|
ESP32-WROOM-32DEspressif Systems
|
x 1 | |
|
G6K-2F-Y3VDCOMRON
|
x 1 | |
![]() |
Mini Breadboard |
x 1 | |
![]() |
269JKL Components Corp.
|
x 1 |
Esp32 Home Automation
There are relay modules whose electromagnet can be powered by 5V and with 3.3V. Both can be used with the ESP32 or ESP8266 – you can either use the VIN pin (that provides 5V) or the 3.3V pin.
Additionally, some come with built-in optocoupler that add an extra “layer” of protection, optically isolating the ESP boards from the relay circuit.
The second set of pins consists of GND, VCC, and JD-VCC pins. The JD-VCC pin powers the electromagnet of the relay. Notice that the module has a jumper cap connecting the VCC and JD-VCC pins; the one shown here is yellow, but yours may be a different color.
With the jumper cap on, the VCC and JD-VCC pins are connected. That means the relay electromagnet is directly powered from the ESP power pin, so the relay module and the ESP circuits are not physically isolated from each other.
Without the jumper cap, you need to provide an independent power source to power up the relay’s electromagnet through the JD-VCC pin. That configuration physically isolates the relays from the ESP with the module’s built-in optocoupler, which prevents damage to the ESP in case of electrical spikes.
Controlling a Relay Module – MicroPython Code (Script)
The code to control a relay with the ESP32 or ESP8266 is as simple as controlling an LED or any other output. In this example, as we’re using a normally open configuration, we need to send a LOW signal to let the current flow, and a HIGH signal to stop the current flow.
boot.py script
def web_page():
if relay.value() == 1:
relay_state = ''
else:
relay_state = 'checked'
html = """<html><head><meta name="viewport" content="width=device-width, initial-scale=1"><style>
body{font-family:Arial; text-align: center; margin: 0px auto; padding-top:30px;}
.switch{position:relative;display:inline-block;width:120px;height:68px}.switch input{display:none}
.slider{position:absolute;top:0;left:0;right:0;bottom:0;background-color:#ccc;border-radius:34px}
.slider:before{position:absolute;content:"";height:52px;width:52px;left:8px;bottom:8px;background-color:#fff;-webkit-transition:.4s;transition:.4s;border-radius:68px}
input:checked+.slider{background-color:#2196F3}
input:checked+.slider:before{-webkit-transform:translateX(52px);-ms-transform:translateX(52px);transform:translateX(52px)}
</style><script>function toggleCheckbox(element) { var xhr = new XMLHttpRequest(); if(element.checked){ xhr.open("GET", "/?relay=on", true); }
else { xhr.open("GET", "/?relay=off", true); } xhr.send(); }</script></head><body>
<h1>ESP Relay Web Server</h1><label class="switch"><input type="checkbox" onchange="toggleCheckbox(this)" %s><span class="slider">
</span></label></body></html>""" % (relay_state)
return html
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind(('', 80))
s.listen(5)
while True:
try:
if gc.mem_free() < 102000:
gc.collect()
conn, addr = s.accept()
conn.settimeout(3.0)
print('Got a connection from %s' % str(addr))
request = conn.recv(1024)
conn.settimeout(None)
request = str(request)
print('Content = %s' % request)
relay_on = request.find('/?relay=on')
relay_off = request.find('/?relay=off')
if relay_on == 6:
print('RELAY ON')
relay.value(0)
if relay_off == 6:
print('RELAY OFF')
relay.value(1)
response = web_page()
conn.send('HTTP/1.1 200 OK\n')
conn.send('Content-Type: text/html\n')
conn.send('Connection: close\n\n')
conn.sendall(response)
conn.close()
except OSError as e:
conn.close()
print('Connection closed')
After making the necessary changes, upload the boot.py and main.py files to your board. Press the EN/RST button and in the Shell you should get the ESP IP address.
Then, open a browser in your local network and type the ESP IP address to get access to the web server.
You should get a web page with a toggle button that allows you to control your relay remotely using your smartphone or your computer.
Insert your network credentials in the following variables:
ssid = 'REPLACE_WITH_YOUR_SSID'
password = 'REPLACE_WITH_YOUR_PASSWORD'
Uncomment one of the following lines accordingly to the board you’re using. By default, it’s set to use the ESP32 GPIO.
# ESP32 GPIO 26
relay = Pin(26, Pin.OUT)
# ESP8266 GPIO 5
#relay = Pin(5, Pin.OUT)
def web_page():
if relay.value() == 1:
relay_state = ''
else:
relay_state = 'checked'
html = """<html><head><meta name="viewport" content="width=device-width, initial-scale=1"><style>
body{font-family:Arial; text-align: center; margin: 0px auto; padding-top:30px;}
.switch{position:relative;display:inline-block;width:120px;height:68px}.switch input{display:none}
.slider{position:absolute;top:0;left:0;right:0;bottom:0;background-color:#ccc;border-radius:34px}
.slider:before{position:absolute;content:"";height:52px;width:52px;left:8px;bottom:8px;background-color:#fff;-webkit-transition:.4s;transition:.4s;border-radius:68px}
input:checked+.slider{background-color:#2196F3}
input:checked+.slider:before{-webkit-transform:translateX(52px);-ms-transform:translateX(52px);transform:translateX(52px)}
</style><script>function toggleCheckbox(element) { var xhr = new XMLHttpRequest(); if(element.checked){ xhr.open("GET", "/?relay=on", true); }
else { xhr.open("GET", "/?relay=off", true); } xhr.send(); }</script></head><body>
<h1>ESP Relay Web Server</h1><label class="switch"><input type="checkbox" onchange="toggleCheckbox(this)" %s><span class="slider">
</span></label></body></html>""" % (relay_state)
return html
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind(('', 80))
s.listen(5)
while True:
try:
if gc.mem_free() < 102000:
gc.collect()
conn, addr = s.accept()
conn.settimeout(3.0)
print('Got a connection from %s' % str(addr))
request = conn.recv(1024)
conn.settimeout(None)
request = str(request)
print('Content = %s' % request)
relay_on = request.find('/?relay=on')
relay_off = request.find('/?relay=off')
if relay_on == 6:
print('RELAY ON')
relay.value(0)
if relay_off == 6:
print('RELAY OFF')
relay.value(1)
response = web_page()
conn.send('HTTP/1.1 200 OK\n')
conn.send('Content-Type: text/html\n')
conn.send('Connection: close\n\n')
conn.sendall(response)
conn.close()
except OSError as e:
conn.close()
print('Connection closed')
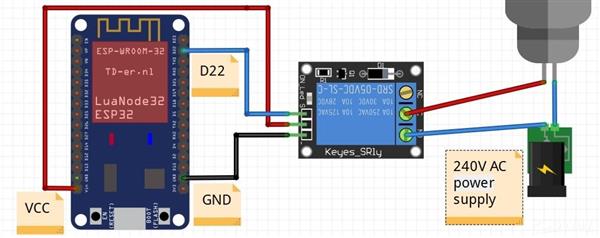
Esp32 Home Automation
- Comments(0)
- Likes(0)
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by electronicguru0007
-
How to make an alarm clock with pic microcontroller he five push buttons will act as an input for setting the alarm for the required time. So one end of...
-
How to make RMS to DC Converter using IC AD736 A True-RMS or TRMS is a type of converter which converts RMS value to equivalent DC value. Here in t...
-
STM32 SPI Communcation and Data Sent SPI in STM32F103C8Comparing SPI bus in Arduino & STM32F103C8 Blue Pill board, STM32 has 2 SPI bu...
-
How to Communicate Arduinos via RS-485 What project will you develop?The project consists of 3 Arduino's. We have an Arduino UNO, a Nano, a...
-
PIC16F877A Temperature and Humidity Measurement Board Temperature and Humidity measurement is often useful in many applications like Home Automation, Envi...
-
Diy Buck Converter n the field of DC-DC Converters, A single-ended primary-inductor converter or SEPIC converter is a t...
-
Iot AC Current Measuring System Smart power monitoring is getting increasingly popular to improve energy efficiency in medium/small ...
-
ESP32 Weather Station In this project, we will learn how to create a weather station, which will display reading from a BM...
-
NRF Data Transfer Via 2 Boards There are various wireless communication technologies used in building IoT applications and RF (Radi...
-
Iot patient monitoring system When we are talking about major vital signs of a human body, there are four major parameters that we...
-
Setting up zigbee communication with nodemcu and arduino Zigbee is a popular wireless communication protocol used to transfer a small amount of data with ver...
-
Ac Dimmer Remote PCB The brightness can be controlled using the IR remote of TV, DVD, etc. Dimming Control system using M...
-
Esp32 Home Automation There are relay modules whose electromagnet can be powered by 5V and with 3.3V. Both can be used wit...
-
Lora Communication With Network This was a very simple project and can come in handy for various applications. But what it can't do ...
-
GPS Module Based Tracking Device Pcb ESP32 GPS vehicle tracker using NEO 6M GPS module and Arduino IDE. With the help of this GPS tracker...
-
Traffic Management for Emergency Vehicles using AT89S52 Microcontroller These days’ traffic congestion is the biggest problem of densely populated cities. The project focus...
-
Diy Multimeter Pcb This is a project based on Arduino board which can measureresistance, diode, continuity[H1] , voltag...
-
Live Instagram Followers Pcb ESP8266 is capable of functioning reliably in industrial environments, with an operating temperature...
-
-
-
kmMiniSchield MIDI I/O - IN/OUT/THROUGH MIDI extension for kmMidiMini
117 0 0 -
DIY Laser Power Meter with Arduino
166 0 2 -
-
-
Box & Bolt, 3D Printed Cardboard Crafting Tools
156 0 2 -