![]() |
Autodesk Fusion 360Autodesk
|
|
![]() |
Arduino Web Editor |
|
![]() |
3D Printer (generic) |
Weather gadget with clock through ESP8266
Introduction
Imagine a device that combines technology with an elegant design, bringing functionality and style to any desk. We have developed our latest project: an innovative gadget that not only displays the time accurately, but also monitors the temperature and humidity of the environment and can send and receive important information through an internet connection. It is composed of an ESP8266, allowing you to develop countless projects with the Internet of Things.
In this article, we will introduce the creation process of this fascinating device and present the structure of the control board provided by PCBWay to carry out communication with the internet and control of the TFT display. In addition, we will explore the 3D modeling process that brought to life the sophisticated design, combining aesthetics and functionality to transform a simple gadget into a piece of technological decoration.
Below we present the complete structure of the weather and climate gadget with the ESP8266.
What will you learn at the end of this article?
Creation of the communication system between the control board and the TFT display,
Reading the BMP280 sensor,
Structure of the gadget's 3D model,
And much more.
Do you want to have access to all the files for this project? Go to the PCBWay website now and download the 3D model and the electronic board files.
Now, let's get started and understand how this project works.
Development of time and weather gadget with ESP8266
The weather gadget is an essential device to have on any desk, because in addition to displaying the time, date and weather conditions, you can change the firmware and communicate with various APIs on the internet through the ESP8266.
The framework that was created is open source and you can develop whatever you want. Below we have the project structure with an exploded view of each part that makes it up.
As you can see, the gadget is made up of the following parts:
- Front enclosure,
- Cover enclosure,
- Printed circuit board with ESP8266,
- TFT display,
- And power battery.
First, let's understand the structure of the electronic circuit on the printed circuit board.
Electronic Schematic of the Printed Circuit Board
The 3D electronic design of the printed circuit board is shown in the figure below. As you can see, it consists of an ESP8266 and several terminals that will be used to connect the board directly to a TFT display
Below we have the electronic structure of each circuit block that forms the control board.
The printed circuit board is made up of 5 electronic circuit blocks. Below we have each of them.
- TX and RX pins for code transfer,
- ON/OFF LED circuit to signal the board is powered,
- Circuit for configuring the buttons for programming modes,
- ESP8266 circuit, and
- TFT display connection pins.
The combination of all these circuits ensures the process of transferring code from the computer to the ESP8266. This process is performed using the two Flash and Reset buttons.
They are responsible for configuring the ESP8266 for programming mode. The user must use a USB-SERIAL converter and transfer the code via the RX and TX pins.
After transferring the code, the ESP8266 will start executing the application and send the information to the TFT display. It can be used to display the time, weather conditions and other parameters of interest that can be obtained from various APIs on the internet.
After transferring the code, the ESP8266 will start executing the application and send the information to the TFT display. It can be used to display the time, weather conditions and other parameters of interest that can be obtained from various APIs on the internet.
After assembling the structure of the electronic board with the display, we created 3D modeling of the electronic housing of the time and weather gadget.
Development of the electronic housing of the IoT gadget
In the development of a compact electronic enclosure project, the choice of design and mounting methods is crucial to ensure functionality and efficiency. In this project, we used Fusion 360 to model a plastic enclosure, which was subsequently 3D printed. The main concern was to create a compact structure without compromising the integrity and functionality of the control circuit board.
To achieve this compactness, we opted for an innovative approach to mounting the printed circuit board. Instead of traditional methods, we designed a grid-like structure. This grid is responsible for securely and efficiently fixing and restricting the movement of the board. To ensure a simple and solid assembly, we used the snap fit technique, which allows the grid to be attached to the internal structure of the electronic enclosure without the need for additional tools.
See the structure presented below.
This solution not only optimizes the internal space but also facilitates the assembly and maintenance of the device, demonstrating a practical and creative application of 3D printing technology and computer-aided design. Below, we have the printed circuito board fixed with aid of grids.
After building the 3D model and developing the electronic board, we developed the project's control code.
Development of Programming Logic
Below we have the developed programming logic. For this project we used the TFT Display TFT IPS 1.3 SPI 240X240 RGB display.
#include <Arduino.h> #include <Adafruit_GFX.h> // Core graphics library #include <Adafruit_I2CDevice.h> #include <Adafruit_ST7789.h> // Hardware-specific library for ST7789 #include <NTPClient.h> #include <WiFiUdp.h> #include <WiFi.h> #include <Wire.h> #include <Adafruit_BMP280.h> #include <SPI.h> // Arduino SPI library #define TFT_MOSI 23 // SDA Pin on ESP32 wemos D7 #define TFT_SCLK 18 // SCL Pin on ESP32 wemos D5 #define TFT_CS 15 // Chip select control pin D16 #define TFT_DC 2 // Data Command control pin #define TFT_RST 4 // Reset pin (could connect to RST pin) #define BMP_SDA 21 //Definição dos pinos I2C #define BMP_SCL 22 Adafruit_BMP280 bmp; // Replace with your network credentials const char* ssid = "brisa-2185220"; const char* password = "bruxxf6d"; // Define NTP Client to get time WiFiUDP ntpUDP; NTPClient timeClient(ntpUDP); // Variables to save date and time String formattedDate; String dayStamp; String timeStamp; // Initialize Adafruit ST7789 TFT library Adafruit_ST7789 tft = Adafruit_ST7789(TFT_CS, TFT_DC, TFT_RST); float p = 3.1415926; void tftPrintTest() { tft.setTextWrap(false); tft.fillScreen(ST77XX_BLACK); tft.setCursor(0, 30); tft.setTextColor(ST77XX_RED); tft.setTextSize(1); tft.println("Diego Moreira"); tft.setTextColor(ST77XX_YELLOW); tft.setTextSize(2); tft.println("Diego Moreira"); tft.setTextColor(ST77XX_GREEN); tft.setTextSize(3); tft.println("Diego Moreira"); tft.setTextColor(ST77XX_BLUE); tft.setTextSize(5); tft.print(1234.567); delay(1500); tft.setCursor(0, 0); tft.fillScreen(ST77XX_BLACK); tft.setTextColor(ST77XX_WHITE); tft.setTextSize(0); tft.println("Hello World!"); tft.setTextSize(1); tft.setTextColor(ST77XX_GREEN); tft.print(p, 6); tft.println("Umidade"); tft.println(" "); tft.print(8675309, HEX); // print 8,675,309 out in HEX! tft.println(" Print HEX!"); tft.println(" "); tft.setTextColor(ST77XX_WHITE); tft.println("Sketch has been"); tft.println("running for: "); tft.setTextColor(ST77XX_MAGENTA); tft.print(millis() / 1000); tft.setTextColor(ST77XX_WHITE); tft.print(" seconds."); } void setup(void) { Serial.begin(115200); WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); setRotation(3); } Wire.begin(); // Print local IP address and start web server Serial.println(""); Serial.println("WiFi connected."); Serial.println("IP address: "); Serial.println(WiFi.localIP()); // Initialize a NTPClient to get time timeClient.begin(); // Set offset time in seconds to adjust for your timezone, for example:// GMT +1 = 3600// GMT +8 = 28800// GMT -1 = -3600// GMT 0 = 0 timeClient.setTimeOffset(-10800); delay(1000); tft.init(240, 240, SPI_MODE2); // Init ST7789 display 135x240 pixel tft.setRotation(4); tft.fillScreen(ST77XX_BLACK); //if (!bmp.begin(0x76)) { //Tenta iniciar o sensor//Serial.println("Sensor não encontrado");//while(1);//} } void loop() { tft.setCursor(55, 15); tft.fillScreen(ST77XX_BLACK); tft.drawLine(0, 0, 239, 0, ST77XX_YELLOW); tft.drawLine(0, 0, 0, 239, ST77XX_YELLOW); tft.drawLine(0, 239, 239, 239, ST77XX_YELLOW); tft.drawLine(239, 0, 239, 239, ST77XX_YELLOW); tft.setTextColor(ST77XX_WHITE); tft.setTextSize(3); tft.println("Weather"); tft.setCursor(40, 45); tft.setTextSize(2); tft.println("Station"); tft.drawLine(20, 75, 220, 75, ST77XX_YELLOW); while(!timeClient.update()) { timeClient.forceUpdate(); } // The formattedDate comes with the following format:// 2018-05-28T16:00:13Z// We need to extract date and time formattedDate = timeClient.getFormattedDate(); Serial.println(formattedDate); // Extract dateint splitT = formattedDate.indexOf("T"); dayStamp = formattedDate.substring(0, splitT); Serial.print("DATE: "); Serial.println(dayStamp); // Extract time timeStamp = formattedDate.substring(splitT+1, formattedDate.length()-1); Serial.print("HOUR: "); Serial.println(timeStamp); //Apresentar Data tft.setTextSize(2); tft.setCursor(60, 95); tft.println(dayStamp); tft.setCursor(155, 95); //Apresentar hora tft.setTextSize(2); tft.setCursor(65, 120); tft.println(timeStamp); tft.setCursor(162, 120); tft.println("h"); tft.drawLine(20, 155, 220, 155, ST77XX_YELLOW); int temp = bmp.readTemperature(); int pres = (bmp.readPressure()/1000); //Apresentar hora tft.setTextSize(2); tft.setCursor(35, 175); tft.println("Temp:"); tft.setCursor(162, 175); tft.println("C"); //Apresentar hora tft.setTextSize(2); tft.setCursor(120, 175); tft.println(temp); //Apresentar hora tft.setTextSize(2); tft.setCursor(35, 200); tft.println("Pres:"); tft.setCursor(162, 200); tft.println("kPa"); //Apresentar hora tft.setTextSize(2); tft.setCursor(120, 200); tft.println(pres); delay(3000); }
The code above is responsible for performing the following actions:
- Reading the date and time from an NTP server;
- Reading the sensors;
- Displaying the date, time, temperature and humidity information on the TFT display screen.
The code above was created to read and display this information every 3 seconds.
Using the code above, it was possible to program the device and put it into operation. Below we will show a video showing the assembly and complete operation of the device.
Project Construction Result and Final Thoughts
The project of the gadget that displays date, time, temperature and humidity using the ESP8266 successfully achieved the proposed objectives. The device was assembled according to the step-by-step instructions presented in the video, clearly demonstrating how the system works. The integration of the programming with the display allows for precise and up-to-date viewing of the information received from the internet.
The structure of the gadget, modeled and printed in 3D, contributed significantly to the aesthetic and functional design of the device, showing how customization can add value to electronic projects.
The electronic boards used were manufactured by PCBWay, known for its efficiency and cost-effectiveness. With a cost of only $5 to produce 10 boards, PCBWay stood out as an economical and high-quality solution for manufacturing the boards required for the project.
This project not only exemplifies the practical application of the ESP8266 in the creation of connected devices, but also illustrates how affordable features and advanced technologies can be combined to create innovative and functional solutions. The end result is a compact, efficient, and aesthetically pleasing gadget that can serve as inspiration for future electronics and 3D modeling projects.
Acknowledgments
We would like to thank PCBWAY for supportting the creation of this project and made some units available for you to earn for free and receive 5 units at your home. To receive them, access this link, create an account on the website and receive coupons for you to win right now.
#include <Arduino.h>
#include <Adafruit_GFX.h> // Core graphics library
#include <Adafruit_I2CDevice.h>
#include <Adafruit_ST7789.h> // Hardware-specific library for ST7789
#include <NTPClient.h>
#include <WiFiUdp.h>
#include <WiFi.h>
//#include <ESP8266WiFi.h>
#include <Wire.h>
#include <Adafruit_BMP280.h>
#include <SPI.h> // Arduino SPI library
#define TFT_MOSI 23 // SDA Pin on ESP32 wemos D7
#define TFT_SCLK 18 // SCL Pin on ESP32 wemos D5
#define TFT_CS 15 // Chip select control pin D16
#define TFT_DC 2 // Data Command control pin
#define TFT_RST 4 // Reset pin (could connect to RST pin)
#define BMP_SDA 21 //Definição dos pinos I2C
#define BMP_SCL 22
Adafruit_BMP280 bmp;
// Replace with your network credentials
const char* ssid = "brisa-2185220";
const char* password = "bruxxf6d";
// Define NTP Client to get time
WiFiUDP ntpUDP;
NTPClient timeClient(ntpUDP);
// Variables to save date and time
String formattedDate;
String dayStamp;
String timeStamp;
// Initialize Adafruit ST7789 TFT library
Adafruit_ST7789 tft = Adafruit_ST7789(TFT_CS, TFT_DC, TFT_RST);
float p = 3.1415926;
void tftPrintTest() {
tft.setTextWrap(false);
tft.fillScreen(ST77XX_BLACK);
tft.setCursor(0, 30);
tft.setTextColor(ST77XX_RED);
tft.setTextSize(1);
tft.println("Diego Moreira");
tft.setTextColor(ST77XX_YELLOW);
tft.setTextSize(2);
tft.println("Diego Moreira");
tft.setTextColor(ST77XX_GREEN);
tft.setTextSize(3);
tft.println("Diego Moreira");
tft.setTextColor(ST77XX_BLUE);
tft.setTextSize(5);
tft.print(1234.567);
delay(1500);
tft.setCursor(0, 0);
tft.fillScreen(ST77XX_BLACK);
tft.setTextColor(ST77XX_WHITE);
tft.setTextSize(0);
tft.println("Hello World!");
tft.setTextSize(1);
tft.setTextColor(ST77XX_GREEN);
tft.print(p, 6);
tft.println("Umidade");
tft.println(" ");
tft.print(8675309, HEX); // print 8,675,309 out in HEX!
tft.println(" Print HEX!");
tft.println(" ");
tft.setTextColor(ST77XX_WHITE);
tft.println("Sketch has been");
tft.println("running for: ");
tft.setTextColor(ST77XX_MAGENTA);
tft.print(millis() / 1000);
tft.setTextColor(ST77XX_WHITE);
tft.print(" seconds.");
}
void setup(void)
{
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
setRotation(3);
}
Wire.begin();
// Print local IP address and start web server
Serial.println("");
Serial.println("WiFi connected.");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
// Initialize a NTPClient to get time
timeClient.begin();
// Set offset time in seconds to adjust for your timezone, for example:
// GMT +1 = 3600
// GMT +8 = 28800
// GMT -1 = -3600
// GMT 0 = 0
timeClient.setTimeOffset(-10800);
delay(1000);
tft.init(240, 240, SPI_MODE2); // Init ST7789 display 135x240 pixel
tft.setRotation(4);
tft.fillScreen(ST77XX_BLACK);
//if (!bmp.begin(0x76)) { //Tenta iniciar o sensor
//Serial.println("Sensor não encontrado");
//while(1);
//}
}
void loop()
{
tft.setCursor(55, 15);
tft.fillScreen(ST77XX_BLACK);
tft.drawLine(0, 0, 239, 0, ST77XX_YELLOW);
tft.drawLine(0, 0, 0, 239, ST77XX_YELLOW);
tft.drawLine(0, 239, 239, 239, ST77XX_YELLOW);
tft.drawLine(239, 0, 239, 239, ST77XX_YELLOW);
tft.setTextColor(ST77XX_WHITE);
tft.setTextSize(3);
tft.println("Estacao");
tft.setCursor(40, 45);
tft.setTextSize(2);
tft.println("Meteorologica");
tft.drawLine(20, 75, 220, 75, ST77XX_YELLOW);
while(!timeClient.update())
{
timeClient.forceUpdate();
}
// The formattedDate comes with the following format:
// 2018-05-28T16:00:13Z
// We need to extract date and time
formattedDate = timeClient.getFormattedDate();
Serial.println(formattedDate);
// Extract date
int splitT = formattedDate.indexOf("T");
dayStamp = formattedDate.substring(0, splitT);
Serial.print("DATE: ");
Serial.println(dayStamp);
// Extract time
timeStamp = formattedDate.substring(splitT+1, formattedDate.length()-1);
Serial.print("HOUR: ");
Serial.println(timeStamp);
//Apresentar Data
tft.setTextSize(2);
tft.setCursor(60, 95);
tft.println(dayStamp);
tft.setCursor(155, 95);
//Apresentar hora
tft.setTextSize(2);
tft.setCursor(65, 120);
tft.println(timeStamp);
tft.setCursor(162, 120);
tft.println("h");
tft.drawLine(20, 155, 220, 155, ST77XX_YELLOW);
int temp = bmp.readTemperature();
int pres = (bmp.readPressure()/1000);
//Apresentar hora
tft.setTextSize(2);
tft.setCursor(35, 175);
tft.println("Temp:");
tft.setCursor(162, 175);
tft.println("C");
//Apresentar hora
tft.setTextSize(2);
tft.setCursor(120, 175);
tft.println(temp);
//Apresentar hora
tft.setTextSize(2);
tft.setCursor(35, 200);
tft.println("Pres:");
tft.setCursor(162, 200);
tft.println("kPa");
//Apresentar hora
tft.setTextSize(2);
tft.setCursor(120, 200);
tft.println(pres);
delay(3000);
}
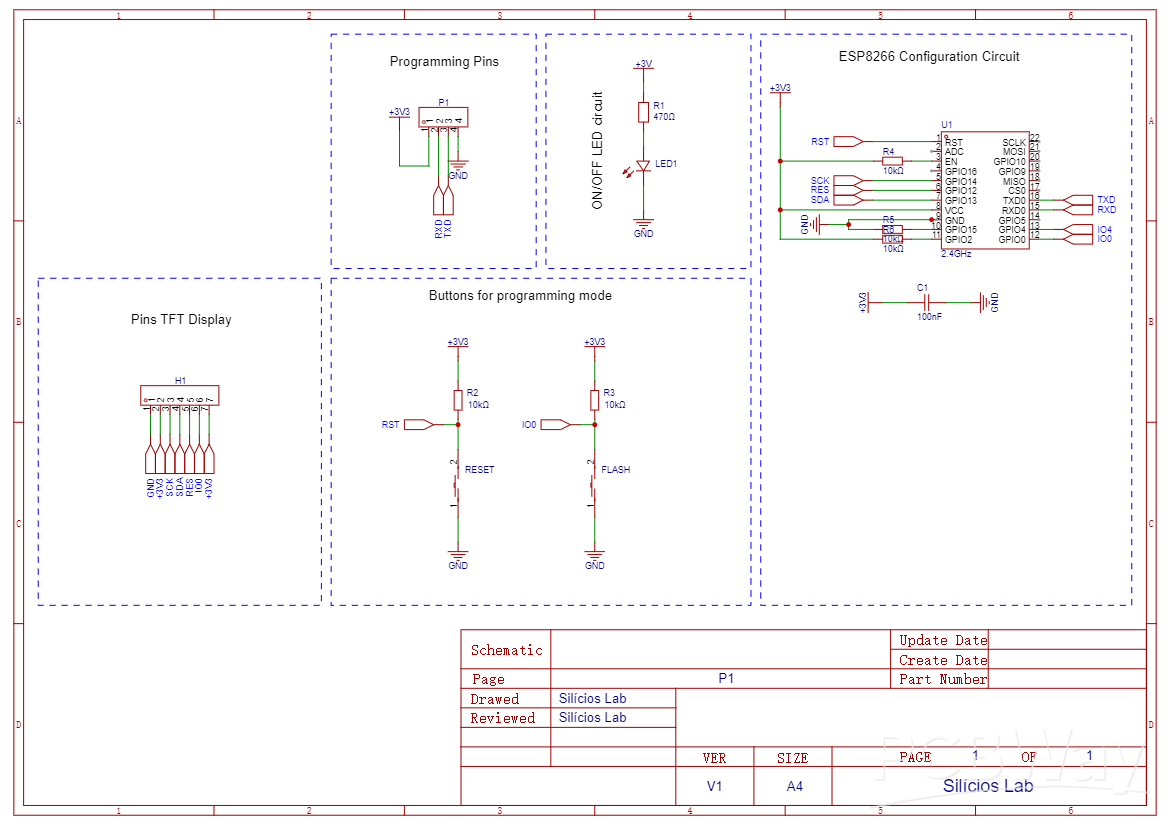
Weather gadget with clock through ESP8266
*PCBWay community is a shared platform and we are not responsible for any design issues.
- Comments(0)
- Likes(0)
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by Silícios Lab silicioslab
-
Electronic Enclosure applied for electronic projects IntroductionWhen designing electronics, the enclosure plays a crucial role that is often overlooked....
-
IoT Indoor system with ESP32 to monitor Temperature, Humidity, Pressure, and Air Quality IntroductionAir quality, temperature, humidity and pressure are essential elements to ensure healthy...
-
WS2812B RGB LED Controller with ESP8266 via WiFi IntroductionWS2812b addressable RGB LEDs are devices widely used in lighting projects. They are foun...
-
Electronic Board for Cutting Electrical Power to Devices and Machines IntroductionAn energy saving system for cutting electrical energy in machines is a fundamental piece...
-
PCB Board Home Automation with ESP8266 IntroductionThe incorporation of the ESP8266 module into home automation represents a significant ad...
-
Dedicated Control Board for Mobile Robots with Wheels IntroductionFor a long time we developed several prototypes and teaching kits of mobile robots and w...
-
Traffic turn signal for bicycles IntroductionDoes every project with electronic logic need a Microcontroller or Arduino to be develop...
-
Mini Arduino with ATTINY85 Do you know the ATTINY85 microcontroller? This article has news and a gift for you. Many people deve...
-
Christmas Tree The tree used to signal light of Christmas.
-
Payment PCB for machines and services IntroductionIn many commercial establishments, hospitals and other places, there are video game equi...
-
Relay High Power Printed Circuit Board IntroductionEfficient management of electrical loads is essential for optimizing performance and saf...
-
Weather gadget with clock through ESP8266 IntroductionImagine a device that combines technology with an elegant design, bringing functionality...
-
ESP32 MPU6050 Monitor IntroductionVarious industrial equipment is essential for the adequate production of products, parts...
-
Digital Speedometer for Bicycles IntroductionCycling, increasingly popular both as a recreational activity and as a means of transpor...
-
Arduino-based development board with extra features IntroductionArduino is an excellent tool for anyone who wants to develop prototypes. The board has a...
-
How to develop low-energy devices powered by batteries? IntroductionIn recent years, there has been a major advance in the area of embedded systems through ...
-
Activating loads with relay via WiFi with ESP8266 IntroductionDo you want an electronic board for activating loads via WiFi that is safe against surge...
-
Hospital Water Tank Monitoring via the Internet IntroductionOne of the elements of great importance for the functioning of hospitals is water. It is...