![]() |
Blynk App |
GPS Module Based Tracking Device Pcb
ESP32 GPS vehicle tracker using NEO 6M GPS module and Arduino IDE. With the help of this GPS tracker, we will be able to track vehicles from anywhere around the world. We will also show GPS location coordinates on an OLED display connected with the ESP32 board. The location of the vehicle will be displayed in the form of longitude and latitude. To make our vehicle tracking system even more practical, users can track the location of vehicles on the Blynk app. In other words, it becomes extremely handy to track the location of vehicles from anywhere through a mobile application. We will program our ESP32 board using Arduino IDE.
NEO 6M GPS Module
We will be using NEO 6M GPS module to help us track the vehicle via GPS. It consists of high performance u-blox 6 positioning engine. Measuring 16 x 12.2 x 2.4 mm, its compact architecture along with its low power consumption makes it a good choice for IoT projects. Overall it is a good cost effective GPS receiver. The table below shows some key features of the module.
TypeGPSSupply2.7 V-3.6 VFeaturesRTC Crystal and External Interrupt/Wake upInterfaceUART, SPI, USB and DDC
For more information regarding the NEO-6M module refer to its datasheet
Pinout of NEO 6M Module
The diagram below shows the pin out of the NEO 6M module. It consists of 4 pins named GND, TX, RX and VCC.
the NEO-6M GPS module also has 4 terminals which we will connect with the ESP32 board. As the GPS module requires an operating voltage in the range of 2.7-3.6V hence we will connect the VCC terminal with 3.3V which will be in common with the ESP32 board. The TX (transmitter) terminal of the GPS module will be connected with the RX2 pin of the ESP32 for communication. Likewise, the RX (receiver) terminal of the GPS module will be connected with the TX2 pin of the ESP32.
Search for ‘Blynk’ and install the application.
You will have to create an account to proceed further. You can also log in with your Facebook account if you possess that.
Creating Project
After you have successfully signed in the following window will appear. Click ‘New Project.’
Now specify the name of your project, device and connection type. After that press the ‘Create’ button.
You will receive a notification regarding your Authorization token. This can be accessed from the email account you signed in with and also through Project Settings.
Now the Blynk canvas will open. Press on the screen. The widget box will appear. Press the ‘Map’ widget. You can view information
regarding it by pressing the icon present in the far right.
Now click on this widget and the map settings appear. Choose the virtual pin V0 as an input pin. Press ‘OK’.
These are the map settings which we are using.
After you alter the settings of the map the canvas will look something like this:
Installing Required Arduino Libraries
We will use Arduino IDE to program our ESP32 development board. Make sure your Arduino IDE already has the ESP32 plugin installed. To program our ESP32 board for this GPS tracker we will be required to install four libraries: BlynkSimpleEsp32.h, TinyGPS++.h, Adafruit_SSD1306.h and Adafruit_GFX.h
Installing Blynk Library
To use the Blynk app with our ESP32 board, we would have to install its library.
To download the library, click here. Click on ‘Code’ and then ‘Download Zip’.
You have to go to Sketch > Include Library > Add .zip Library inside the IDE to add the library as well. After installation of the library, restart your IDE.
Installing TinyGPS++ Library
To make our project easier we will install the TinyGPS++ library to easily handle the receiving data from the GPS module. To download the library, click here. Click on ‘Code’ and then ‘Download Zip’.
You have to go to Sketch > Include Library > Add .zip Library inside the IDE to add the library as well.
Installing OLED Display Libraries
To use the OLED display in our project, we have to install the Adafruit SSD 1306 library and Adafruit GFX library in Arduino IDE. Follow the steps below to successfully install them.
Open Arduino IDE and click on Sketch > Library > Manage Libraries
The following window will open up.
How the Code Works?
In this section let us see how the code works.
Including Libraries
Firstly, we will include all the following libraries which are required for this project. The WiFi.h and will be used to connect to the wireless network. The Wire.h will allow us to communicate through the I2C protocol. Whereas the other libraries are the ones which we previously installed and are required for the proper functionality of the OLED display, the GPS module and the Blynk application.
#include <TinyGPS++.h>
#include <HardwareSerial.h>
#include <WiFi.h>
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <BlynkSimpleEsp32.h>
Defining OLED Display
We will first define the width and height of our OLED display in pixels. We are using a 128×64 display hence the width will be 128 and the height will be 64.
#define SCREEN_WIDTH 128
#define SCREEN_HEIGHT 64
Next, we will initialize the display by creating an object of Adafruit_SSD1306 and specifying the width, height, I2C instance (&Wire), and -1 as parameters inside it.’ -1′ specifies that the OLED display which we are using does not have a RESET pin. If you are using the RESET pin then specify the GPIO through which you are connecting it with your development board.
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1);
Defining Variables
Next we will define some string and float variable that we will use later on in the code. These will save the latitude and longitude values of the vehicle.
float latitude , longitude;
String latitude_string , longitiude_string;
Setting Network Credentials
Now, we need to define the SSID name and the password. Following lines set the name of SSID and password. You can replace the SSID and password to your own network credentials.
const char *ssid = "Your_SSID";
const char *pass = "Your_Password";
Defining Authorization key
We will define the authorization key which was emailed to us through Blynk in our account through which we logged in it. Keep this key safe with you for security concerns.
char auth[] = "ec8EX0fnX1y78Oo6pO8lp8Of_AWUd***";
Creating Instances
Create the following instances for the widget map, wifi client, TinyGPSPlus library and HardwareSerial.
WidgetMap myMap(V0);
WiFiClient client;
TinyGPSPlus gps;
HardwareSerial SerialGPS(2);
setup()
Inside the setup() function, we will open a serial connection at a baud rate of 115200.
Serial.begin(115200);
First, we will clear the buffer by using clearDisplay() on the Adafruit_SSD1306 object. Next, we will control the colour of the text by using the setTextColor() function and passing WHITE as an argument. We will set the size of the text using setTextSize() and pass the size as a parameter inside it. We have set the font size as default which is 1.
We will use the setCursor() function to denote the x and the y axis position from where the text should start. Then by using print() we will pass the text which we want to display on the OLED. We will set the cursor again at different positions to display the all the values correctly. We will call the display() function on the display object so that the text displays on the OLED.
#include <TinyGPS++.h>
#include <HardwareSerial.h>
#include <WiFi.h>
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <BlynkSimpleEsp32.h>
float latitude , longitude;
String latitude_string , longitiude_string;
#define SCREEN_WIDTH 128
#define SCREEN_HEIGHT 64
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1);
const char *ssid = "Your_SSID";
const char *pass = "Your_Password";
char auth[] = "ec8EX0fnX1y78Oo6pO8lp8Of_AWUd***";
WidgetMap myMap(V0);
WiFiClient client;
TinyGPSPlus gps;
HardwareSerial SerialGPS(2);
void setup()
{
Serial.begin(115200);
if(!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
for(;;);
}
WiFi.begin(ssid, pass);
while (WiFi.status() != WL_CONNECTED)
{
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected!");
SerialGPS.begin(9600, SERIAL_8N1, 16, 17);
Blynk.begin(auth, ssid, pass);
Blynk.virtualWrite(V0, "clr");
}
void loop()
{
while (SerialGPS.available() > 0) {
if (gps.encode(SerialGPS.read()))
{
if (gps.location.isValid())
{
latitude = gps.location.lat();
latitude_string = String(latitude , 6);
longitude = gps.location.lng();
longitiude_string = String(longitude , 6);
Serial.print("Latitude = ");
Serial.println(latitude_string);
Serial.print("Longitude = ");
Serial.println(longitiude_string);
display.clearDisplay();
display.setTextSize(1);
display.setTextColor(WHITE);
display.setCursor(0, 20);
display.println("Latitude: ");
display.setCursor(45, 20);
display.print(latitude_string);
display.setCursor(0, 40);
display.print("Longitude: ");
display.setCursor(45, 40);
display.print(longitiude_string);
Blynk.virtualWrite(V0, 1, latitude, longitude, "Location");
display.display();
}
delay(1000);
Serial.println();
}
}
Blynk.run();
}
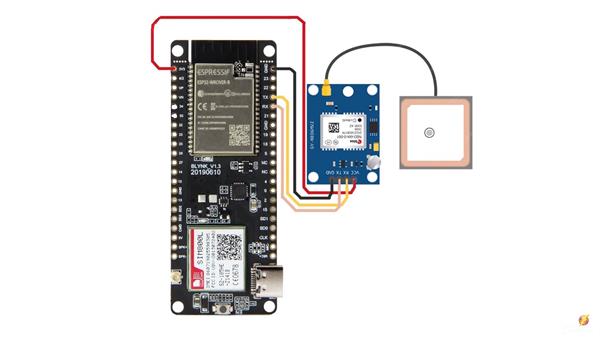
GPS Module Based Tracking Device Pcb
- Comments(0)
- Likes(0)
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by electronicguru0007
-
How to make an alarm clock with pic microcontroller he five push buttons will act as an input for setting the alarm for the required time. So one end of...
-
How to make RMS to DC Converter using IC AD736 A True-RMS or TRMS is a type of converter which converts RMS value to equivalent DC value. Here in t...
-
STM32 SPI Communcation and Data Sent SPI in STM32F103C8Comparing SPI bus in Arduino & STM32F103C8 Blue Pill board, STM32 has 2 SPI bu...
-
How to Communicate Arduinos via RS-485 What project will you develop?The project consists of 3 Arduino's. We have an Arduino UNO, a Nano, a...
-
PIC16F877A Temperature and Humidity Measurement Board Temperature and Humidity measurement is often useful in many applications like Home Automation, Envi...
-
Diy Buck Converter n the field of DC-DC Converters, A single-ended primary-inductor converter or SEPIC converter is a t...
-
Iot AC Current Measuring System Smart power monitoring is getting increasingly popular to improve energy efficiency in medium/small ...
-
ESP32 Weather Station In this project, we will learn how to create a weather station, which will display reading from a BM...
-
NRF Data Transfer Via 2 Boards There are various wireless communication technologies used in building IoT applications and RF (Radi...
-
Iot patient monitoring system When we are talking about major vital signs of a human body, there are four major parameters that we...
-
Setting up zigbee communication with nodemcu and arduino Zigbee is a popular wireless communication protocol used to transfer a small amount of data with ver...
-
Ac Dimmer Remote PCB The brightness can be controlled using the IR remote of TV, DVD, etc. Dimming Control system using M...
-
Esp32 Home Automation There are relay modules whose electromagnet can be powered by 5V and with 3.3V. Both can be used wit...
-
Lora Communication With Network This was a very simple project and can come in handy for various applications. But what it can't do ...
-
GPS Module Based Tracking Device Pcb ESP32 GPS vehicle tracker using NEO 6M GPS module and Arduino IDE. With the help of this GPS tracker...
-
Traffic Management for Emergency Vehicles using AT89S52 Microcontroller These days’ traffic congestion is the biggest problem of densely populated cities. The project focus...
-
Diy Multimeter Pcb This is a project based on Arduino board which can measureresistance, diode, continuity[H1] , voltag...
-
Live Instagram Followers Pcb ESP8266 is capable of functioning reliably in industrial environments, with an operating temperature...
-
-
-
kmMiniSchield MIDI I/O - IN/OUT/THROUGH MIDI extension for kmMidiMini
117 0 0 -
DIY Laser Power Meter with Arduino
167 0 2 -
-
-
Box & Bolt, 3D Printed Cardboard Crafting Tools
157 0 2 -