|
ESP8266EXESPRESSIF
|
x 1 | |
![]() |
Arduino Nano V3 |
x 1 |
![]() |
Arduino Web Editor |
Home Automation With esp8266
IoT Based Home Automation Project using Google Firebase & NodeMCU ESP8266. One of the most common & popular hobby projects you will come across the internet is Home Automation Project. By Home Automation we mean controlling home appliances without a manual switch. When connected with the Internet, home devices are an important constituent of the Internet of Things (“IoT”) Application.
Earlier we learned about Home automation using Blynk Application, WebServer, Amazon Alexa & Arduino IoT cloud and also AWS IoT Core. But in this project we will use Google Firebase. Google Firebase is a Google-backed application development software used for creating, managing,and modifying data generated from any android/IOS application, web services, IoT sensors & Hardware. To learn more about the Google Firebase Console, you can read the official Google Firebase Documentation from Google Firebase.
We will design a Home Automation Android Application using MIT APP Inventor. Using the Firebase Host & Authentication Key, we will connect the Android Application with Google Firebase. We will then send the ON/OFF command as 1/0 from App. The data from the App is sent to Google Firebase. Now the Google Firebase data is updated to NodeMCU Board via Internet Connection. Thus the digital GPIO Pins becomes high & low turning ON/OFF the appliances connected to Relay.
Circuit Diagram & Connection
The circuit diagram for Google Firebase Based Home Automation using ESP8266 is given below. Using this circuit diagram you can assemble the circuit on Breadboard using 4 channel Relay and NodeMCU Board.
Home Automation PCB Gerber File & PCB Ordering Online
If you don’t want to assemble the circuit on breadboard and you want PCB for the project, then here is the PCB for you. The PCB Board for the Home Automation Project is designed using EasyEDA online Circuit Schematics & PCB designing tool.
Setting up Google Firebase Console Database
Now the main thing that we need to do is Setting up Google Firebase Console Database. Once the setup is done, we can then Send Real-Time Data from Android Application to Google Firebase.
But I won’t be explaining here how you can set up the Google Firebase Console Database because I have already explained the entire process in the previous tutorial. You can check the following tutorial to learn how to do the setup.
Getting Started with Google Firebase with ESP8266
You need Firebase Host & Authentication Token. These both parameters are required in Arduino Code as well as Firebase App.
Designing & Setting Up Android Application
Now we need to design an Android Application that can send data to Google Firebase. The easiest way to design an Android Application is using MIT App Inventor. The MIT APP Inventor lets you develop applications for Android phones using a web browser and either the connected phone or emulator. The App Inventor servers store your work and help you keep track of your projects.
I have simply designed an Interface for Home Automation project using Google Firebase. The App has 4 pairs on ON/OFF Switch to Send the 0 and 1 command to Google Firebase.
Adding Firebase & JSON Library to Arduino IDE
1. ArduinoJSON Library
So first go to the library manager and search for “JSON” & install the library as shown in the figure below.
Note: The latest JSON library might not work with the code. So you may need to downgrade the library to version v5.13.5
2. Google FirebaseExtended Library
Uploading Your Lua Script:
Follow these steps to send code to your ESP8266:
Connect your FTDI programmer and select your FTDI COM Port.
Set baud rate at 9600
Select NodeMCU+MicroPtyhon tab
Copy the your Lua script into ESPlorer and save your file with the name “init.lua”.
Upload to the ESP and Run on ESP.
Don’t forgot to change the SSID and Password to your Home WiFi Network.
Now you need to install Google Firebase library as well. So, download the library from below link and add it to Library folder after extraction.
#include <ESP8266WiFi.h>
#include <FirebaseArduino.h>
#define FIREBASE_HOST "**********" // Your Firebase Project URL
#define FIREBASE_AUTH "************" // Your Firebase Database Secret
#define WIFI_SSID "**************" // your WiFi SSID
#define WIFI_PASSWORD "********" // your WiFi PASSWORD
#define Relay1 12 //D6
int value1;
#define Relay2 14 //D2
int value2;
#define Relay3 4 //D1
int value3;
#define Relay4 5 //D5
int value4;
void setup()
{
Serial.begin(115200);
pinMode(Relay1,OUTPUT);
pinMode(Relay2,OUTPUT);
pinMode(Relay3,OUTPUT);
pinMode(Relay4,OUTPUT);
digitalWrite(Relay1,HIGH);
digitalWrite(Relay2,HIGH);
digitalWrite(Relay3,HIGH);
digitalWrite(Relay4,HIGH);
WiFi.begin(WIFI_SSID,WIFI_PASSWORD);
Serial.print("connecting");
while (WiFi.status()!=WL_CONNECTED)
{
Serial.print(".");
delay(500);
}
Serial.println();
Serial.print("connected:");
Serial.println(WiFi.localIP());
Firebase.begin(FIREBASE_HOST);
Firebase.setInt("S1",0);
Firebase.setInt("S2",0);
Firebase.setInt("S3",0);
Firebase.setInt("S4",0);
}
void firebasereconnect()
{
Serial.println("Trying to reconnect");
Firebase.begin(FIREBASE_HOST);
}
void loop()
{
if (Firebase.failed())
{
Serial.print("setting number failed:");
Serial.println(Firebase.error());
firebasereconnect();
return;
}
value1=Firebase.getString("S1").toInt(); //Reading the Status of Variable 1 from the firebase
if(value1==1)
{
digitalWrite(Relay1,LOW);
Serial.println("Relay 1 ON");
}
else if(value1==0)
{
digitalWrite(Relay1,HIGH);
Serial.println("Relay 1 OFF");
}
value2=Firebase.getString("S2").toInt(); //Reading the Status of Variable 2 from the firebase
if(value2==1)
{
digitalWrite(Relay2,LOW);
Serial.println("Relay 2 ON");
}
else if(value2==0)
{
digitalWrite(Relay2,HIGH);
Serial.println("Relay 2 OFF");
}
value3=Firebase.getString("S3").toInt(); //Reading the Status of Variable 3 from the firebase
if(value3==1)
{
digitalWrite(Relay3,LOW);
Serial.println("Relay 3 ON");
}
else if(value3==0)
{
digitalWrite(Relay3,HIGH);
Serial.println("Relay 3 OFF");
}
value4=Firebase.getString("S4").toInt(); //Reading the Status of Variable 4 from the firebase
if(value4==1)
{
digitalWrite(Relay4,LOW);
Serial.println("Relay 4 ON");
}
else if(value4==0)
{
digitalWrite(Relay4,HIGH);
Serial.println("Relay 4 OFF");
}
}
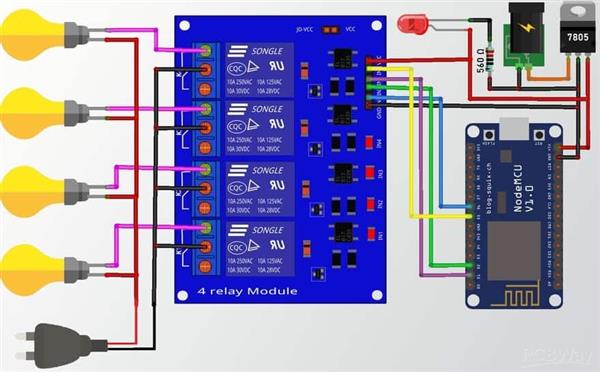
Home Automation With esp8266
- Comments(0)
- Likes(0)
- 0 USER VOTES
- YOUR VOTE 0.00 0.00
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
More by electronicguru0007
-
How to make an alarm clock with pic microcontroller he five push buttons will act as an input for setting the alarm for the required time. So one end of...
-
How to make RMS to DC Converter using IC AD736 A True-RMS or TRMS is a type of converter which converts RMS value to equivalent DC value. Here in t...
-
STM32 SPI Communcation and Data Sent SPI in STM32F103C8Comparing SPI bus in Arduino & STM32F103C8 Blue Pill board, STM32 has 2 SPI bu...
-
How to Communicate Arduinos via RS-485 What project will you develop?The project consists of 3 Arduino's. We have an Arduino UNO, a Nano, a...
-
PIC16F877A Temperature and Humidity Measurement Board Temperature and Humidity measurement is often useful in many applications like Home Automation, Envi...
-
Diy Buck Converter n the field of DC-DC Converters, A single-ended primary-inductor converter or SEPIC converter is a t...
-
Iot AC Current Measuring System Smart power monitoring is getting increasingly popular to improve energy efficiency in medium/small ...
-
ESP32 Weather Station In this project, we will learn how to create a weather station, which will display reading from a BM...
-
NRF Data Transfer Via 2 Boards There are various wireless communication technologies used in building IoT applications and RF (Radi...
-
Iot patient monitoring system When we are talking about major vital signs of a human body, there are four major parameters that we...
-
Setting up zigbee communication with nodemcu and arduino Zigbee is a popular wireless communication protocol used to transfer a small amount of data with ver...
-
Ac Dimmer Remote PCB The brightness can be controlled using the IR remote of TV, DVD, etc. Dimming Control system using M...
-
Esp32 Home Automation There are relay modules whose electromagnet can be powered by 5V and with 3.3V. Both can be used wit...
-
Lora Communication With Network This was a very simple project and can come in handy for various applications. But what it can't do ...
-
GPS Module Based Tracking Device Pcb ESP32 GPS vehicle tracker using NEO 6M GPS module and Arduino IDE. With the help of this GPS tracker...
-
Traffic Management for Emergency Vehicles using AT89S52 Microcontroller These days’ traffic congestion is the biggest problem of densely populated cities. The project focus...
-
Diy Multimeter Pcb This is a project based on Arduino board which can measureresistance, diode, continuity[H1] , voltag...
-
Live Instagram Followers Pcb ESP8266 is capable of functioning reliably in industrial environments, with an operating temperature...
-
-
-
kmMiniSchield MIDI I/O - IN/OUT/THROUGH MIDI extension for kmMidiMini
124 0 0 -
DIY Laser Power Meter with Arduino
173 0 2 -
-
-
Box & Bolt, 3D Printed Cardboard Crafting Tools
163 0 2 -