeedstudio.com/Wio- RP2040-Module-p-4932.html The problem is that this information is not available in all the documentation related to the module and I only found it after some hard Google searches. The solution is almost the same used by another card, the Challenger RP2040 Wifi, this one has already been ported to Circuitpython.
Well, once I found that information, I downloaded the Challenger firmware, to test at least some degree of compatibility. Obviously that didn't work. Unlike the Seeed board, the Challenger is very well documented and we soon understand how the communication between the RP2040 and ESP8285 is done: https://ilabs.se/challenger-rp2040-wifi-datasheet/
The files to be analyzed are at https://github.com/IsQianGe/rp2040-spi/tree/master/ports/rp2
machine_spi.c mod_wifi_spi.c wifi_spi.c wifi_spi.h
Looking through these files, I was able to find some valuable information. The RP2040 is connected to the ESP8285 using the second SPI port.
SCK > GP10
MOSI > GP11
MISO > GP8
CS > GP9
But there were still some gaps to fill:
What would that pin be?
SPI_HANDSHAKE > GP21
Well, now is the time to see how Circuitpython communicates with Espressif chips...
The library used by Challenger is Adafruit_Circuitpython_ESP32_SPI, which has well-documented examples.
When we are going to initialize Wifi using this library, we need an SPI object already configured and the assignment of these three pins:
esp32_cs = DigitalInOut(board.ESP_CS)
esp32_ready = DigitalInOut(board.ESP_BUSY)
esp32_reset = DigitalInOut(board.ESP_RESET)
Well, a good deduction is that the pin described as Esp_ready in this library corresponds to SPI_HANDSHAKE in the other. But what would be the RESET pin?
It's time for more investigation.
Seeed already provides a repository with the Hardware for the Arduino IDE board manager.
The step by step to install the support is in this link: https://wiki.seeedstudio.com/Wio-RP2040-with-Arduino-WIFI/
The files will be installed in your User folder: Users\<YOURUSER>\AppData\Local\Arduino15\packages\Seeeduino\hardware\rp2040\1.9.3
Dig into it and find, at libraries folder, the Wifi/src/utility subfolder.
Find the spi_drv.cpp and inside it, you will find this information:
#define NINA_GPIO0 (21u)
#define SPIWIFI_SS (24u)
#define SPIWIFI_ACK (21u)
#define SPIWIFI_RESET (22u)
...
static uint8_t SLAVESELECT = 9; // ss
static uint8_t SLAVEREADY = 21; // handshake pin
static uint8_t SLAVERESET = 22; // reset pin
static uint8_t SLAVEPOWER = 25; // A power pin? What a hell!
Well, appears that we find all info that we need.
Resuming:
In this Seeed solution, the RP2040 communicates with the ESP8285 using SPI1.
The pins are:
SCK > GP10
MOSI > GP11
MISO > GP8
CS > GP9
ESP32_READY, ACKNOWLEDGE or Handshake > GP21
ESP32_RESET > GP22
ESP_POWER > GP25
Time to compile my port of Circuitpython...
Porting Circuitpython to this board
The process is not very different from what I've done on the other Seeed board, in this tutorial here: https://www.pcbway.com/project/shareproject/Circuitpython_on_Seeed_XIAO_RP2040_efd3eaef.html
Follow the lead. The Adafruit team has already made an excellent tutorial on this step.
https://learn.adafruit.com/building-circuitpython/build-circuitpython
At Linux (or WSL) you can do this:
git clone https://github.com/adafruit/circuitpython.git
cd circuitpython
make fetch-submodules
# Install pip if it is not already installed (Linux only)
sudo apt install python3-pip
# Install needed Python packages from pypi.org.
pip3 install -r requirements-dev.txt
make -C mpy-cross
# Go to boards folder and make a copy of default board (raspberry_pi_pico) with a new name
cd ports/raspberrypi/boards
cp -r raspberry_pi_pico wio_rp2040
This new folder is an exact copy of default raspberry pi pico definitions. Then you need to change some stuff on certain files:
The mpconfigboard.h file:
#define MICROPY_HW_BOARD_NAME "Seeed Wio RP2040"
#define MICROPY_HW_MCU_NAME "rp2040"
#define DEFAULT_UART_BUS_TX (&pin_GPIO12)
#define DEFAULT_UART_BUS_RX (&pin_GPIO13)
#define DEFAULT_I2C_BUS_SDA (&pin_GPIO0)
#define DEFAULT_I2C_BUS_SCL (&pin_GPIO1)
#define DEFAULT_SPI_BUS_SCK (&pin_GPIO18)
#define DEFAULT_SPI_BUS_MOSI (&pin_GPIO19)
#define DEFAULT_SPI_BUS_MISO (&pin_GPIO16)
Then the pins.c file:
#include "shared-bindings/board/__init__.h"
STATIC const mp_rom_map_elem_t board_module_globals_table[] = {
CIRCUITPYTHON_BOARD_DICT_STANDARD_ITEMS
{ MP_ROM_QSTR(MP_QSTR_SDA), MP_ROM_PTR(&pin_GPIO0) },
{ MP_ROM_QSTR(MP_QSTR_GP0), MP_ROM_PTR(&pin_GPIO0) },
{ MP_ROM_QSTR(MP_QSTR_SCL), MP_ROM_PTR(&pin_GPIO1) },
{ MP_ROM_QSTR(MP_QSTR_GP1), MP_ROM_PTR(&pin_GPIO1) },
{ MP_ROM_QSTR(MP_QSTR_GP2), MP_ROM_PTR(&pin_GPIO2) },
{ MP_ROM_QSTR(MP_QSTR_GP3), MP_ROM_PTR(&pin_GPIO3) },
{ MP_ROM_QSTR(MP_QSTR_GP4), MP_ROM_PTR(&pin_GPIO4) },
{ MP_ROM_QSTR(MP_QSTR_GP5), MP_ROM_PTR(&pin_GPIO5) },
{ MP_ROM_QSTR(MP_QSTR_GP6), MP_ROM_PTR(&pin_GPIO6) },
{ MP_ROM_QSTR(MP_QSTR_GP7), MP_ROM_PTR(&pin_GPIO7) },
# WIFI SPI DEFINITIONS
{ MP_ROM_QSTR(MP_QSTR_WIFI_MISO), MP_ROM_PTR(&pin_GPIO8) },
{ MP_ROM_QSTR(MP_QSTR_GP8), MP_ROM_PTR(&pin_GPIO8) },
{ MP_ROM_QSTR(MP_QSTR_WIFI_SS), MP_ROM_PTR(&pin_GPIO9) },
{ MP_ROM_QSTR(MP_QSTR_GP9), MP_ROM_PTR(&pin_GPIO9) },
{ MP_ROM_QSTR(MP_QSTR_WIFI_SCK), MP_ROM_PTR(&pin_GPIO10) },
{ MP_ROM_QSTR(MP_QSTR_GP10), MP_ROM_PTR(&pin_GPIO10) },
{ MP_ROM_QSTR(MP_QSTR_WIFI_MOSI), MP_ROM_PTR(&pin_GPIO11) },
{ MP_ROM_QSTR(MP_QSTR_GP11), MP_ROM_PTR(&pin_GPIO11) },
{ MP_ROM_QSTR(MP_QSTR_ESP_BUSY), MP_ROM_PTR(&pin_GPIO21) },
{ MP_ROM_QSTR(MP_QSTR_WIFI_ACK), MP_ROM_PTR(&pin_GPIO21) },
{ MP_ROM_QSTR(MP_QSTR_GP21), MP_ROM_PTR(&pin_GPIO21) },
{ MP_ROM_QSTR(MP_QSTR_ESP_RESET), MP_ROM_PTR(&pin_GPIO22) },
{ MP_ROM_QSTR(MP_QSTR_WIFI_RESET), MP_ROM_PTR(&pin_GPIO22) },
{ MP_ROM_QSTR(MP_QSTR_GP22), MP_ROM_PTR(&pin_GPIO22) },
{ MP_ROM_QSTR(MP_QSTR_ESP_CS), MP_ROM_PTR(&pin_GPIO24) }, # I am not sure of this one...
{ MP_ROM_QSTR(MP_QSTR_GP24), MP_ROM_PTR(&pin_GPIO24) },
{ MP_ROM_QSTR(MP_QSTR_ESP_POWER), MP_ROM_PTR(&pin_GPIO25) },
{ MP_ROM_QSTR(MP_QSTR_WIFI_POWER), MP_ROM_PTR(&pin_GPIO25) },
{ MP_ROM_QSTR(MP_QSTR_GP25), MP_ROM_PTR(&pin_GPIO25) },
# ON BOARD LED
{ MP_ROM_QSTR(MP_QSTR_LED), MP_ROM_PTR(&pin_GPIO13) }, // Changed to 13
{ MP_ROM_QSTR(MP_QSTR_GP12), MP_ROM_PTR(&pin_GPIO12) },
{ MP_ROM_QSTR(MP_QSTR_GP14), MP_ROM_PTR(&pin_GPIO14) },
{ MP_ROM_QSTR(MP_QSTR_GP15), MP_ROM_PTR(&pin_GPIO15) },
{ MP_ROM_QSTR(MP_QSTR_GP16), MP_ROM_PTR(&pin_GPIO16) },
{ MP_ROM_QSTR(MP_QSTR_GP17), MP_ROM_PTR(&pin_GPIO17) },
{ MP_ROM_QSTR(MP_QSTR_GP18), MP_ROM_PTR(&pin_GPIO18) },
{ MP_ROM_QSTR(MP_QSTR_GP19), MP_ROM_PTR(&pin_GPIO19) },
{ MP_ROM_QSTR(MP_QSTR_A0), MP_ROM_PTR(&pin_GPIO26) },
{ MP_ROM_QSTR(MP_QSTR_GP26), MP_ROM_PTR(&pin_GPIO26) },
{ MP_ROM_QSTR(MP_QSTR_A1), MP_ROM_PTR(&pin_GPIO27) },
{ MP_ROM_QSTR(MP_QSTR_GP27), MP_ROM_PTR(&pin_GPIO27) },
{ MP_ROM_QSTR(MP_QSTR_A2), MP_ROM_PTR(&pin_GPIO28) },
{ MP_ROM_QSTR(MP_QSTR_GP28), MP_ROM_PTR(&pin_GPIO28) },
{ MP_ROM_QSTR(MP_QSTR_A3), MP_ROM_PTR(&pin_GPIO29) },
{ MP_ROM_QSTR(MP_QSTR_GP29), MP_ROM_PTR(&pin_GPIO29) },
{ MP_ROM_QSTR(MP_QSTR_I2C), MP_ROM_PTR(&board_i2c_obj) },
{ MP_ROM_QSTR(MP_QSTR_SPI), MP_ROM_PTR(&board_spi_obj) },
{ MP_ROM_QSTR(MP_QSTR_UART), MP_ROM_PTR(&board_uart_obj) },
};
MP_DEFINE_CONST_DICT(board_module_globals, board_module_globals_table);
Save files and then go to ports folder and compile the firmware to the board.
cd ports/raspberrypi
make BOARD=wio_rp2040
Once ended, the compiled firmware will be on ports/raspberry/build-wio_rp2040 folder named as firmware.uf2
Simply reset WIO RP2040 with the BOOT button pressed and then upload the firmware.
(My compiled version is in this link: https://github.com/djairjr/Wio-Rp2040---Circuitpython-port-notes/blob/main/firmware.uf2, but not working version yet...)
Testing the port
After install the firmware and adafruit_circuitpython_esp32spi library, I couldn't access the wifi from the SPI. Timeout errors appear. There is probably a correct boot sequence that should be found in the libraries for Arduino and Micropython, which already work with this board.
All the other stuff is perfect. At present day, I am working in a test with REPL, trying to start manually de WIFI co-processor from SPI Bus.
Final comments
It's not an easy task to port a card when the documentation is poor.
It demands a lot of collective work, research by users and buyers.
Although Seeed's solution is quite attractive in terms of cost-effectiveness, it ends up demanding unpaid work on our part.
It's like you buy a bottle of water, you can't open the cap and you have to buy a tool to do it.
I will continue to publish my findings, but for now, I do not recommend buying this board.
Please wait until Seeed improves its support and documentation.
The Adventures of Porting Circuitpython to Wio RP2040
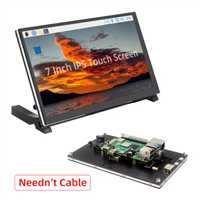
Raspberry Pi 5 7 Inch Touch Screen IPS 1024x600 HD LCD HDMI-compatible Display for RPI 4B 3B+ OPI 5 AIDA64 PC Secondary Screen(Without Speaker)
BUY NOW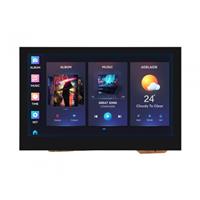
ESP32-S3 4.3inch Capacitive Touch Display Development Board, 800×480, 5-point Touch, 32-bit LX7 Dual-core Processor
BUY NOW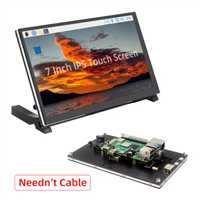
Raspberry Pi 5 7 Inch Touch Screen IPS 1024x600 HD LCD HDMI-compatible Display for RPI 4B 3B+ OPI 5 AIDA64 PC Secondary Screen(Without Speaker)
BUY NOW- Comments(2)
- Likes(1)

- Simen E. SørensenSep 23,20220 CommentsReply
- EngineerAug 07,20220 CommentsReply
-
rickybrent Jun 10,2022
- 1 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10

-
8design
-
8usability
-
8creativity
-
10content
More by nicolaudosbrinquedos
-
Alastor Moody Eye using Raspberry Pi Pico, CircuitPython and Round Display GC9A01 I am developing a series of objects for a children's play about fear and terror. And then I got insp...
-
The Crazy Pots Game At some point on the internet I came across someone who had made a game like that, but unfortunately...
-
Arduino Mastermind Game I created this little game as a hobby for my children during the Covid-19 quarantine. I had already ...
-
Raspberry Pi Pico With I2C Oled Display and CircuitPython This is my first experience using this little board from Raspberry Pi Foundation.I preferred to inst...
-
Raspberry Pi Pico and TFT ILI9341 with Circuit Python I decided to write another tutorial on the Raspberry Pi Pico, mainly because the card is very recent...
-
Arduino Minesweeeper It was then that I found the works of Rachit Belwariar, on the page https://www.geeksforgeeks.org/cp...
-
Homeassistant in Beaglebone Black With Debian 11 Intro: The primary objective of my project was to give some use to the Beaglebone Black that has bee...
-
The Adventures of Porting Circuitpython to Wio RP2040 I have been developing some electronic props solutions for Escape Rooms. And periodically I usually ...
-
Simple Electronics to Escape Room Owners - Using a bunch of voltmeters with PCA9685 This is a preliminary study for an Escape Room game. My main goal was to add as many analog displays...
-
Simple Electronics to Escape Room Owners - First Chapter I've been developing puzzles and artifacts for Escape Room since 2018 and most of the time, I've bee...
-
Finally! Animated Eyes using Seed Xiao RP2040 This is another advance in my studies to enable a more economically viable version for the Monster M...
-
Circuitpython on Seeed XIAO RP2040 Step 1: Unboxing... I2C Not Working?As soon as the card arrived, I installed the firmware version fo...
-
Raspberry Pi Pico with GC9A01 Round Display using Arduino IDE and TFT-eSPI Library This is a work in progress for another one of the artifacts I used in Leonardo Cortez's "Strange Hou...
-
Recreating an 80s TV with Raspberry Pi Recently I built a series of special effects for the scenography of the play "Strange House" by Leon...
-
Talk to Me This project is part of an extensive research on the use of animatronics and interactive objects tha...
-
Back in time! Make a Zoetrope using Arduino I'm working on a series of animated objects for a children's play and decided to build a Zoetrope, t...
-
Alastor Moody Eye using Raspberry Pi Pico, CircuitPython and Round Display GC9A01 I am developing a series of objects for a children's play about fear and terror. And then I got insp...
-
The Crazy Pots Game At some point on the internet I came across someone who had made a game like that, but unfortunately...
-
Arduino Mastermind Game I created this little game as a hobby for my children during the Covid-19 quarantine. I had already ...
-
Raspberry Pi Pico With I2C Oled Display and CircuitPython This is my first experience using this little board from Raspberry Pi Foundation.I preferred to inst...
-
Raspberry Pi Pico and TFT ILI9341 with Circuit Python I decided to write another tutorial on the Raspberry Pi Pico, mainly because the card is very recent...
-
Arduino Minesweeeper It was then that I found the works of Rachit Belwariar, on the page https://www.geeksforgeeks.org/cp...
-
Homeassistant in Beaglebone Black With Debian 11 Intro: The primary objective of my project was to give some use to the Beaglebone Black that has bee...
-
The Adventures of Porting Circuitpython to Wio RP2040 I have been developing some electronic props solutions for Escape Rooms. And periodically I usually ...
-
Simple Electronics to Escape Room Owners - Using a bunch of voltmeters with PCA9685 This is a preliminary study for an Escape Room game. My main goal was to add as many analog displays...
-
Simple Electronics to Escape Room Owners - First Chapter I've been developing puzzles and artifacts for Escape Room since 2018 and most of the time, I've bee...
-
Finally! Animated Eyes using Seed Xiao RP2040 This is another advance in my studies to enable a more economically viable version for the Monster M...
-
Circuitpython on Seeed XIAO RP2040 Step 1: Unboxing... I2C Not Working?As soon as the card arrived, I installed the firmware version fo...
-
-
-
Modifying a Hotplate to a Reflow Solder Station
753 1 5 -
MPL3115A2 Barometric Pressure, Altitude, and Temperature Sensor
405 0 1 -
-
Nintendo 64DD Replacement Shell
352 0 2 -
V2 Commodore AMIGA USB-C Power Sink Delivery High Efficiency Supply Triple Output 5V ±12V OLED display ATARI compatible shark 100W
974 4 2 -
How to measure weight with Load Cell and HX711
640 0 3